appDisplay: Make _items object a Map
Use a Map instead of an Object here makes it easier to loop through keys, which we're going to do in the next commit. https://gitlab.gnome.org/GNOME/gnome-shell/merge_requests/799
This commit is contained in:
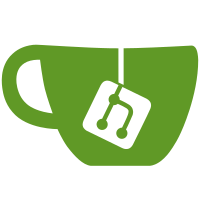
committed by
Florian Müllner
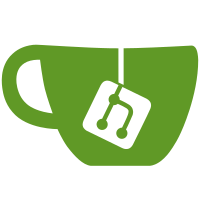
parent
c4fa052b03
commit
61b71998a0
@@ -165,7 +165,7 @@ var BaseAppView = GObject.registerClass({
|
|||||||
// Standard hack for ClutterBinLayout
|
// Standard hack for ClutterBinLayout
|
||||||
this._grid.x_expand = true;
|
this._grid.x_expand = true;
|
||||||
|
|
||||||
this._items = {};
|
this._items = new Map();
|
||||||
this._allItems = [];
|
this._allItems = [];
|
||||||
}
|
}
|
||||||
|
|
||||||
@@ -190,7 +190,7 @@ var BaseAppView = GObject.registerClass({
|
|||||||
|
|
||||||
this._allItems.splice(iconIndex, 1);
|
this._allItems.splice(iconIndex, 1);
|
||||||
icon.destroy();
|
icon.destroy();
|
||||||
delete this._items[id];
|
this._items.delete(id);
|
||||||
});
|
});
|
||||||
|
|
||||||
// Add new app icons
|
// Add new app icons
|
||||||
@@ -199,7 +199,7 @@ var BaseAppView = GObject.registerClass({
|
|||||||
|
|
||||||
this._allItems.splice(iconIndex, 0, icon);
|
this._allItems.splice(iconIndex, 0, icon);
|
||||||
this._grid.addItem(icon, iconIndex);
|
this._grid.addItem(icon, iconIndex);
|
||||||
this._items[icon.id] = icon;
|
this._items.set(icon.id, icon);
|
||||||
});
|
});
|
||||||
|
|
||||||
this.emit('view-loaded');
|
this.emit('view-loaded');
|
||||||
@@ -214,24 +214,27 @@ var BaseAppView = GObject.registerClass({
|
|||||||
}
|
}
|
||||||
|
|
||||||
_selectAppInternal(id) {
|
_selectAppInternal(id) {
|
||||||
if (this._items[id])
|
if (this._items.has(id))
|
||||||
this._items[id].navigate_focus(null, St.DirectionType.TAB_FORWARD, false);
|
this._items.get(id).navigate_focus(null, St.DirectionType.TAB_FORWARD, false);
|
||||||
else
|
else
|
||||||
log(`No such application ${id}`);
|
log(`No such application ${id}`);
|
||||||
}
|
}
|
||||||
|
|
||||||
selectApp(id) {
|
selectApp(id) {
|
||||||
if (this._items[id] && this._items[id].mapped) {
|
if (this._items.has(id)) {
|
||||||
|
let item = this._items.get(id);
|
||||||
|
|
||||||
|
if (item.mapped) {
|
||||||
this._selectAppInternal(id);
|
this._selectAppInternal(id);
|
||||||
} else if (this._items[id]) {
|
} else {
|
||||||
// Need to wait until the view is mapped
|
// Need to wait until the view is mapped
|
||||||
let signalId = this._items[id].connect('notify::mapped',
|
let signalId = item.connect('notify::mapped', actor => {
|
||||||
actor => {
|
|
||||||
if (actor.mapped) {
|
if (actor.mapped) {
|
||||||
actor.disconnect(signalId);
|
actor.disconnect(signalId);
|
||||||
this._selectAppInternal(id);
|
this._selectAppInternal(id);
|
||||||
}
|
}
|
||||||
});
|
});
|
||||||
|
}
|
||||||
} else {
|
} else {
|
||||||
// Need to wait until the view is built
|
// Need to wait until the view is built
|
||||||
let signalId = this.connect('view-loaded', () => {
|
let signalId = this.connect('view-loaded', () => {
|
||||||
@@ -448,7 +451,7 @@ var AllView = GObject.registerClass({
|
|||||||
this.folderIcons.forEach(folder => {
|
this.folderIcons.forEach(folder => {
|
||||||
let folderApps = folder.getAppIds();
|
let folderApps = folder.getAppIds();
|
||||||
folderApps.forEach(appId => {
|
folderApps.forEach(appId => {
|
||||||
let appIcon = this._items[appId];
|
let appIcon = this._items.get(appId);
|
||||||
appIcon.visible = false;
|
appIcon.visible = false;
|
||||||
});
|
});
|
||||||
});
|
});
|
