mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
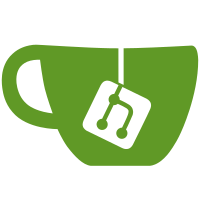
With the recent change to internal floating point values, ClutterUnit has become a redundant type, defined to be a float. All integer entry points are being internally converted to floating point values to be passed to the GL pipeline with the least amount of conversion. ClutterUnit is thus exposed as just a "pixel with fractionary bits", and not -- as users might think -- as generic, resolution and device independent units. not that it was the case, but a definitive amount of people was convinced it did provide this "feature", and was flummoxed about the mere existence of this type. So, having ClutterUnit exposed in the public API doubles the entry points and has the following disadvantages: - we have to maintain twice the amount of entry points in ClutterActor - we still do an integer-to-float implicit conversion - we introduce a weird impedance between pixels and "pixels with fractionary bits" - language bindings will have to choose what to bind, and resort to manually overriding the API + *except* for language bindings based on GObject-Introspection, as they cannot do manual overrides, thus will replicate the entire set of entry points For these reason, we should coalesces every Actor entry point for pixels and for ClutterUnit into a single entry point taking a float, like: void clutter_actor_set_x (ClutterActor *self, gfloat x); void clutter_actor_get_size (ClutterActor *self, gfloat *width, gfloat *height); gfloat clutter_actor_get_height (ClutterActor *self); etc. The issues I have identified are: - we'll have a two cases of compiler warnings: - printf() format of the return values from %d to %f - clutter_actor_get_size() taking floats instead of unsigned ints - we'll have a problem with varargs when passing an integer instead of a floating point value, except on 64bit platforms where the size of a float is the same as the size of an int To be clear: the *intent* of the API should not change -- we still use pixels everywhere -- but: - we remove ambiguity in the API with regard to pixels and units - we remove entry points we get to maintain for the whole 1.0 version of the API - we make things simpler to bind for both manual language bindings and automatic (gobject-introspection based) ones - we have the simplest API possible while still exposing the capabilities of the underlying GL implementation
333 lines
9.7 KiB
C
333 lines
9.7 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2006 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#if !defined(__CLUTTER_H_INSIDE__) && !defined(CLUTTER_COMPILATION)
|
|
#error "Only <clutter/clutter.h> can be included directly."
|
|
#endif
|
|
|
|
#ifndef __CLUTTER_TYPES_H__
|
|
#define __CLUTTER_TYPES_H__
|
|
|
|
#include <glib-object.h>
|
|
#include <clutter/clutter-units.h>
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_GEOMETRY (clutter_geometry_get_type ())
|
|
#define CLUTTER_TYPE_KNOT (clutter_knot_get_type ())
|
|
#define CLUTTER_TYPE_VERTEX (clutter_vertex_get_type ())
|
|
|
|
/* Forward delarations to avoid header catch 22's */
|
|
typedef struct _ClutterActor ClutterActor;
|
|
typedef struct _ClutterStage ClutterStage;
|
|
typedef struct _ClutterContainer ClutterContainer; /* dummy */
|
|
typedef struct _ClutterChildMeta ClutterChildMeta;
|
|
|
|
/**
|
|
* ClutterGravity:
|
|
* @CLUTTER_GRAVITY_NONE: Do not apply any gravity
|
|
* @CLUTTER_GRAVITY_NORTH: Scale from topmost downwards
|
|
* @CLUTTER_GRAVITY_NORTH_EAST: Scale from the top right corner
|
|
* @CLUTTER_GRAVITY_EAST: Scale from the right side
|
|
* @CLUTTER_GRAVITY_SOUTH_EAST: Scale from the bottom right corner
|
|
* @CLUTTER_GRAVITY_SOUTH: Scale from the bottom upwards
|
|
* @CLUTTER_GRAVITY_SOUTH_WEST: Scale from the bottom left corner
|
|
* @CLUTTER_GRAVITY_WEST: Scale from the left side
|
|
* @CLUTTER_GRAVITY_NORTH_WEST: Scale from the top left corner
|
|
* @CLUTTER_GRAVITY_CENTER: Scale from the center.
|
|
*
|
|
* Gravity of the scaling operations. When a gravity different than
|
|
* %CLUTTER_GRAVITY_NONE is used, an actor is scaled keeping the position
|
|
* of the specified portion at the same coordinates.
