mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
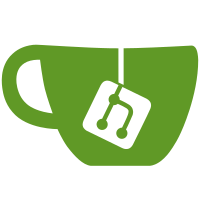
This moves the direct use of GL in cogl-framebuffer.c for handling cogl_framebuffer_read_pixels_into_bitmap() into driver/gl/cogl-framebuffer-gl.c and adds a ->framebuffer_read_pixels_into_bitmap vfunc to CoglDriverVtable. Reviewed-by: Neil Roberts <neil@linux.intel.com> (cherry picked from commit 2f893054d6754e6bc7983f061b27c7858f1a593c)
122 lines
3.6 KiB
C
122 lines
3.6 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009,2012 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see
|
|
* <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "cogl-framebuffer-nop-private.h"
|
|
|
|
#include <glib.h>
|
|
|
|
|
|
void
|
|
_cogl_framebuffer_nop_flush_state (CoglFramebuffer *draw_buffer,
|
|
CoglFramebuffer *read_buffer,
|
|
CoglFramebufferState state)
|
|
{
|
|
}
|
|
|
|
CoglBool
|
|
_cogl_offscreen_nop_allocate (CoglOffscreen *offscreen,
|
|
CoglError **error)
|
|
{
|
|
return TRUE;
|
|
}
|
|
|
|
void
|
|
_cogl_offscreen_nop_free (CoglOffscreen *offscreen)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_clear (CoglFramebuffer *framebuffer,
|
|
unsigned long buffers,
|
|
float red,
|
|
float green,
|
|
float blue,
|
|
float alpha)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_query_bits (CoglFramebuffer *framebuffer,
|
|
int *red,
|
|
int *green,
|
|
int *blue,
|
|
int *alpha)
|
|
{
|
|
*red = 0;
|
|
*green = 0;
|
|
*blue = 0;
|
|
*alpha = 0;
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_finish (CoglFramebuffer *framebuffer)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_discard_buffers (CoglFramebuffer *framebuffer,
|
|
unsigned long buffers)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_draw_attributes (CoglFramebuffer *framebuffer,
|
|
CoglPipeline *pipeline,
|
|
CoglVerticesMode mode,
|
|
int first_vertex,
|
|
int n_vertices,
|
|
CoglAttribute **attributes,
|
|
int n_attributes,
|
|
CoglDrawFlags flags)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_framebuffer_nop_draw_indexed_attributes (CoglFramebuffer *framebuffer,
|
|
CoglPipeline *pipeline,
|
|
CoglVerticesMode mode,
|
|
int first_vertex,
|
|
int n_vertices,
|
|
CoglIndices *indices,
|
|
CoglAttribute **attributes,
|
|
int n_attributes,
|
|
CoglDrawFlags flags)
|
|
{
|
|
}
|
|
|
|
CoglBool
|
|
_cogl_framebuffer_nop_read_pixels_into_bitmap (CoglFramebuffer *framebuffer,
|
|
int x,
|
|
int y,
|
|
CoglReadPixelsFlags source,
|
|
CoglBitmap *bitmap,
|
|
CoglError **error)
|
|
{
|
|
return TRUE;
|
|
}
|