mirror of
https://github.com/brl/mutter.git
synced 2024-12-04 22:00:42 -05:00
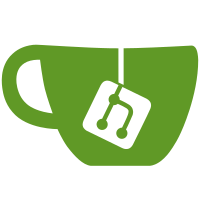
Sometimes it makes no sense to have a shared pointer device, for example when they have no set region occupying the global stage coordinate space. This applies to for example window screen cast based pointer device regions - they are always local to the window, and have no position. We do need shared absolute devices in some cases though, primarily multi-head remote desktop, where it must be possible to keep a button reliably pressed when crossing monitors that have their own corresponding regions. To handle this, outsource all this policy to the one who drives the emulated input devices. Remote desktop sessions where the screen casts correspond to specific monitors (physical or virtual), we need to make sure they map to the stage coordinate space, while for window screencast or area screencasts, we create standalone absolute pointer devices with a single region each. Part-of: <https://gitlab.gnome.org/GNOME/mutter/-/merge_requests/3228>
68 lines
2.8 KiB
C
68 lines
2.8 KiB
C
/* -*- mode: C; c-file-style: "gnu"; indent-tabs-mode: nil; -*- */
|
|
|
|
/*
|
|
* Copyright (C) 2015-2017 Red Hat Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include "backends/meta-screen-cast.h"
|
|
|
|
#include "backends/meta-backend-types.h"
|
|
#include "backends/meta-screen-cast-stream.h"
|
|
#include "meta/meta-remote-access-controller.h"
|
|
|
|
typedef enum _MetaScreenCastSessionType
|
|
{
|
|
META_SCREEN_CAST_SESSION_TYPE_NORMAL,
|
|
META_SCREEN_CAST_SESSION_TYPE_REMOTE_DESKTOP,
|
|
} MetaScreenCastSessionType;
|
|
|
|
#define META_TYPE_SCREEN_CAST_SESSION (meta_screen_cast_session_get_type ())
|
|
G_DECLARE_FINAL_TYPE (MetaScreenCastSession, meta_screen_cast_session,
|
|
META, SCREEN_CAST_SESSION,
|
|
MetaDBusScreenCastSessionSkeleton)
|
|
|
|
#define META_TYPE_SCREEN_CAST_SESSION_HANDLE (meta_screen_cast_session_handle_get_type ())
|
|
G_DECLARE_FINAL_TYPE (MetaScreenCastSessionHandle,
|
|
meta_screen_cast_session_handle,
|
|
META, SCREEN_CAST_SESSION_HANDLE,
|
|
MetaRemoteAccessHandle)
|
|
|
|
char * meta_screen_cast_session_get_object_path (MetaScreenCastSession *session);
|
|
|
|
char * meta_screen_cast_session_get_peer_name (MetaScreenCastSession *session);
|
|
|
|
MetaScreenCastSessionType meta_screen_cast_session_get_session_type (MetaScreenCastSession *session);
|
|
|
|
MetaRemoteDesktopSession * meta_screen_cast_session_get_remote_desktop_session (MetaScreenCastSession *session);
|
|
|
|
gboolean meta_screen_cast_session_start (MetaScreenCastSession *session,
|
|
GError **error);
|
|
|
|
gboolean meta_screen_cast_session_is_active (MetaScreenCastSession *session);
|
|
|
|
GList * meta_screen_cast_session_peek_streams (MetaScreenCastSession *session);
|
|
|
|
MetaScreenCastStream * meta_screen_cast_session_get_stream (MetaScreenCastSession *session,
|
|
const char *path);
|
|
|
|
MetaScreenCast * meta_screen_cast_session_get_screen_cast (MetaScreenCastSession *session);
|
|
|
|
void meta_screen_cast_session_set_disable_animations (MetaScreenCastSession *session,
|
|
gboolean disable_animations);
|