mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
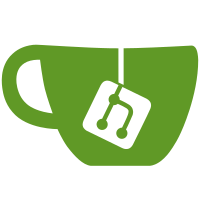
* clutter-color.h: * clutter-color.c: Reimplement ClutterColor as a boxed type; add convenience API for color handling, like: add, subtract, shade, HSL color-space conversion, packing and unpacking. * clutter-private.h: Update ClutterMainContext, and export the main context pointer here. * clutter-rectangle.h: * clutter-rectangle.c: Update the color-related code; make clutter_rectangle_new() and empty constructor and provide clutter_rectangle_new_with_color(); provide color setter and getter API. * clutter-label.h: * clutter-label.c: Rename the "font" property to "font-name"; update the color-related code to the new ClutterColor object; rename clutter_label_new() to clutter_label_new_with_text(), and add setters and getters for the properties. * clutter-marshal.list: Add VOID:OBJECT and VOID:BOXED marshallers generators. * clutter-stage.h: * clutter-stage.c: Rework the API: provide a default constructor for a singleton object, named clutter_stage_get_default(), which supercedes the clutter_stage() function in clutter-main; provide new events: button-press-event, button-release-event, key-press-event and key-release-event; update the color-related code; (clutter_stage_snapshot): Allow negative width and height when taking a snapshot (meaning: use full width/height). (clutter_stage_get_element_at_pos): Rename clutter_stage_pick(). * clutter-element.c (clutter_element_paint): Clean up the stage and color related code. * clutter-event.h: * clutter-event.c: Add generic ClutterAnyEvent type; add clutter_event_new(), clutter_event_copy() and clutter_event_free(); make ClutterEvent a boxed type. * clutter-main.h: * clutter-main.c: Remove clutter_stage(); add clutter_main_quit(), for cleanly quitting from clutter_main(); add multiple mainloops support; allocate the ClutterCntx instead of adding it to the stack; re-work the ClutterEvent dispatching. * clutter-group.c (clutter_group_add), (clutter_group_remove): Keep a reference on the element when added to a ClutterGroup. * examples/rects.py * examples/test.c: * examples/test-text.c: * examples/video-cube.c: * examples/super-oh.c: * examples/test-video.c: Update.
230 lines
5.9 KiB
Scheme
230 lines
5.9 KiB
Scheme
;; -*- scheme -*-
|
|
;;
|
|
;; Try and keep everything sorted
|
|
;;
|
|
|
|
;; Boxed types
|
|
|
|
(define-boxed Color
|
|
(in-module "Clutter")
|
|
(c-name "ClutterColor")
|
|
(gtype-id "CLUTTER_TYPE_COLOR")
|
|
(fields
|
|
'("guint8" "red")
|
|
'("guint8" "green")
|
|
'("guint8" "blue")
|
|
'("guint8" "alpha")
|
|
)
|
|
)
|
|
|
|
(define-boxed ElementBox
|
|
(in-module "Clutter")
|
|
(c-name "ClutterElementBox")
|
|
(gtype-id "CLUTTER_TYPE_ELEMENT_BOX")
|
|
(fields
|
|
'("gint" "x1")
|
|
'("gint" "y1")
|
|
'("gint" "x2")
|
|
'("gint" "y2")
|
|
)
|
|
)
|
|
|
|
(define-boxed Event
|
|
(in-module "Clutter")
|
|
(c-name "ClutterEvent")
|
|
(gtype-id "CLUTTER_TYPE_EVENT")
|
|
(fields
|
|
'("ClutterEventType" "type")
|
|
)
|
|
)
|
|
|
|
(define-boxed Geometry
|
|
(in-module "Clutter")
|
|
(c-name "ClutterGeometry")
|
|
(gtype-id "CLUTTER_TYPE_GEOMETRY")
|
|
(fields
|
|
'("gint" "x")
|
|
'("gint" "y")
|
|
'("gint" "width")
|
|
'("gint" "height")
|
|
)
|
|
)
|
|
|
|
;; Enumerations and flags ...
