mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
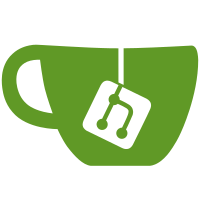
This merges the two implementations of CoglProgram for the GLES2 and GL backends into one. The implementation is more like the GLES2 version which would track the uniform values and delay sending them to GL. CoglProgram is now effectively just a GList of CoglShaders along with an array of stored uniform values. CoglProgram never actually creates a GL program, instead this is left up to the GLSL material backend. This is necessary on GLES2 where we may need to relink the user's program with different generated shaders depending on the other emulated fixed function state. It will also be necessary in the future GLSL backends for regular OpenGL. The GLSL and ARBfp material backends are now the ones that create and link the GL program from the list of shaders. The linked program is attached to the private material state so that it can be reused if the CoglProgram is used again with the same material. This does mean the program will get relinked if the shader is used with multiple materials. This will be particularly bad if the legacy cogl_program_use function is used because that effectively always makes one-shot materials. This problem will hopefully be alleviated if we make a hash table with a cache of generated programs. The cogl program would then need to become part of the hash lookup. Each CoglProgram now has an age counter which is incremented every time a shader is added. This is used by the material backends to detect when we need to create a new GL program for the user program. The internal _cogl_use_program function now takes a GL program handle rather than a CoglProgram. It no longer needs any special differences for GLES2. The GLES2 wrapper function now also uses this function to bind its generated shaders. The ARBfp shaders no longer store a copy of the program source but instead just directly create a program object when cogl_shader_source is called. This avoids having to reupload the source if the same shader is used in multiple materials. There are currently a few gross hacks to get the GLES2 backend to work with this. The problem is that the GLSL material backend is now generating a complete GL program but the GLES2 wrapper still needs to add its fixed function emulation shaders if the program doesn't provide either a vertex or fragment shader. There is a new function in the GLES2 wrapper called _cogl_gles2_use_program which replaces the previous cogl_program_use implementation. It extracts the GL shaders from the GL program object and creates a new GL program containing all of the shaders plus its fixed function emulation. This new program is returned to the GLSL material backend so that it can still flush the custom uniforms using it. The user_program is attached to the GLES2 settings struct as before but its stored using a GL program handle rather than a CoglProgram pointer. This hack will go away once the GLSL material backend replaces the GLES2 wrapper by generating the code itself. Under Mesa this currently generates some GL errors when glClear is called in test-cogl-shader-glsl. I think this is due to a bug in Mesa however. When the user program on the material is changed the GLSL backend gets notified and deletes the GL program that it linked from the user shaders. The program will still be bound in GL however. Leaving a deleted shader bound exposes a bug in Mesa's glClear implementation. More details are here: https://bugs.freedesktop.org/show_bug.cgi?id=31194
53 lines
1.3 KiB
C
53 lines
1.3 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_SHADER_H
|
|
#define __COGL_SHADER_H
|
|
|
|
#include "cogl-handle.h"
|
|
|
|
typedef struct _CoglShader CoglShader;
|
|
|
|
typedef enum
|
|
{
|
|
COGL_SHADER_LANGUAGE_GLSL,
|
|
#ifdef HAVE_COGL_GL
|
|
COGL_SHADER_LANGUAGE_ARBFP
|
|
#endif
|
|
} CoglShaderLanguage;
|
|
|
|
struct _CoglShader
|
|
{
|
|
CoglHandleObject _parent;
|
|
GLuint gl_handle;
|
|
CoglShaderType type;
|
|
CoglShaderLanguage language;
|
|
};
|
|
|
|
CoglShader *_cogl_shader_pointer_from_handle (CoglHandle handle);
|
|
|
|
CoglShaderLanguage
|
|
_cogl_program_get_language (CoglHandle handle);
|
|
|
|
#endif /* __COGL_SHADER_H */
|