mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
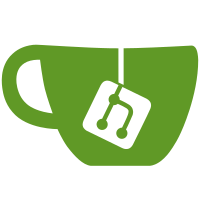
This adds hook points to add global function and variable declarations to either the fragment or vertex shader. The declarations can then be used by subsequent snippets. Only the ‘declarations’ string of the snippet is used and the code is directly put in the global scope near the top of the shader. The reason this is necessary rather than just adding a normal snippet with the declarations is that for the other hooks Cogl assumes that the snippets are independent of each other. That means if a snippet has a replace string then it will assume that it doesn't even need to generate the code for earlier hooks which means the global declarations would be lost. Reviewed-by: Robert Bragg <robert@linux.intel.com> (cherry picked from commit ebb82d5b0bc30487b7101dc66b769160b40f92ca)
118 lines
3.4 KiB
C
118 lines
3.4 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2011, 2013 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see
|
|
* <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*
|
|
* Authors:
|
|
* Neil Roberts <neil@linux.intel.com>
|
|
*/
|
|
|
|
#ifndef __COGL_PIPELINE_SNIPPET_PRIVATE_H
|
|
#define __COGL_PIPELINE_SNIPPET_PRIVATE_H
|
|
|
|
#include <glib.h>
|
|
|
|
#include "cogl-snippet.h"
|
|
#include "cogl-queue.h"
|
|
|
|
typedef struct _CoglPipelineSnippet CoglPipelineSnippet;
|
|
|
|
COGL_LIST_HEAD (CoglPipelineSnippetList, CoglPipelineSnippet);
|
|
|
|
struct _CoglPipelineSnippet
|
|
{
|
|
COGL_LIST_ENTRY (CoglPipelineSnippet) list_node;
|
|
|
|
CoglSnippet *snippet;
|
|
};
|
|
|
|
/* Arguments to pass to _cogl_pipeline_snippet_generate_code() */
|
|
typedef struct
|
|
{
|
|
CoglPipelineSnippetList *snippets;
|
|
|
|
/* Only snippets at this hook point will be used */
|
|
CoglSnippetHook hook;
|
|
|
|
/* The final function to chain on to after all of the snippets code
|
|
has been run */
|
|
const char *chain_function;
|
|
|
|
/* The name of the final generated function */
|
|
const char *final_name;
|
|
|
|
/* A prefix to insert before each generate function name */
|
|
const char *function_prefix;
|
|
|
|
/* The return type of all of the functions, or NULL to use void */
|
|
const char *return_type;
|
|
|
|
/* A variable to return from the functions. The snippets are
|
|
expected to modify this variable. Ignored if return_type is
|
|
NULL */
|
|
const char *return_variable;
|
|
|
|
/* If this is TRUE then it won't allocate a separate variable for
|
|
the return value. Instead it is expected that the snippet will
|
|
modify one of the argument variables directly and that will be
|
|
returned */
|
|
CoglBool return_variable_is_argument;
|
|
|
|
/* The argument names or NULL if there are none */
|
|
const char *arguments;
|
|
|
|
/* The argument types or NULL */
|
|
const char *argument_declarations;
|
|
|
|
/* The string to generate the source into */
|
|
GString *source_buf;
|
|
} CoglPipelineSnippetData;
|
|
|
|
void
|
|
_cogl_pipeline_snippet_generate_code (const CoglPipelineSnippetData *data);
|
|
|
|
void
|
|
_cogl_pipeline_snippet_generate_declarations (GString *declarations_buf,
|
|
CoglSnippetHook hook,
|
|
CoglPipelineSnippetList *list);
|
|
|
|
void
|
|
_cogl_pipeline_snippet_list_free (CoglPipelineSnippetList *list);
|
|
|
|
void
|
|
_cogl_pipeline_snippet_list_add (CoglPipelineSnippetList *list,
|
|
CoglSnippet *snippet);
|
|
|
|
void
|
|
_cogl_pipeline_snippet_list_copy (CoglPipelineSnippetList *dst,
|
|
const CoglPipelineSnippetList *src);
|
|
|
|
void
|
|
_cogl_pipeline_snippet_list_hash (CoglPipelineSnippetList *list,
|
|
unsigned int *hash);
|
|
|
|
CoglBool
|
|
_cogl_pipeline_snippet_list_equal (CoglPipelineSnippetList *list0,
|
|
CoglPipelineSnippetList *list1);
|
|
|
|
#endif /* __COGL_PIPELINE_SNIPPET_PRIVATE_H */
|
|
|