mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
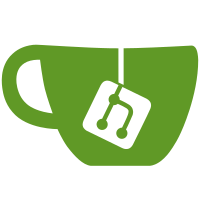
This adds cogl_onscreen_template_set_swap_throttled() api that allows developers to specify their preference for swap buffer throttling up-front as part of the onscreen template that is used to create a CoglDisplay when initializing Cogl. This is desirable because some platforms may not support configuring swap throttling on a per framebuffer basis and also since applications often want to apply the same policy to all onscreen framebuffers anyway.
97 lines
2.8 KiB
C
97 lines
2.8 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2011 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*
|
|
* Authors:
|
|
* Robert Bragg <robert@linux.intel.com>
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-object.h"
|
|
|
|
#include "cogl-framebuffer-private.h"
|
|
#include "cogl-onscreen-template-private.h"
|
|
|
|
#include <stdlib.h>
|
|
|
|
static void _cogl_onscreen_template_free (CoglOnscreenTemplate *onscreen_template);
|
|
|
|
COGL_OBJECT_DEFINE (OnscreenTemplate, onscreen_template);
|
|
|
|
GQuark
|
|
cogl_onscreen_template_error_quark (void)
|
|
{
|
|
return g_quark_from_static_string ("cogl-onscreen-template-error-quark");
|
|
}
|
|
|
|
static void
|
|
_cogl_onscreen_template_free (CoglOnscreenTemplate *onscreen_template)
|
|
{
|
|
g_slice_free (CoglOnscreenTemplate, onscreen_template);
|
|
}
|
|
|
|
CoglOnscreenTemplate *
|
|
cogl_onscreen_template_new (CoglSwapChain *swap_chain)
|
|
{
|
|
CoglOnscreenTemplate *onscreen_template = g_slice_new0 (CoglOnscreenTemplate);
|
|
char *user_config;
|
|
|
|
onscreen_template->config.swap_chain = swap_chain;
|
|
if (swap_chain)
|
|
cogl_object_ref (swap_chain);
|
|
else
|
|
onscreen_template->config.swap_chain = cogl_swap_chain_new ();
|
|
|
|
onscreen_template->config.need_stencil = TRUE;
|
|
onscreen_template->config.samples_per_pixel = 0;
|
|
|
|
user_config = getenv ("COGL_POINT_SAMPLES_PER_PIXEL");
|
|
if (user_config)
|
|
{
|
|
unsigned long samples_per_pixel = strtoul (user_config, NULL, 10);
|
|
if (samples_per_pixel != ULONG_MAX)
|
|
onscreen_template->config.samples_per_pixel =
|
|
samples_per_pixel;
|
|
}
|
|
|
|
return _cogl_onscreen_template_object_new (onscreen_template);
|
|
}
|
|
|
|
void
|
|
cogl_onscreen_template_set_samples_per_pixel (
|
|
CoglOnscreenTemplate *onscreen_template,
|
|
int samples_per_pixel)
|
|
{
|
|
onscreen_template->config.samples_per_pixel = samples_per_pixel;
|
|
}
|
|
|
|
void
|
|
cogl_onscreen_template_set_swap_throttled (
|
|
CoglOnscreenTemplate *onscreen_template,
|
|
gboolean throttled)
|
|
{
|
|
onscreen_template->config.swap_throttled = throttled;
|
|
}
|