mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
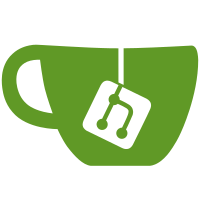
This adds a CoglAtlas type which is a data structure that keeps track of unused sub rectangles of a larger rectangle. There is a new atlased texture backend which uses this to put multiple textures into a single larger texture. Currently the atlas is always sized 256x256 and the textures are never moved once they are put in. Eventually it needs to be able to reorganise the atlas and grow it if necessary. It also needs to migrate the textures out of the atlas if mipmaps are required.
77 lines
2.1 KiB
C
77 lines
2.1 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_ATLAS_H
|
|
#define __COGL_ATLAS_H
|
|
|
|
#include <glib.h>
|
|
|
|
typedef struct _CoglAtlas CoglAtlas;
|
|
typedef struct _CoglAtlasRectangle CoglAtlasRectangle;
|
|
|
|
typedef void (* CoglAtlasCallback) (const CoglAtlasRectangle *rectangle,
|
|
gpointer rectangle_data,
|
|
gpointer user_data);
|
|
|
|
struct _CoglAtlasRectangle
|
|
{
|
|
guint x, y;
|
|
guint width, height;
|
|
};
|
|
|
|
CoglAtlas *
|
|
cogl_atlas_new (guint width, guint height,
|
|
GDestroyNotify value_destroy_func);
|
|
|
|
gboolean
|
|
cogl_atlas_add_rectangle (CoglAtlas *atlas,
|
|
guint width, guint height,
|
|
gpointer data,
|
|
CoglAtlasRectangle *rectangle);
|
|
|
|
void
|
|
cogl_atlas_remove_rectangle (CoglAtlas *atlas,
|
|
const CoglAtlasRectangle *rectangle);
|
|
|
|
guint
|
|
cogl_atlas_get_width (CoglAtlas *atlas);
|
|
|
|
guint
|
|
cogl_atlas_get_height (CoglAtlas *atlas);
|
|
|
|
guint
|
|
cogl_atlas_get_remaining_space (CoglAtlas *atlas);
|
|
|
|
guint
|
|
cogl_atlas_get_n_rectangles (CoglAtlas *atlas);
|
|
|
|
void
|
|
cogl_atlas_foreach (CoglAtlas *atlas,
|
|
CoglAtlasCallback callback,
|
|
gpointer data);
|
|
|
|
void
|
|
cogl_atlas_free (CoglAtlas *atlas);
|
|
|
|
#endif /* __COGL_ATLAS_H */
|