shell/util: Add touch_file_async() helper
Add a small helper method to asynchronously "touch" a file and return whether the file was created or not. As g_file_make_directory_with_parents() doesn't have an async variant, we need a C helper to make the entire operation non-blocking. https://gitlab.gnome.org/GNOME/gnome-shell/issues/2432
This commit is contained in:
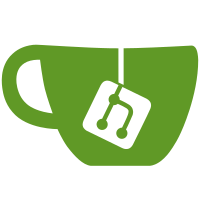
committed by
Florian Müllner
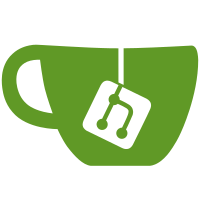
parent
e6a814fac8
commit
f52574bd28
@ -291,6 +291,68 @@ shell_get_file_contents_utf8_sync (const char *path,
|
||||
return contents;
|
||||
}
|
||||
|
||||
static void
|
||||
touch_file (GTask *task,
|
||||
gpointer object,
|
||||
gpointer task_data,
|
||||
GCancellable *cancellable)
|
||||
{
|
||||
GFile *file = object;
|
||||
g_autoptr (GFile) parent = NULL;
|
||||
g_autoptr (GFileOutputStream) stream = NULL;
|
||||
GError *error = NULL;
|
||||
|
||||
parent = g_file_get_parent (file);
|
||||
g_file_make_directory_with_parents (parent, cancellable, &error);
|
||||
|
||||
if (error && !g_error_matches (error, G_IO_ERROR, G_IO_ERROR_EXISTS))
|
||||
{
|
||||
g_task_return_error (task, error);
|
||||
return;
|
||||
}
|
||||
g_clear_error (&error);
|
||||
|
||||
stream = g_file_create (file, G_FILE_CREATE_NONE, cancellable, &error);
|
||||
|
||||
if (error && !g_error_matches (error, G_IO_ERROR, G_IO_ERROR_EXISTS))
|
||||
{
|
||||
g_task_return_error (task, error);
|
||||
return;
|
||||
}
|
||||
g_clear_error (&error);
|
||||
|
||||
if (stream)
|
||||
g_output_stream_close (G_OUTPUT_STREAM (stream), NULL, NULL);
|
||||
|
||||
g_task_return_boolean (task, stream != NULL);
|
||||
}
|
||||
|
||||
void
|
||||
shell_util_touch_file_async (GFile *file,
|
||||
GAsyncReadyCallback callback,
|
||||
gpointer user_data)
|
||||
{
|
||||
g_autoptr (GTask) task = NULL;
|
||||
|
||||
g_return_if_fail (G_IS_FILE (file));
|
||||
|
||||
task = g_task_new (file, NULL, callback, user_data);
|
||||
g_task_set_source_tag (task, shell_util_touch_file_async);
|
||||
|
||||
g_task_run_in_thread (task, touch_file);
|
||||
}
|
||||
|
||||
gboolean
|
||||
shell_util_touch_file_finish (GFile *file,
|
||||
GAsyncResult *res,
|
||||
GError **error)
|
||||
{
|
||||
g_return_val_if_fail (G_IS_FILE (file), FALSE);
|
||||
g_return_val_if_fail (G_IS_TASK (res), FALSE);
|
||||
|
||||
return g_task_propagate_boolean (G_TASK (res), error);
|
||||
}
|
||||
|
||||
/**
|
||||
* shell_util_wifexited:
|
||||
* @status: the status returned by wait() or waitpid()
|
||||
|
@ -30,6 +30,13 @@ gboolean shell_write_string_to_stream (GOutputStream *stream,
|
||||
char *shell_get_file_contents_utf8_sync (const char *path,
|
||||
GError **error);
|
||||
|
||||
void shell_util_touch_file_async (GFile *file,
|
||||
GAsyncReadyCallback callback,
|
||||
gpointer user_data);
|
||||
gboolean shell_util_touch_file_finish (GFile *file,
|
||||
GAsyncResult *res,
|
||||
GError **error);
|
||||
|
||||
gboolean shell_util_wifexited (int status,
|
||||
int *exit);
|
||||
|
||||
|
Reference in New Issue
Block a user