|
|
|
|
(define-flags ElementFlags
|
|
(in-module "Clutter")
|
|
(c-name "ClutterElementFlags")
|
|
(gtype-id "CLUTTER_TYPE_ELEMENT_FLAGS")
|
|
(values
|
|
'("mapped" "CLUTTER_ELEMENT_MAPPED")
|
|
'("realized" "CLUTTER_ELEMENT_REALIZED")
|
|
)
|
|
)
|
|
|
|
(define-flags ElementTransform
|
|
(in-module "Clutter")
|
|
(c-name "ClutterElementTransform")
|
|
(gtype-id "CLUTTER_TYPE_ELEMENT_TRANSFORM")
|
|
(values
|
|
'("x" "CLUTTER_ELEMENT_MIRROR_X")
|
|
'("y" "CLUTTER_ELEMENT_MIRROR_Y")
|
|
)
|
|
)
|
|
|
|
(define-enum EventType
|
|
(in-module "Clutter")
|
|
(c-name "ClutterEventType")
|
|
(gtype-id "CLUTTER_TYPE_EVENT_TYPE")
|
|
(values
|
|
'("nothing" "CLUTTER_NOTHING")
|
|
'("key-press" "CLUTTER_KEY_PRESS")
|
|
'("key-release" "CLUTTER_KEY_RELEASE")
|
|
'("motion" "CLUTTER_MOTION")
|
|
'("button-press" "CLUTTER_BUTTON_PRESS")
|
|
'("2button-press" "CLUTTER_2BUTTON_PRESS")
|
|
'("button-release" "CLUTTER_BUTTON_RELEASE")
|
|
)
|
|
)
|
|
|
|
(define-enum VideoTextureAspectRatio
|
|
(in-module "Clutter")
|
|
(c-name "ClutterVideoTextureAspectRatio")
|
|
(gtype-id "CLUTTER_TYPE_VIDEO_TEXTURE_ASPECT_RATIO")
|
|
(values
|
|
'("auto" "CLUTTER_VIDEO_TEXTURE_AUTO")
|
|
'("square" "CLUTTER_VIDEO_TEXTURE_SQUARE")
|
|
'("fourbythree" "CLUTTER_VIDEO_TEXTURE_FOURBYTHREE")
|
|
'("anamorphic" "CLUTTER_VIDEO_TEXTURE_ANAMORPHIC")
|
|
'("dvb" "CLUTTER_VIDEO_TEXTURE_DVB")
|
|
)
|
|
)
|
|
|
|
(define-enum VideoTextureError
|
|
(in-module "Clutter")
|
|
(c-name "ClutterVideoTextureError")
|
|
(gtype-id "CLUTTER_TYPE_VIDEO_TEXTURE_ERROR")
|
|
(values
|
|
'("audio-plugin" "CLUTTER_VIDEO_TEXTURE_ERROR_AUDIO_PLUGIN")
|
|
'("no-plugin-for-file" "CLUTTER_VIDEO_TEXTURE_ERROR_NO_PLUGIN_FOR_FILE")
|
|
'("video-plugin" "CLUTTER_VIDEO_TEXTURE_ERROR_VIDEO_PLUGIN")
|
|
'("audio-busy" "CLUTTER_VIDEO_TEXTURE_ERROR_AUDIO_BUSY")
|
|
'("broken-file" "CLUTTER_VIDEO_TEXTURE_ERROR_BROKEN_FILE")
|
|
'("file-generic" "CLUTTER_VIDEO_TEXTURE_ERROR_FILE_GENERIC")
|
|
'("file-permission" "CLUTTER_VIDEO_TEXTURE_ERROR_FILE_PERMISSION")
|
|
'("file-encrypted" "CLUTTER_VIDEO_TEXTURE_ERROR_FILE_ENCRYPTED")
|
|
'("file-not-found" "CLUTTER_VIDEO_TEXTURE_ERROR_FILE_NOT_FOUND")
|
|
'("dvd-encrypted" "CLUTTER_VIDEO_TEXTURE_ERROR_DVD_ENCRYPTED")
|
|
'("invalid-device" "CLUTTER_VIDEO_TEXTURE_ERROR_INVALID_DEVICE")
|
|
'("unknown-host" "CLUTTER_VIDEO_TEXTURE_ERROR_UNKNOWN_HOST")
|
|
'("network-unreachable" "CLUTTER_VIDEO_TEXTURE_ERROR_NETWORK_UNREACHABLE")
|
|
'("connection-refused" "CLUTTER_VIDEO_TEXTURE_ERROR_CONNECTION_REFUSED")
|
|
'("unvalid-location" "CLUTTER_VIDEO_TEXTURE_ERROR_UNVALID_LOCATION")
|
|
'("generic" "CLUTTER_VIDEO_TEXTURE_ERROR_GENERIC")
|
|
'("codec-not-handled" "CLUTTER_VIDEO_TEXTURE_ERROR_CODEC_NOT_HANDLED")
|
|