|
|
*
|
|
* Since: 0.2
|
|
*/
|
|
typedef enum { /*< prefix=CLUTTER_GRAVITY >*/
|
|
CLUTTER_GRAVITY_NONE = 0,
|
|
CLUTTER_GRAVITY_NORTH,
|
|
CLUTTER_GRAVITY_NORTH_EAST,
|
|
CLUTTER_GRAVITY_EAST,
|
|
CLUTTER_GRAVITY_SOUTH_EAST,
|
|
CLUTTER_GRAVITY_SOUTH,
|
|
CLUTTER_GRAVITY_SOUTH_WEST,
|
|
CLUTTER_GRAVITY_WEST,
|
|
CLUTTER_GRAVITY_NORTH_WEST,
|
|
CLUTTER_GRAVITY_CENTER
|
|
} ClutterGravity;
|
|
|
|
typedef struct _ClutterGeometry ClutterGeometry;
|
|
typedef struct _ClutterKnot ClutterKnot;
|
|
typedef struct _ClutterVertex ClutterVertex;
|
|
|
|
/**
|
|
* ClutterGeometry:
|
|
* @x: X coordinate of the top left corner of an actor
|
|
* @y: Y coordinate of the top left corner of an actor
|
|
* @width: width of an actor
|
|
* @height: height of an actor
|
|
*
|
|
* The rectangle containing an actor's bounding box, measured in pixels.
|
|
*/
|
|
struct _ClutterGeometry
|
|
{
|
|
/*< public >*/
|
|
gint x;
|
|
gint y;
|
|
guint width;
|
|
guint height;
|
|
};
|
|
|
|
GType clutter_geometry_get_type (void) G_GNUC_CONST;
|
|
|
|
|
|
/**
|
|
* ClutterVertex:
|
|
* @x: X coordinate of the vertex
|
|
* @y: Y coordinate of the vertex
|
|
* @z: Z coordinate of the vertex
|
|
*
|
|
* Vertex of an actor in 3D space, expressed in pixels
|
|
*
|
|
* Since: 0.4
|
|
*/
|
|
struct _ClutterVertex
|
|
{
|
|
gfloat x;
|
|
gfloat y;
|
|
gfloat z;
|
|
};
|
|
|
|
GType clutter_vertex_get_type (void) G_GNUC_CONST;
|
|
|
|
/**
|
|
* ClutterKnot:
|
|
* @x: X coordinate of the knot
|
|
* @y: Y coordinate of the knot
|
|
*
|
|
* Point in a path behaviour.
|
|
*
|
|
* Since: 0.2
|
|
*/
|
|
struct _ClutterKnot
|
|
{
|
|
gint x;
|
|
gint y;
|
|
};
|
|
|
|
GType clutter_knot_get_type (void) G_GNUC_CONST;
|
|
ClutterKnot *clutter_knot_copy (const ClutterKnot *knot);
|
|
void clutter_knot_free (ClutterKnot *knot);
|
|
gboolean clutter_knot_equal (const ClutterKnot *knot_a,
|
|
const ClutterKnot *knot_b);
|
|
|
|
/**
|
|
* ClutterRotateAxis:
|
|
* @CLUTTER_X_AXIS: Rotate around the X axis
|
|
* @CLUTTER_Y_AXIS: Rotate around the Y axis
|
|
* @CLUTTER_Z_AXIS: Rotate around the Z axis
|
|
*
|
|
* Axis of a rotation.
|
|
*
|
|
* Since: 0.4
|
|
*/
|
|
typedef enum { /*< prefix=CLUTTER >*/
|
|
CLUTTER_X_AXIS,
|
|
CLUTTER_Y_AXIS,
|
|
CLUTTER_Z_AXIS
|
|
} ClutterRotateAxis;
|
|
|
|
/**
|
|
* ClutterRotateDirection:
|
|
* @CLUTTER_ROTATE_CW: Clockwise rotation
|
|
* @CLUTTER_ROTATE_CCW: Counter-clockwise rotation
|
|
*
|
|
* Direction of a rotation.
|
|
*
|
|
* Since: 0.4
|
|
*/
|
|
typedef enum { /*< prefix=CLUTTER_ROTATE >*/
|
|
CLUTTER_ROTATE_CW,
|
|
CLUTTER_ROTATE_CCW
|
|
} ClutterRotateDirection;
|
|
|
|
/**
|
|
* ClutterRequestMode:
|
|
* @CLUTTER_REQUEST_HEIGHT_FOR_WIDTH: Height for width requests
|
|
* @CLUTTER_REQUEST_WIDTH_FOR_HEIGHT: Width for height requests
|
|
*
|
|
* Specifies the type of requests for a #ClutterActor.
