mirror of
https://github.com/brl/mutter.git
synced 2024-11-13 01:36:10 -05:00
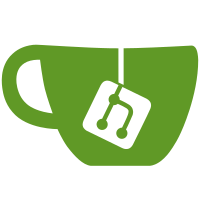
ClutterEffect is an abstract class that should be used to apply effects on generic actors. The ClutterEffect class just defines what an effect should implement; it could be defined as an interface, but we might want to add some default behavior dependent on the internal state at a later point. The effect API applies to any actor, so we need to provide a way to assign an effect to an actor, and let ClutterActor call the Effect methods during the paint sequence. Once an effect is attached to an actor we will perform the paint in this order: • Effect::pre_paint() • Actor::paint signal emission • Effect::post_paint() Since an effect might collide with the Shader class, we either allow a shader or an effect for the time being.
81 lines
2.2 KiB
C
81 lines
2.2 KiB
C
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include <glib.h>
|
|
#include <gmodule.h>
|
|
|
|
#include <clutter/clutter.h>
|
|
|
|
G_MODULE_EXPORT int
|
|
test_rotate_main (int argc, char *argv[])
|
|
{
|
|
ClutterTimeline *timeline;
|
|
ClutterAlpha *alpha;
|
|
ClutterBehaviour *r_behave;
|
|
ClutterActor *stage;
|
|
ClutterActor *hand, *label;
|
|
ClutterColor stage_color = { 0xcc, 0xcc, 0xcc, 0xff };
|
|
gchar *file;
|
|
|
|
clutter_init (&argc, &argv);
|
|
|
|
stage = clutter_stage_get_default ();
|
|
|
|
clutter_stage_set_color (CLUTTER_STAGE (stage),
|
|
&stage_color);
|
|
|
|
/* Make a hand */
|
|
file = g_build_filename (TESTS_DATADIR, "redhand.png", NULL);
|
|
hand = clutter_texture_new_from_file (file, NULL);
|
|
if (!hand)
|
|
g_error("Unable to load '%s'", file);
|
|
|
|
g_free (file);
|
|
|
|
clutter_actor_set_position (hand, 240, 140);
|
|
clutter_actor_show (hand);
|
|
clutter_container_add_actor (CLUTTER_CONTAINER (stage), hand);
|
|
|
|
label = clutter_text_new_with_text ("Mono 16",
|
|
"The Wonder\n"
|
|
"of the\n"
|
|
"Spinning Hand");
|
|
clutter_text_set_line_alignment (CLUTTER_TEXT (label), PANGO_ALIGN_CENTER);
|
|
clutter_actor_set_position (label, 150, 150);
|
|
clutter_actor_set_size (label, 500, 100);
|
|
|
|
clutter_container_add (CLUTTER_CONTAINER (stage), hand, label, NULL);
|
|
|
|
/* Make a timeline */
|
|
timeline = clutter_timeline_new (7692); /* num frames, fps */
|
|
clutter_timeline_set_loop (timeline, TRUE);
|
|
|
|
/* Set an alpha func to power behaviour */
|
|
alpha = clutter_alpha_new_full (timeline, CLUTTER_LINEAR);
|
|
|
|
/* Create a behaviour for that alpha */
|
|
r_behave = clutter_behaviour_rotate_new (alpha,
|
|
CLUTTER_Z_AXIS,
|
|
CLUTTER_ROTATE_CW,
|
|
0.0, 360.0);
|
|
|
|
clutter_behaviour_rotate_set_center (CLUTTER_BEHAVIOUR_ROTATE (r_behave),
|
|
86, 125, 0);
|
|
|
|
/* Apply it to our actor */
|
|
clutter_behaviour_apply (r_behave, hand);
|
|
clutter_behaviour_apply (r_behave, label);
|
|
|
|
/* start the timeline and thus the animations */
|
|
clutter_timeline_start (timeline);
|
|
|
|
clutter_actor_show_all (stage);
|
|
|
|
clutter_main();
|
|
|
|
g_object_unref (r_behave);
|
|
|
|
return 0;
|
|
}
|