mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
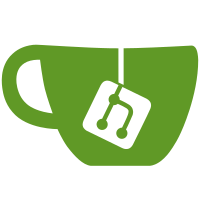
Previously flushing the matrices was performed as part of the framebuffer state. When on GLES2 this matrix flushing is actually diverted so that it only keeps a reference to the intended matrix stack. This is necessary because on GLES2 there are no builtin uniforms so it can't actually flush the matrices until the program for the pipeline is generated. When the matrices are flushed it would store the age of modifications on the matrix stack so that it could detect when the matrix hasn't changed and avoid flushing it. This patch changes it so that the pipeline is responsible for flushing the matrices even when we are using the GL builtins. The same mechanism for detecting unmodified matrix stacks is used in all cases. There is a new CoglMatrixStackCache type which is used to store a reference to the intended matrix stack along with its last flushed age. There are now two of these attached to the CoglContext to track the flushed state for the global matrix builtins and also two for each glsl progend program state to track the flushed state for a program. The framebuffer matrix flush now just updates the intended matrix stacks without actually trying to flush. When a vertex snippet is attached to the pipeline, the GLSL vertend will now avoid using the projection matrix to flip the rendering. This is necessary because any vertex snippet may cause the projection matrix not to be used. Instead the flip is done as a forced final step by multiplying cogl_position_out by a vec4 uniform. This uniform is updated as part of the progend pre_paint depending on whether the framebuffer is offscreen or not. Reviewed-by: Robert Bragg <robert@linux.intel.com>
142 lines
4.2 KiB
C
142 lines
4.2 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2008,2009,2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see
|
|
* <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*
|
|
* Authors:
|
|
* Neil Roberts <neil@linux.intel.com>
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "cogl-context-private.h"
|
|
#include "cogl-pipeline-private.h"
|
|
#include "cogl-pipeline-state-private.h"
|
|
#include "cogl-pipeline-opengl-private.h"
|
|
|
|
#ifdef COGL_PIPELINE_VERTEND_FIXED
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-internal.h"
|
|
#include "cogl-context-private.h"
|
|
#include "cogl-handle.h"
|
|
#include "cogl-program-private.h"
|
|
|
|
const CoglPipelineVertend _cogl_pipeline_fixed_vertend;
|
|
|
|
static gboolean
|
|
_cogl_pipeline_vertend_fixed_start (CoglPipeline *pipeline,
|
|
int n_layers,
|
|
unsigned long pipelines_difference,
|
|
int n_tex_coord_attribs)
|
|
{
|
|
CoglProgram *user_program;
|
|
|
|
_COGL_GET_CONTEXT (ctx, FALSE);
|
|
|
|
if (G_UNLIKELY (COGL_DEBUG_ENABLED (COGL_DEBUG_DISABLE_FIXED)))
|
|
return FALSE;
|
|
|
|
if (ctx->driver == COGL_DRIVER_GLES2)
|
|
return FALSE;
|
|
|
|
/* Vertex snippets are only supported in the GLSL fragend */
|
|
if (_cogl_pipeline_has_vertex_snippets (pipeline))
|
|
return FALSE;
|
|
|
|
/* If there is a user program with a vertex shader then the
|
|
appropriate backend for that language should handle it. We can
|
|
still use the fixed vertex backend if the program only contains
|
|
a fragment shader */
|
|
user_program = cogl_pipeline_get_user_program (pipeline);
|
|
if (user_program != COGL_INVALID_HANDLE &&
|
|
_cogl_program_has_vertex_shader (user_program))
|
|
return FALSE;
|
|
|
|
_cogl_use_vertex_program (0, COGL_PIPELINE_PROGRAM_TYPE_FIXED);
|
|
|
|
return TRUE;
|
|
}
|
|
|
|
static gboolean
|
|
_cogl_pipeline_vertend_fixed_add_layer (CoglPipeline *pipeline,
|
|
CoglPipelineLayer *layer,
|
|
unsigned long layers_difference)
|
|
{
|
|
int unit_index = _cogl_pipeline_layer_get_unit_index (layer);
|
|
CoglTextureUnit *unit = _cogl_get_texture_unit (unit_index);
|
|
|
|
_COGL_GET_CONTEXT (ctx, FALSE);
|
|
|
|
if (layers_difference & COGL_PIPELINE_LAYER_STATE_USER_MATRIX)
|
|
{
|
|
CoglPipelineLayerState state = COGL_PIPELINE_LAYER_STATE_USER_MATRIX;
|
|
CoglPipelineLayer *authority =
|
|
_cogl_pipeline_layer_get_authority (layer, state);
|
|
|
|
_cogl_matrix_stack_set (unit->matrix_stack,
|
|
&authority->big_state->matrix);
|
|
|
|
_cogl_set_active_texture_unit (unit_index);
|
|
|
|
_cogl_matrix_stack_flush_to_gl_builtins (ctx, unit->matrix_stack,
|
|
COGL_MATRIX_TEXTURE,
|
|
FALSE /* enable flip */);
|
|
}
|
|
|
|
return TRUE;
|
|
}
|
|
|
|
static gboolean
|
|
_cogl_pipeline_vertend_fixed_end (CoglPipeline *pipeline,
|
|
unsigned long pipelines_difference)
|
|
{
|
|
_COGL_GET_CONTEXT (ctx, FALSE);
|
|
|
|
if (pipelines_difference & COGL_PIPELINE_STATE_POINT_SIZE)
|
|
{
|
|
CoglPipeline *authority =
|
|
_cogl_pipeline_get_authority (pipeline, COGL_PIPELINE_STATE_POINT_SIZE);
|
|
|
|
if (ctx->point_size_cache != authority->big_state->point_size)
|
|
{
|
|
GE( ctx, glPointSize (authority->big_state->point_size) );
|
|
ctx->point_size_cache = authority->big_state->point_size;
|
|
}
|
|
}
|
|
|
|
return TRUE;
|
|
}
|
|
|
|
const CoglPipelineVertend _cogl_pipeline_fixed_vertend =
|
|
{
|
|
_cogl_pipeline_vertend_fixed_start,
|
|
_cogl_pipeline_vertend_fixed_add_layer,
|
|
_cogl_pipeline_vertend_fixed_end,
|
|
NULL, /* pipeline_change_notify */
|
|
NULL /* layer_change_notify */
|
|
};
|
|
|
|
#endif /* COGL_PIPELINE_VERTEND_FIXED */
|
|
|