mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
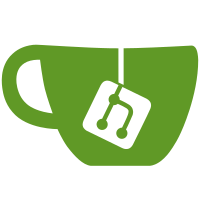
According to the EGL spec, eglGetProcAddress should only be used to retrieve extension functions. It also says that returning non-NULL does not mean the extension is available so you could interpret this as saying that the function is allowed to return garbage for core functions. This seems to happen at least for the Android implementation of EGL. To workaround this the winsys's are now passed down a flag to say whether the function is from the core API. This information is already in the gl-prototypes headers as the minimum core GL version and as a pair of flags to specify whether it is available in core GLES1 and GLES2. If the function is in core the EGL winsys will now avoid using eglGetProcAddress and always fallback to querying the library directly with the GModule API. The GLX winsys is left alone because glXGetProcAddress apparently supports querying core API and extension functions. The WGL winsys could ideally be changed because wglGetProcAddress should also only be used for extension functions but the situation is slightly different because WGL considers anything from GL > 1.1 to be an extension so it would need a bit more information to determine whether to query the function directly from the library. The SDL winsys is also left alone because it's not as easy to portably determine which GL library SDL has chosen to load in order to resolve the symbols directly. Reviewed-by: Robert Bragg <robert@linux.intel.com> (cherry picked from commit 72089730ad06ccdd38a344279a893965ae68cec1) Since we aren't able to break API on the 1.12 branch cogl_get_proc_address is still supported but isn't easily able to determine whether the given name corresponds to a core symbol or not. For now we just assume the symbol being queried isn't part of the core GL api and update the documentation accordingly.
99 lines
2.7 KiB
C
99 lines
2.7 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2011 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_RENDERER_PRIVATE_H
|
|
#define __COGL_RENDERER_PRIVATE_H
|
|
|
|
#include <gmodule.h>
|
|
|
|
#include "cogl-object-private.h"
|
|
#include "cogl-winsys-private.h"
|
|
#include "cogl-internal.h"
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
#include <X11/Xlib.h>
|
|
#endif
|
|
|
|
#if defined (COGL_HAS_EGL_PLATFORM_WAYLAND_SUPPORT)
|
|
#include <wayland-client.h>
|
|
#endif
|
|
|
|
struct _CoglRenderer
|
|
{
|
|
CoglObject _parent;
|
|
CoglBool connected;
|
|
CoglDriver driver_override;
|
|
const CoglWinsysVtable *winsys_vtable;
|
|
CoglWinsysID winsys_id_override;
|
|
GList *constraints;
|
|
|
|
#ifdef COGL_HAS_XLIB_SUPPORT
|
|
Display *foreign_xdpy;
|
|
CoglBool xlib_enable_event_retrieval;
|
|
#endif
|
|
|
|
CoglDriver driver;
|
|
#ifndef HAVE_DIRECTLY_LINKED_GL_LIBRARY
|
|
GModule *libgl_module;
|
|
#endif
|
|
|
|
#if defined (COGL_HAS_EGL_PLATFORM_WAYLAND_SUPPORT)
|
|
struct wl_display *foreign_wayland_display;
|
|
struct wl_compositor *foreign_wayland_compositor;
|
|
struct wl_shell *foreign_wayland_shell;
|
|
#endif
|
|
|
|
#ifdef COGL_HAS_SDL_SUPPORT
|
|
CoglBool sdl_event_type_set;
|
|
uint8_t sdl_event_type;
|
|
#endif
|
|
|
|
/* List of callback functions that will be given every native event */
|
|
GSList *event_filters;
|
|
void *winsys;
|
|
};
|
|
|
|
typedef CoglFilterReturn (* CoglNativeFilterFunc) (void *native_event,
|
|
void *data);
|
|
|
|
CoglFilterReturn
|
|
_cogl_renderer_handle_native_event (CoglRenderer *renderer,
|
|
void *event);
|
|
|
|
void
|
|
_cogl_renderer_add_native_filter (CoglRenderer *renderer,
|
|
CoglNativeFilterFunc func,
|
|
void *data);
|
|
|
|
void
|
|
_cogl_renderer_remove_native_filter (CoglRenderer *renderer,
|
|
CoglNativeFilterFunc func,
|
|
void *data);
|
|
|
|
void *
|
|
_cogl_renderer_get_proc_address (CoglRenderer *renderer,
|
|
const char *name,
|
|
CoglBool in_core);
|
|
|
|
#endif /* __COGL_RENDERER_PRIVATE_H */
|