'("audio-only" "CLUTTER_VIDEO_TEXTURE_ERROR_AUDIO_ONLY")
|
|
'("cannot-capture" "CLUTTER_VIDEO_TEXTURE_ERROR_CANNOT_CAPTURE")
|
|
'("read-error" "CLUTTER_VIDEO_TEXTURE_ERROR_READ_ERROR")
|
|
'("plugin-load" "CLUTTER_VIDEO_TEXTURE_ERROR_PLUGIN_LOAD")
|
|
'("still-image" "CLUTTER_VIDEO_TEXTURE_ERROR_STILL_IMAGE")
|
|
'("empty-file" "CLUTTER_VIDEO_TEXTURE_ERROR_EMPTY_FILE")
|
|
)
|
|
)
|
|
|
|
(define-enum VideoTextureMetadataType
|
|
(in-module "Clutter")
|
|
(c-name "ClutterVideoTextureMetadataType")
|
|
(gtype-id "CLUTTER_TYPE_VIDEO_TEXTURE_METADATA_TYPE")
|
|
(values
|
|
'("title" "CLUTTER_INFO_TITLE")
|
|
'("artist" "CLUTTER_INFO_ARTIST")
|
|
'("year" "CLUTTER_INFO_YEAR")
|
|
'("album" "CLUTTER_INFO_ALBUM")
|
|
'("duration" "CLUTTER_INFO_DURATION")
|
|
'("track-number" "CLUTTER_INFO_TRACK_NUMBER")
|
|
'("has-video" "CLUTTER_INFO_HAS_VIDEO")
|
|
'("dimension-x" "CLUTTER_INFO_DIMENSION_X")
|
|
'("dimension-y" "CLUTTER_INFO_DIMENSION_Y")
|
|
'("video-bitrate" "CLUTTER_INFO_VIDEO_BITRATE")
|
|
'("video-codec" "CLUTTER_INFO_VIDEO_CODEC")
|
|
'("fps" "CLUTTER_INFO_FPS")
|
|
'("has-audio" "CLUTTER_INFO_HAS_AUDIO")
|
|
'("audio-bitrate" "CLUTTER_INFO_AUDIO_BITRATE")
|
|
'("audio-codec" "CLUTTER_INFO_AUDIO_CODEC")
|
|
)
|
|
)
|
|
|
|
;; Objects
|
|
|
|
(define-object CloneTexture
|
|
(in-module "Clutter")
|
|
(parent "ClutterElement")
|
|
(c-name "ClutterCloneTexture")
|
|
(gtype-id "CLUTTER_TYPE_CLONE_TEXTURE")
|
|
)
|
|
|
|
(define-object Element
|
|
(in-module "Clutter")
|
|
(parent "GObject")
|
|
(c-name "ClutterElement")
|
|
(gtype-id "CLUTTER_TYPE_ELEMENT")
|
|
)
|
|
|
|
(define-object Group
|
|
(in-module "Clutter")
|
|
(parent "ClutterElement")
|
|
(c-name "ClutterGroup")
|
|
(gtype-id "CLUTTER_TYPE_GROUP")
|
|
)
|
|
|
|
(define-object Label
|
|
(in-module "Clutter")
|
|
(parent "ClutterTexture")
|
|
(c-name "ClutterLabel")
|
|
(gtype-id "CLUTTER_TYPE_LABEL")
|
|
)
|
|
|
|
(define-object Rectangle
|
|
(in-module "Clutter")
|
|
(parent "ClutterElement")
|
|
(c-name "ClutterRectangle")
|
|
(gtype-id "CLUTTER_TYPE_RECTANGLE")
|
|
)
|
|
|
|
(define-object Stage
|
|
(in-module "Clutter")
|
|
(parent "ClutterGroup")
|
|
(c-name "ClutterStage")
|
|
(gtype-id "CLUTTER_TYPE_STAGE")
|
|
)
|
|
|
|
(define-object Texture
|
|
(in-module "Clutter")
|
|
(parent "ClutterElement")
|
|
(c-name "ClutterTexture")
|
|
(gtype-id "CLUTTER_TYPE_TEXTURE")
|
|
)
|
|
|
|
(define-object Timeline
|
|
(in-module "Clutter")
|
|
(parent "GObject")
|
|
(c-name "ClutterTimeline")
|
|
(gtype-id "CLUTTER_TYPE_TIMELINE")
|
|
)
|
|
|
|
(define-object VideoTexture
|
|
(in-module "Clutter")
|
|
(parent "ClutterTexture")
|
|
(c-name "ClutterVideoTexture")
|
|
(gtype-id "CLUTTER_TYPE_VIDEO_TEXTURE")
|
|
)
|
|
|
|
;; Pointers
|
|
|
|
|
|
|
|
;; Unsupported
|
|
|
|
|
|
|