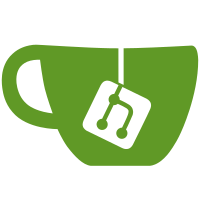
The user widget is the username and avatar shown on the unlock dialog. The login dialog has something very similar. This commit separates the user widget out to its own file, so we can use it from the login dialog in a later commit. https://bugzilla.gnome.org/show_bug.cgi?id=694062
62 lines
1.8 KiB
JavaScript
62 lines
1.8 KiB
JavaScript
|
|
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
//
|
|
// A widget showing the user avatar and name
|
|
const AccountsService = imports.gi.AccountsService;
|
|
const Lang = imports.lang;
|
|
const St = imports.gi.St;
|
|
|
|
const UserMenu = imports.ui.userMenu;
|
|
|
|
const UserWidget = new Lang.Class({
|
|
Name: 'UserWidget',
|
|
|
|
_init: function(user) {
|
|
this._user = user;
|
|
|
|
this.actor = new St.BoxLayout({ style_class: 'user-widget',
|
|
vertical: false });
|
|
|
|
this._avatar = new UserMenu.UserAvatarWidget(user);
|
|
this.actor.add(this._avatar.actor,
|
|
{ x_fill: true, y_fill: true });
|
|
|
|
this._label = new St.Label({ style_class: 'user-widget-label' });
|
|
this.actor.add(this._label,
|
|
{ expand: true,
|
|
x_fill: true,
|
|
y_fill: false,
|
|
y_align: St.Align.MIDDLE });
|
|
|
|
this._userLoadedId = this._user.connect('notify::is-loaded',
|
|
Lang.bind(this, this._updateUser));
|
|
this._userChangedId = this._user.connect('changed',
|
|
Lang.bind(this, this._updateUser));
|
|
if (this._user.is_loaded)
|
|
this._updateUser();
|
|
},
|
|
|
|
destroy: function() {
|
|
if (this._userLoadedId != 0) {
|
|
this._user.disconnect(this._userLoadedId);
|
|
this._userLoadedId = 0;
|
|
}
|
|
|
|
if (this._userChangedId != 0) {
|
|
this._user.disconnect(this._userChangedId);
|
|
this._userChangedId = 0;
|
|
}
|
|
|
|
this.actor.destroy();
|
|
},
|
|
|
|
_updateUser: function() {
|
|
if (this._user.is_loaded)
|
|
this._label.text = this._user.get_real_name();
|
|
else
|
|
this._label.text = '';
|
|
|
|
this._avatar.update();
|
|
}
|
|
});
|