|
|
*
|
|
* Since: 0.8
|
|
*/
|
|
typedef enum {
|
|
CLUTTER_REQUEST_HEIGHT_FOR_WIDTH,
|
|
CLUTTER_REQUEST_WIDTH_FOR_HEIGHT
|
|
} ClutterRequestMode;
|
|
|
|
/**
|
|
* ClutterAnimationMode:
|
|
* @CLUTTER_CUSTOM_MODE: custom progress function
|
|
* @CLUTTER_LINEAR: linear tweening
|
|
* @CLUTTER_EASE_IN_QUAD: quadratic tweening
|
|
* @CLUTTER_EASE_OUT_QUAD: quadratic tweening, inverse of
|
|
* %CLUTTER_EASE_IN_QUAD
|
|
* @CLUTTER_EASE_IN_OUT_QUAD: quadratic tweening, combininig
|
|
* %CLUTTER_EASE_IN_QUAD and %CLUTTER_EASE_OUT_QUAD
|
|
* @CLUTTER_EASE_IN_CUBIC: cubic tweening
|
|
* @CLUTTER_EASE_OUT_CUBIC: cubic tweening, invers of
|
|
* %CLUTTER_EASE_IN_CUBIC
|
|
* @CLUTTER_EASE_IN_OUT_CUBIC: cubic tweening, combining
|
|
* %CLUTTER_EASE_IN_CUBIC and %CLUTTER_EASE_OUT_CUBIC
|
|
* @CLUTTER_EASE_IN_QUART: quartic tweening
|
|
* @CLUTTER_EASE_OUT_QUART: quartic tweening, inverse of
|
|
* %CLUTTER_EASE_IN_QUART
|
|
* @CLUTTER_EASE_IN_OUT_QUART: quartic tweening, combining
|
|
* %CLUTTER_EASE_IN_QUART and %CLUTTER_EASE_OUT_QUART
|
|
* @CLUTTER_EASE_IN_QUINT: quintic tweening
|
|
* @CLUTTER_EASE_OUT_QUINT: quintic tweening, inverse of
|
|
* %CLUTTER_EASE_IN_QUINT
|
|
* @CLUTTER_EASE_IN_OUT_QUINT: fifth power tweening, combining
|
|
* %CLUTTER_EASE_IN_QUINT and %CLUTTER_EASE_OUT_QUINT
|
|
* @CLUTTER_EASE_IN_SINE: sinusoidal tweening
|
|
* @CLUTTER_EASE_OUT_SINE: sinusoidal tweening, inverse of
|
|
* %CLUTTER_EASE_IN_SINE
|
|
* @CLUTTER_EASE_IN_OUT_SINE: sine wave tweening, combining
|
|
* %CLUTTER_EASE_IN_SINE and %CLUTTER_EASE_OUT_SINE
|
|
* @CLUTTER_EASE_IN_EXPO: exponential tweening
|
|
* @CLUTTER_EASE_OUT_EXPO: exponential tweening, inverse of
|
|
* %CLUTTER_EASE_IN_EXPO
|
|
* @CLUTTER_EASE_IN_OUT_EXPO: exponential tweening, combining
|
|
* %CLUTTER_EASE_IN_EXPO and %CLUTTER_EASE_OUT_EXPO
|
|
* @CLUTTER_EASE_IN_CIRC: circular tweening
|
|
* @CLUTTER_EASE_OUT_CIRC: circular tweening, inverse of
|
|
* %CLUTTER_EASE_IN_CIRC
|
|
* @CLUTTER_EASE_IN_OUT_CIRC: circular tweening, combining
|
|
* %CLUTTER_EASE_IN_CIRC and %CLUTTER_EASE_OUT_CIRC
|
|
* @CLUTTER_EASE_IN_ELASTIC: elastic tweening, with offshoot on start
|
|
* @CLUTTER_EASE_OUT_ELASTIC: elastic tweening, with offshoot on end
|
|
* @CLUTTER_EASE_IN_OUT_ELASTIC: elastic tweening with offshoot on both ends
|
|
* @CLUTTER_EASE_IN_BACK: overshooting cubic tweening, with
|
|
* backtracking on start
|
|
* @CLUTTER_EASE_OUT_BACK: overshooting cubic tweening, with
|
|
* backtracking on end
|
|
* @CLUTTER_EASE_IN_OUT_BACK: overshooting cubic tweening, with
|
|
* backtracking on both ends
|
|
* @CLUTTER_EASE_IN_BOUNCE: exponentially decaying parabolic (bounce)
|
|
* tweening, with bounce on start
|
|
* @CLUTTER_EASE_OUT_BOUNCE: exponentially decaying parabolic (bounce)
|
|
* tweening, with bounce on end
|
|
* @CLUTTER_EASE_IN_OUT_BOUNCE: exponentially decaying parabolic (bounce)
|
|
* tweening, with bounce on both ends
|
|
* @CLUTTER_ANIMATION_LAST: last animation mode, used as a guard for
|
|
* registered global alpha functions
|
|
*
|
|
* The animation modes used by #ClutterAlpha and #ClutterAnimation. This
|
|
* enumeration can be expanded in later versions of Clutter. See the
|
|
* #ClutterAlpha documentation for a graph of all the animation modes.
