mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
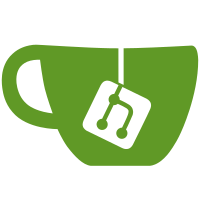
CoglTexture2D had an assert to verify that the EGL winsys was being used. This doesn't make any sense any more because the EGL winsys can't be used directly but instead it is just a base winsys for the platform winsys's. To fix this this patch adds a set of 'criteria' flags to each winsys, one of which is 'uses EGL'. CoglTexture2D can use this to determine if the winsys is supported. Eventually we might want to expose these flags publically so that an application can select a winsys based on certain conditions. For example, an application may need a winsys that uses X or EGL but doesn't care exactly which one it is. Reviewed-by: Robert Bragg <robert@linux.intel.com>
177 lines
4.5 KiB
C
177 lines
4.5 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_WINSYS_PRIVATE_H
|
|
#define __COGL_WINSYS_PRIVATE_H
|
|
|
|
#include "cogl-renderer.h"
|
|
#include "cogl-onscreen.h"
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
#include "cogl-texture-pixmap-x11-private.h"
|
|
#endif
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
#include <X11/Xutil.h>
|
|
#include "cogl-texture-pixmap-x11-private.h"
|
|
#endif
|
|
|
|
GQuark
|
|
_cogl_winsys_error_quark (void);
|
|
|
|
#define COGL_WINSYS_ERROR (_cogl_winsys_error_quark ())
|
|
|
|
typedef enum { /*< prefix=COGL_WINSYS_ERROR >*/
|
|
COGL_WINSYS_ERROR_INIT,
|
|
COGL_WINSYS_ERROR_CREATE_CONTEXT,
|
|
COGL_WINSYS_ERROR_CREATE_ONSCREEN,
|
|
} CoglWinsysError;
|
|
|
|
typedef enum
|
|
{
|
|
COGL_WINSYS_RECTANGLE_STATE_UNKNOWN,
|
|
COGL_WINSYS_RECTANGLE_STATE_DISABLE,
|
|
COGL_WINSYS_RECTANGLE_STATE_ENABLE
|
|
} CoglWinsysRectangleState;
|
|
|
|
/* These criteria flags are hard-coded features of the winsys
|
|
regardless of the underlying driver or GPU. We might eventually
|
|
want to use these in a mechanism for the application to specify
|
|
criteria for the winsys instead of a specific winsys but for now
|
|
they are only used internally to assert that an EGL winsys is
|
|
selected */
|
|
typedef enum
|
|
{
|
|
COGL_WINSYS_CRITERIA_USES_X11 = (1 << 0),
|
|
COGL_WINSYS_CRITERIA_USES_XLIB = (1 << 1),
|
|
COGL_WINSYS_CRITERIA_USES_EGL = (1 << 2)
|
|
} CoglWinsysCriteria;
|
|
|
|
typedef struct _CoglWinsysVtable
|
|
{
|
|
CoglWinsysID id;
|
|
CoglWinsysCriteria criteria;
|
|
|
|
const char *name;
|
|
|
|
/* Required functions */
|
|
|
|
CoglFuncPtr
|
|
(*renderer_get_proc_address) (CoglRenderer *renderer,
|
|
const char *name);
|
|
|
|
gboolean
|
|
(*renderer_connect) (CoglRenderer *renderer, GError **error);
|
|
|
|
void
|
|
(*renderer_disconnect) (CoglRenderer *renderer);
|
|
|
|
gboolean
|
|
(*display_setup) (CoglDisplay *display, GError **error);
|
|
|
|
void
|
|
(*display_destroy) (CoglDisplay *display);
|
|
|
|
gboolean
|
|
(*context_init) (CoglContext *context, GError **error);
|
|
|
|
void
|
|
(*context_deinit) (CoglContext *context);
|
|
|
|
gboolean
|
|
(*onscreen_init) (CoglOnscreen *onscreen, GError **error);
|
|
|
|
void
|
|
(*onscreen_deinit) (CoglOnscreen *onscreen);
|
|
|
|
void
|
|
(*onscreen_bind) (CoglOnscreen *onscreen);
|
|
|
|
void
|
|
(*onscreen_swap_buffers) (CoglOnscreen *onscreen);
|
|
|
|
void
|
|
(*onscreen_update_swap_throttled) (CoglOnscreen *onscreen);
|
|
|
|
void
|
|
(*onscreen_set_visibility) (CoglOnscreen *onscreen,
|
|
gboolean visibility);
|
|
|
|
/* Optional functions */
|
|
|
|
void
|
|
(*onscreen_swap_region) (CoglOnscreen *onscreen,
|
|
const int *rectangles,
|
|
int n_rectangles);
|
|
|
|
#ifdef COGL_HAS_EGL_SUPPORT
|
|
EGLDisplay
|
|
(*context_egl_get_egl_display) (CoglContext *context);
|
|
#endif
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
XVisualInfo *
|
|
(*xlib_get_visual_info) (void);
|
|
#endif
|
|
|
|
guint32
|
|
(*onscreen_x11_get_window_xid) (CoglOnscreen *onscreen);
|
|
|
|
#ifdef COGL_HAS_WIN32_SUPPORT
|
|
HWND
|
|
(*onscreen_win32_get_window) (CoglOnscreen *onscreen);
|
|
#endif
|
|
|
|
unsigned int
|
|
(*onscreen_add_swap_buffers_callback) (CoglOnscreen *onscreen,
|
|
CoglSwapBuffersNotify callback,
|
|
void *user_data);
|
|
|
|
void
|
|
(*onscreen_remove_swap_buffers_callback) (CoglOnscreen *onscreen,
|
|
unsigned int id);
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
gboolean
|
|
(*texture_pixmap_x11_create) (CoglTexturePixmapX11 *tex_pixmap);
|
|
void
|
|
(*texture_pixmap_x11_free) (CoglTexturePixmapX11 *tex_pixmap);
|
|
|
|
gboolean
|
|
(*texture_pixmap_x11_update) (CoglTexturePixmapX11 *tex_pixmap,
|
|
gboolean needs_mipmap);
|
|
|
|
void
|
|
(*texture_pixmap_x11_damage_notify) (CoglTexturePixmapX11 *tex_pixmap);
|
|
|
|
CoglHandle
|
|
(*texture_pixmap_x11_get_texture) (CoglTexturePixmapX11 *tex_pixmap);
|
|
#endif
|
|
|
|
} CoglWinsysVtable;
|
|
|
|
gboolean
|
|
_cogl_winsys_has_feature (CoglWinsysFeature feature);
|
|
|
|
#endif /* __COGL_WINSYS_PRIVATE_H */
|