mirror of
https://github.com/brl/mutter.git
synced 2024-09-19 22:15:54 -04:00
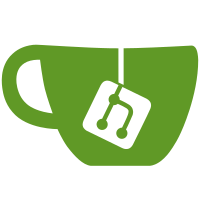
Big rework of the actor management semantics: now ClutterActor objects behave like GtkObjects - that is they have an initial "floating" reference that gets "sunk" when they are added to a ClutterGroup. This makes a group responsible of de-allocating each actor inside it, so you just have to destroy the group to get every child actor destroyed. Also, now you can do: clutter_group_add (group, clutter_video_texture_new ()); without having to care about reference counting and explicit unreffing. * clutter/clutter-private.h: Add private flags setter and getter macros. * clutter/clutter-actor.h: * clutter/clutter-actor.c: Clean up; inherit from GInitiallyUnowned; add a "visible" property; add the "destroy", "show" and "hide" signals to ClutterActorClass. (clutter_actor_show), (clutter_actor_hide): Refactor a bit; emit the "show" and "hide" signals. (clutter_actor_set_property), (clutter_actor_get_property), (clutter_actor_class_init): Implement the "visible" property; add signals. (clutter_actor_finalize): Do not leak the actor's name, if it is set. (clutter_actor_dispose): Emit the "destroy" signal here. (clutter_actor_init): Sink the initial floating flag if needed. (clutter_actor_destroy): Add a function to explicitely destroy a ClutterActor. (clutter_actor_set_parent), (clutter_actor_get_parent), (clutter_actor_unparent): Make set_parent require a valid parent; add unparent; check on get_parent; ref_sink the actor when setting its parent and unref it when unsetting it. Probably we'll need a function that does reparenting as unparent+set_parent in a single shot. * clutter/clutter-group.h: * clutter/clutter-group.c (clutter_group_dispose), (clutter_group_finalize), (clutter_group_add), (clutter_group_remove): Make the group destroy its children when disposing it; clean up, and use the newly-available clutter_actor_unparent(). * clutter/clutter-stage.h: * clutter/clutter-stage.c (clutter_stage_init): ClutterStage is a top-level actor; clean up. * clutter/clutter-video-texture.h: * clutter/clutter-video-texture.c: Clean up. * examples/super-oh.c: * examples/test.c: * examples/video-player.c: * examples/test-text.c: * examples/video-cube.c: Remove the g_object_unref() call, as the ClutterStage object is destroyed on clutter_main_quit().
130 lines
4.0 KiB
C
130 lines
4.0 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2006 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef _HAVE_CLUTTER_STAGE_H
|
|
#define _HAVE_CLUTTER_STAGE_H
|
|
|
|
#include <glib-object.h>
|
|
|
|
#include <clutter/clutter-group.h>
|
|
#include <clutter/clutter-color.h>
|
|
#include <clutter/clutter-event.h>
|
|
|
|
#include <gdk-pixbuf/gdk-pixbuf.h>
|
|
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xatom.h>
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_STAGE (clutter_stage_get_type())
|
|
|
|
#define CLUTTER_STAGE_WIDTH() \
|
|
clutter_actor_get_width(CLUTTER_ACTOR(clutter_stage_get_default()))
|
|
|
|
#define CLUTTER_STAGE_HEIGHT() \
|
|
clutter_actor_get_height(CLUTTER_ACTOR(clutter_stage_get_default()))
|
|
|
|
|
|
#define CLUTTER_STAGE(obj) \
|
|
(G_TYPE_CHECK_INSTANCE_CAST ((obj), \
|
|
CLUTTER_TYPE_STAGE, ClutterStage))
|
|
|
|
#define CLUTTER_STAGE_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_CAST ((klass), \
|
|
CLUTTER_TYPE_STAGE, ClutterStageClass))
|
|
|
|
#define CLUTTER_IS_STAGE(obj) \
|
|
(G_TYPE_CHECK_INSTANCE_TYPE ((obj), \
|
|
CLUTTER_TYPE_STAGE))
|
|
|
|
#define CLUTTER_IS_STAGE_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_TYPE ((klass), \
|
|
CLUTTER_TYPE_STAGE))
|
|
|
|
#define CLUTTER_STAGE_GET_CLASS(obj) \
|
|
(G_TYPE_INSTANCE_GET_CLASS ((obj), \
|
|
CLUTTER_TYPE_STAGE, ClutterStageClass))
|
|
|
|
typedef struct _ClutterStagePrivate ClutterStagePrivate;
|
|
typedef struct _ClutterStage ClutterStage;
|
|
typedef struct _ClutterStageClass ClutterStageClass;
|
|
|
|
struct _ClutterStage
|
|
{
|
|
ClutterGroup parent;
|
|
|
|
/*< private >*/
|
|
ClutterStagePrivate *priv;
|
|
};
|
|
|
|
struct _ClutterStageClass
|
|
{
|
|
ClutterGroupClass parent_class;
|
|
|
|
void (*input_event) (ClutterStage *stage,
|
|
ClutterEvent *event);
|
|
void (*button_press_event) (ClutterStage *stage,
|
|
ClutterButtonEvent *event);
|
|
void (*button_release_event) (ClutterStage *stage,
|
|
ClutterButtonEvent *event);
|
|
void (*key_press_event) (ClutterStage *stage,
|
|
ClutterKeyEvent *event);
|
|
void (*key_release_event) (ClutterStage *stage,
|
|
ClutterKeyEvent *event);
|
|
void (*motion_event) (ClutterStage *stage,
|
|
ClutterMotionEvent *event);
|
|
|
|
/* padding for future expansion */
|
|
void (*_clutter_stage1) (void);
|
|
void (*_clutter_stage2) (void);
|
|
void (*_clutter_stage3) (void);
|
|
void (*_clutter_stage4) (void);
|
|
void (*_clutter_stage5) (void);
|
|
void (*_clutter_stage6) (void);
|
|
};
|
|
|
|
GType clutter_stage_get_type (void) G_GNUC_CONST;
|
|
ClutterActor *clutter_stage_get_default (void);
|
|
Window clutter_stage_get_xwindow (ClutterStage *stage);
|
|
gboolean clutter_stage_set_xwindow_foreign (ClutterStage *stage,
|
|
Window xid);
|
|
void clutter_stage_set_color (ClutterStage *stage,
|
|
const ClutterColor *color);
|
|
void clutter_stage_get_color (ClutterStage *stage,
|
|
ClutterColor *color);
|
|
ClutterActor *clutter_stage_get_actor_at_pos (ClutterStage *stage,
|
|
gint x,
|
|
gint y);
|
|
GdkPixbuf * clutter_stage_snapshot (ClutterStage *stage,
|
|
gint x,
|
|
gint y,
|
|
gint width,
|
|
gint height);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif
|