|
|
*
|
|
* Every global alpha function registered using clutter_alpha_register_func()
|
|
* or clutter_alpha_register_closure() will have a logical id greater than
|
|
* %CLUTTER_ANIMATION_LAST.
|
|
*
|
|
* Since: 1.0
|
|
*/
|
|
typedef enum {
|
|
CLUTTER_CUSTOM_MODE = 0,
|
|
|
|
/* linear */
|
|
CLUTTER_LINEAR,
|
|
|
|
/* quadratic */
|
|
CLUTTER_EASE_IN_QUAD,
|
|
CLUTTER_EASE_OUT_QUAD,
|
|
CLUTTER_EASE_IN_OUT_QUAD,
|
|
|
|
/* cubic */
|
|
CLUTTER_EASE_IN_CUBIC,
|
|
CLUTTER_EASE_OUT_CUBIC,
|
|
CLUTTER_EASE_IN_OUT_CUBIC,
|
|
|
|
/* quartic */
|
|
CLUTTER_EASE_IN_QUART,
|
|
CLUTTER_EASE_OUT_QUART,
|
|
CLUTTER_EASE_IN_OUT_QUART,
|
|
|
|
/* quintic */
|
|
CLUTTER_EASE_IN_QUINT,
|
|
CLUTTER_EASE_OUT_QUINT,
|
|
CLUTTER_EASE_IN_OUT_QUINT,
|
|
|
|
/* sinusoidal */
|
|
CLUTTER_EASE_IN_SINE,
|
|
CLUTTER_EASE_OUT_SINE,
|
|
CLUTTER_EASE_IN_OUT_SINE,
|
|
|
|
/* exponential */
|
|
CLUTTER_EASE_IN_EXPO,
|
|
CLUTTER_EASE_OUT_EXPO,
|
|
CLUTTER_EASE_IN_OUT_EXPO,
|
|
|
|
/* circular */
|
|
CLUTTER_EASE_IN_CIRC,
|
|
CLUTTER_EASE_OUT_CIRC,
|
|
CLUTTER_EASE_IN_OUT_CIRC,
|
|
|
|
/* elastic */
|
|
CLUTTER_EASE_IN_ELASTIC,
|
|
CLUTTER_EASE_OUT_ELASTIC,
|
|
CLUTTER_EASE_IN_OUT_ELASTIC,
|
|
|
|
/* overshooting cubic */
|
|
CLUTTER_EASE_IN_BACK,
|
|
CLUTTER_EASE_OUT_BACK,
|
|
CLUTTER_EASE_IN_OUT_BACK,
|
|
|
|
/* exponentially decaying parabolic */
|
|
CLUTTER_EASE_IN_BOUNCE,
|
|
CLUTTER_EASE_OUT_BOUNCE,
|
|
CLUTTER_EASE_IN_OUT_BOUNCE,
|
|
|
|
/* guard, before registered alpha functions */
|
|
CLUTTER_ANIMATION_LAST
|
|
} ClutterAnimationMode;
|
|
|
|
/**
|
|
* ClutterFontFlags:
|
|
* @CLUTTER_FONT_MIPMAPPING: Set to use mipmaps for the glyph cache textures.
|
|
* @CLUTTER_FONT_HINTING: Set to enable hinting on the glyphs.
|
|
*
|
|
* Runtime flags to change the font quality. To be used with
|
|
* clutter_set_font_flags().
|
|
*
|
|
* Since: 1.0
|
|
*/
|
|
typedef enum
|
|
{
|
|
CLUTTER_FONT_MIPMAPPING = (1 << 0),
|
|
CLUTTER_FONT_HINTING = (1 << 1),
|
|
} ClutterFontFlags;
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __CLUTTER_TYPES_H__ */
|