mirror of
https://github.com/brl/mutter.git
synced 2024-12-04 22:00:42 -05:00
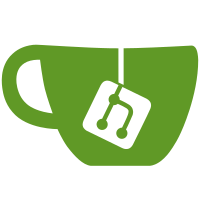
This allows using two separate ICC profiles for one "color profile", which is necessary to properly support color transform calibration profiles from an EFI variable. These types of profiles are intended to be applied using the color transformation matrix (CTM) property on the output, which makes the presented output match sRGB. In order to avoid color profile aware clients making the wrong assumption, we must set the profile exposed externally to be what is the expected perceived result, i.e. sRGB, while still applying CTM from the real ICC profile. The separation is done by introducing a MetaColorCalibration struct, that is filled with relevant data. For profiles coming from EFI, a created profile is practically an sRGB one, but the calibration data comes from EFI, while for other profiles, the calibration data and the ICC profile itself come from the same source. Part-of: <https://gitlab.gnome.org/GNOME/mutter/-/merge_requests/2568>
90 lines
3.5 KiB
C
90 lines
3.5 KiB
C
/*
|
|
* Copyright (C) 2021 Red Hat Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef META_COLOR_PROFILE_H
|
|
#define META_COLOR_PROFILE_H
|
|
|
|
#include <colord.h>
|
|
#include <glib-object.h>
|
|
#include <lcms2.h>
|
|
#include <stdint.h>
|
|
|
|
#include "backends/meta-backend-types.h"
|
|
#include "core/util-private.h"
|
|
|
|
typedef struct _MetaColorCalibration
|
|
{
|
|
gboolean has_vcgt;
|
|
cmsToneCurve *vcgt[3];
|
|
|
|
gboolean has_adaptation_matrix;
|
|
CdMat3x3 adaptation_matrix;
|
|
|
|
char *brightness_profile;
|
|
} MetaColorCalibration;
|
|
|
|
#define META_TYPE_COLOR_PROFILE (meta_color_profile_get_type ())
|
|
G_DECLARE_FINAL_TYPE (MetaColorProfile, meta_color_profile,
|
|
META, COLOR_PROFILE,
|
|
GObject)
|
|
|
|
MetaColorProfile * meta_color_profile_new_from_icc (MetaColorManager *color_manager,
|
|
CdIcc *cd_icc,
|
|
GBytes *raw_bytes,
|
|
MetaColorCalibration *color_calibration);
|
|
|
|
MetaColorProfile * meta_color_profile_new_from_cd_profile (MetaColorManager *color_manager,
|
|
CdProfile *cd_profile,
|
|
CdIcc *cd_icc,
|
|
GBytes *raw_bytes,
|
|
MetaColorCalibration *color_calibration);
|
|
|
|
gboolean meta_color_profile_equals_bytes (MetaColorProfile *color_profile,
|
|
GBytes *bytes);
|
|
|
|
const uint8_t * meta_color_profile_get_data (MetaColorProfile *color_profile);
|
|
|
|
size_t meta_color_profile_get_data_size (MetaColorProfile *color_profile);
|
|
|
|
META_EXPORT_TEST
|
|
CdIcc * meta_color_profile_get_cd_icc (MetaColorProfile *color_profile);
|
|
|
|
CdProfile * meta_color_profile_get_cd_profile (MetaColorProfile *color_profile);
|
|
|
|
gboolean meta_color_profile_is_ready (MetaColorProfile *color_profile);
|
|
|
|
META_EXPORT_TEST
|
|
const char * meta_color_profile_get_id (MetaColorProfile *color_profile);
|
|
|
|
const char * meta_color_profile_get_file_path (MetaColorProfile *color_profile);
|
|
|
|
const char * meta_color_profile_get_brightness_profile (MetaColorProfile *color_profile);
|
|
|
|
MetaGammaLut * meta_color_profile_generate_gamma_lut (MetaColorProfile *color_profile,
|
|
unsigned int temperature,
|
|
size_t lut_size);
|
|
|
|
META_EXPORT_TEST
|
|
const MetaColorCalibration * meta_color_profile_get_calibration (MetaColorProfile *color_profile);
|
|
|
|
MetaColorCalibration * meta_color_calibration_new (CdIcc *cd_icc,
|
|
const CdMat3x3 *adaptation_matrix);
|
|
|
|
void meta_color_calibration_free (MetaColorCalibration *color_calibration);
|
|
|
|
#endif /* META_COLOR_PROFILE_H */
|