mirror of
https://github.com/brl/mutter.git
synced 2025-08-04 07:34:53 +00:00
cogl-color: Provide setters for all the channels
We only had getters for the red, green, blue and alpha channels of a color. This meant that, if you wanted to change, say, the alpha component of a color, one would need to query the red, green and blue channels and use set_from_4ub() or set_from_4f(). Instead of this, just provide some setters for CoglColor, using the same naming scheme than the existing getters.
This commit is contained in:
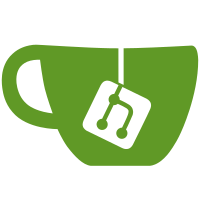
committed by
Robert Bragg
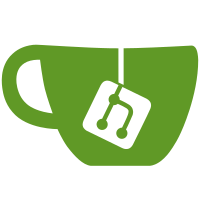
parent
896dd975d1
commit
98f2d2516b
@@ -155,6 +155,90 @@ cogl_color_get_alpha (const CoglColor *color)
|
||||
return ((float) color->alpha / 255.0);
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_red_byte (CoglColor *color,
|
||||
unsigned char red)
|
||||
{
|
||||
color->red = red;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_red_float (CoglColor *color,
|
||||
float red)
|
||||
{
|
||||
color->red = red * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_red (CoglColor *color,
|
||||
float red)
|
||||
{
|
||||
color->red = red * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_green_byte (CoglColor *color,
|
||||
unsigned char green)
|
||||
{
|
||||
color->green = green;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_green_float (CoglColor *color,
|
||||
float green)
|
||||
{
|
||||
color->green = green * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_green (CoglColor *color,
|
||||
float green)
|
||||
{
|
||||
color->green = green * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_blue_byte (CoglColor *color,
|
||||
unsigned char blue)
|
||||
{
|
||||
color->blue = blue;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_blue_float (CoglColor *color,
|
||||
float blue)
|
||||
{
|
||||
color->blue = blue * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_blue (CoglColor *color,
|
||||
float blue)
|
||||
{
|
||||
color->blue = blue * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_alpha_byte (CoglColor *color,
|
||||
unsigned char alpha)
|
||||
{
|
||||
color->alpha = alpha;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_alpha_float (CoglColor *color,
|
||||
float alpha)
|
||||
{
|
||||
color->alpha = alpha * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_set_alpha (CoglColor *color,
|
||||
float alpha)
|
||||
{
|
||||
color->alpha = alpha * 255.0;
|
||||
}
|
||||
|
||||
void
|
||||
cogl_color_premultiply (CoglColor *color)
|
||||
{
|
||||
|
@@ -286,6 +286,162 @@ cogl_color_get_blue (const CoglColor *color);
|
||||
float
|
||||
cogl_color_get_alpha (const CoglColor *color);
|
||||
|
||||
/**
|
||||
* cogl_color_set_red_byte:
|
||||
* @color: a #CoglColor
|
||||
* @red: a byte value between 0 and 255
|
||||
*
|
||||
* Sets the red channel of @color to @red.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_red_byte (CoglColor *color,
|
||||
unsigned char red);
|
||||
|
||||
/**
|
||||
* cogl_color_set_green_byte:
|
||||
* @color: a #CoglColor
|
||||
* @green: a byte value between 0 and 255
|
||||
*
|
||||
* Sets the green channel of @color to @green.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_green_byte (CoglColor *color,
|
||||
unsigned char green);
|
||||
|
||||
/**
|
||||
* cogl_color_set_blue_byte:
|
||||
* @color: a #CoglColor
|
||||
* @blue: a byte value between 0 and 255
|
||||
*
|
||||
* Sets the blue channel of @color to @blue.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_blue_byte (CoglColor *color,
|
||||
unsigned char blue);
|
||||
|
||||
/**
|
||||
* cogl_color_set_alpha_byte:
|
||||
* @color: a #CoglColor
|
||||
* @alpha: a byte value between 0 and 255
|
||||
*
|
||||
* Sets the alpha channel of @color to @alpha.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_alpha_byte (CoglColor *color,
|
||||
unsigned char alpha);
|
||||
|
||||
/**
|
||||
* cogl_color_set_red_float:
|
||||
* @color: a #CoglColor
|
||||
* @red: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the red channel of @color to @red.
|
||||
*
|
||||
* since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_red_float (CoglColor *color,
|
||||
float red);
|
||||
|
||||
/**
|
||||
* cogl_color_set_green_float:
|
||||
* @color: a #CoglColor
|
||||
* @green: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the green channel of @color to @green.
|
||||
*
|
||||
* since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_green_float (CoglColor *color,
|
||||
float green);
|
||||
|
||||
/**
|
||||
* cogl_color_set_blue_float:
|
||||
* @color: a #CoglColor
|
||||
* @blue: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the blue channel of @color to @blue.
|
||||
*
|
||||
* since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_blue_float (CoglColor *color,
|
||||
float blue);
|
||||
|
||||
/**
|
||||
* cogl_color_set_alpha_float:
|
||||
* @color: a #CoglColor
|
||||
* @alpha: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the alpha channel of @color to @alpha.
|
||||
*
|
||||
* since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_alpha_float (CoglColor *color,
|
||||
float alpha);
|
||||
|
||||
/**
|
||||
* cogl_color_set_red:
|
||||
* @color: a #CoglColor
|
||||
* @red: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the red channel of @color to @red.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_red (CoglColor *color,
|
||||
float red);
|
||||
|
||||
/**
|
||||
* cogl_color_set_green:
|
||||
* @color: a #CoglColor
|
||||
* @green: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the green channel of @color to @green.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_green (CoglColor *color,
|
||||
float green);
|
||||
|
||||
/**
|
||||
* cogl_color_set_blue:
|
||||
* @color: a #CoglColor
|
||||
* @blue: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the blue channel of @color to @blue.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_blue (CoglColor *color,
|
||||
float blue);
|
||||
|
||||
/**
|
||||
* cogl_color_set_alpha:
|
||||
* @color: a #CoglColor
|
||||
* @alpha: a float value between 0.0f and 1.0f
|
||||
*
|
||||
* Sets the alpha channel of @color to @alpha.
|
||||
*
|
||||
* Since: 1.4
|
||||
*/
|
||||
void
|
||||
cogl_color_set_alpha (CoglColor *color,
|
||||
float alpha);
|
||||
|
||||
/**
|
||||
* cogl_color_premultiply:
|
||||
* @color: the color to premultiply
|
||||
|
@@ -382,6 +382,24 @@ cogl_color_get_green_float
|
||||
cogl_color_get_blue_float
|
||||
cogl_color_get_alpha_float
|
||||
|
||||
<SUBSECTION>
|
||||
cogl_color_set_red
|
||||
cogl_color_set_green
|
||||
cogl_color_set_blue
|
||||
cogl_color_set_alpha
|
||||
|
||||
<SUBSECTION>
|
||||
cogl_color_set_red_byte
|
||||
cogl_color_set_green_byte
|
||||
cogl_color_set_blue_byte
|
||||
cogl_color_set_alpha_byte
|
||||
|
||||
<SUBSECTION>
|
||||
cogl_color_set_red_float
|
||||
cogl_color_set_green_float
|
||||
cogl_color_set_blue_float
|
||||
cogl_color_set_alpha_float
|
||||
|
||||
<SUBSECTION>
|
||||
cogl_color_premultiply
|
||||
cogl_color_unpremultiply
|
||||
|
Reference in New Issue
Block a user