||||||
@@ -484,7 +487,7 @@ var AllView = GObject.registerClass({
|
|||||||
let folders = this._folderSettings.get_strv('folder-children');
|
let folders = this._folderSettings.get_strv('folder-children');
|
||||||
folders.forEach(id => {
|
folders.forEach(id => {
|
||||||
let path = `${this._folderSettings.path}folders/${id}/`;
|
let path = `${this._folderSettings.path}folders/${id}/`;
|
||||||
let icon = this._items[id];
|
let icon = this._items.get(id);
|
||||||
if (!icon) {
|
if (!icon) {
|
||||||
icon = new FolderIcon(id, path, this);
|
icon = new FolderIcon(id, path, this);
|
||||||
icon.connect('name-changed', this._itemNameChanged.bind(this));
|
icon.connect('name-changed', this._itemNameChanged.bind(this));
|
||||||
@@ -503,7 +506,7 @@ var AllView = GObject.registerClass({
|
|||||||
let favoritesWritable = global.settings.is_writable('favorite-apps');
|
let favoritesWritable = global.settings.is_writable('favorite-apps');
|
||||||
|
|
||||||
apps.forEach(appId => {
|
apps.forEach(appId => {
|
||||||
let icon = this._items[appId];
|
let icon = this._items.get(appId);
|
||||||
if (!icon) {
|
if (!icon) {
|
||||||
let app = appSys.lookup_app(appId);
|
let app = appSys.lookup_app(appId);
|
||||||
|
|
||||||
@@ -721,13 +724,14 @@ var AllView = GObject.registerClass({
|
|||||||
}
|
}
|
||||||
|
|
||||||
_updateIconOpacities(folderOpen) {
|
_updateIconOpacities(folderOpen) {
|
||||||
for (let id in this._items) {
|
for (let icon of this._items.values()) {
|
||||||
let opacity;
|
let opacity;
|
||||||
if (folderOpen && !this._items[id].checked)
|
if (folderOpen && !icon.checked)
|
||||||
opacity = INACTIVE_GRID_OPACITY;
|
opacity = INACTIVE_GRID_OPACITY;
|
||||||
else
|
else
|
||||||
opacity = 255;
|
opacity = 255;
|
||||||
this._items[id].ease({
|
|
||||||
|
icon.ease({
|
||||||
opacity,
|
opacity,
|
||||||
duration: INACTIVE_GRID_OPACITY_ANIMATION_TIME,
|
duration: INACTIVE_GRID_OPACITY_ANIMATION_TIME,
|
||||||
mode: Clutter.AnimationMode.EASE_OUT_QUAD,
|
mode: Clutter.AnimationMode.EASE_OUT_QUAD,
|
||||||
@@ -911,7 +915,7 @@ var AllView = GObject.registerClass({
|
|||||||
return false;
|
return false;
|
||||||
}
|
}
|
||||||
|
|
||||||
let appItems = apps.map(id => this._items[id].app);
|
let appItems = apps.map(id => this._items.get(id).app);
|
||||||
let folderName = _findBestFolderName(appItems);
|
let folderName = _findBestFolderName(appItems);
|
||||||
if (!folderName)
|
if (!folderName)
|
||||||
folderName = _("Unnamed Folder");
|
folderName = _("Unnamed Folder");
|
||||||
@@ -986,7 +990,7 @@ class FrequentView extends BaseAppView {
|
|||||||
for (let i = 0; i < mostUsed.length; i++) {
|
for (let i = 0; i < mostUsed.length; i++) {
|
||||||
if (!mostUsed[i].get_app_info().should_show())
|
if (!mostUsed[i].get_app_info().should_show())
|
||||||
continue;
|
continue;
|
||||||
let appIcon = this._items[mostUsed[i].get_id()];
|
let appIcon = this._items.get(mostUsed[i].get_id());
|
||||||
if (!appIcon) {
|
if (!appIcon) {
|
||||||
appIcon = new AppIcon(mostUsed[i], {
|
appIcon = new AppIcon(mostUsed[i], {
|
||||||
isDraggable: favoritesWritable,
|
isDraggable: favoritesWritable,
|
||||||
@@ -1449,7 +1453,7 @@ class FolderView extends BaseAppView {
|
|||||||
if (apps.some(appIcon => appIcon.id == appId))
|
if (apps.some(appIcon => appIcon.id == appId))
|
||||||
return;
|
return;
|
||||||
|
|
||||||
let icon = this._items[appId];
|
let icon = this._items.get(appId);
|
||||||
if (!icon)
|
if (!icon)
|
||||||
icon = new AppIcon(app);
|
icon = new AppIcon(app);
|
||||||
|
|
||||||
|
Reference in New Issue
Block a user