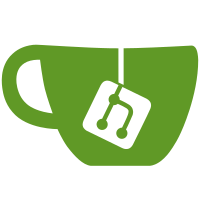
The CoglBitmap struct is now only defined within cogl-bitmap.c so that all of its members can now only be accessed with accessor functions. To get to the data pointer for the bitmap image you must first call _cogl_bitmap_map and later call _cogl_bitmap_unmap. The map function takes the same arguments as cogl_pixel_array_map so that eventually we can make a bitmap optionally internally divert to a pixel array. There is a _cogl_bitmap_new_from_data function which constructs a new bitmap object and takes ownership of the data pointer. The function gets passed a destroy callback which gets called when the bitmap is freed. This is similar to how gdk_pixbuf_new_from_data works. Alternatively NULL can be passed for the destroy function which means that the caller will manage the life of the pointer (but must guarantee that it stays alive at least until the bitmap is freed). This mechanism is used instead of the old approach of creating a CoglBitmap struct on the stack and manually filling in the members. It could also later be used to create a CoglBitmap that owns a GdkPixbuf ref so that we don't necessarily have to copy the GdkPixbuf data when converting to a bitmap. There is also _cogl_bitmap_new_shared. This creates a bitmap using a reference to another CoglBitmap for the data. This is a bit of a hack but it is needed by the atlas texture backend which wants to divert the set_region virtual to another texture but it needs to override the format of the bitmap to ignore the premult flag.
65 lines
2.0 KiB
C
65 lines
2.0 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_ATLAS_TEXTURE_H
|
|
#define __COGL_ATLAS_TEXTURE_H
|
|
|
|
#include "cogl-handle.h"
|
|
#include "cogl-texture-private.h"
|
|
#include "cogl-atlas.h"
|
|
|
|
#define COGL_ATLAS_TEXTURE(tex) ((CoglAtlasTexture *) tex)
|
|
|
|
typedef struct _CoglAtlasTexture CoglAtlasTexture;
|
|
|
|
struct _CoglAtlasTexture
|
|
{
|
|
CoglTexture _parent;
|
|
|
|
/* The format that the texture is in. This isn't necessarily the
|
|
same format as the atlas texture because we can store
|
|
pre-multiplied and non-pre-multiplied textures together */
|
|
CoglPixelFormat format;
|
|
|
|
/* The rectangle that was used to add this texture to the
|
|
atlas. This includes the 1-pixel border */
|
|
CoglAtlasRectangle rectangle;
|
|
|
|
/* The texture might need to be migrated out in which case this will
|
|
be set to TRUE and sub_texture will actually be a real texture */
|
|
gboolean in_atlas;
|
|
|
|
/* A CoglSubTexture representing the region for easy rendering */
|
|
CoglHandle sub_texture;
|
|
};
|
|
|
|
GQuark
|
|
_cogl_handle_atlas_texture_get_type (void);
|
|
|
|
CoglHandle
|
|
_cogl_atlas_texture_new_from_bitmap (CoglBitmap *bmp,
|
|
CoglTextureFlags flags,
|
|
CoglPixelFormat internal_format);
|
|
|
|
#endif /* __COGL_ATLAS_TEXTURE_H */
|