mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
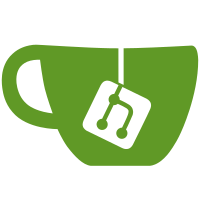
cogl_texture_set_region() and cogl_texture_set_region_from_bitmap() now have a level argument so image data can be uploaded to a specific mipmap level. The prototype for cogl_texture_set_region was also updated to simplify the arguments. The arguments for cogl_texture_set_region_from_bitmap were reordered to be consistent with cogl_texture_set_region with the source related arguments listed first followed by the destination arguments. Reviewed-by: Neil Roberts <neil@linux.intel.com> (cherry picked from commit 3a336a8adcd406b53731a6de0e7d97ba7932c1a8) Note: Public API changes were reverted in cherry-picking this patch
161 lines
5.0 KiB
C
161 lines
5.0 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2009,2010,2011,2012 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*
|
|
* Authors:
|
|
* Neil Roberts <neil@linux.intel.com>
|
|
* Robert Bragg <robert@linux.intel.com>
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include <string.h>
|
|
|
|
#include "cogl-private.h"
|
|
#include "cogl-texture-2d-nop-private.h"
|
|
#include "cogl-texture-2d-private.h"
|
|
#include "cogl-error-private.h"
|
|
|
|
void
|
|
_cogl_texture_2d_nop_free (CoglTexture2D *tex_2d)
|
|
{
|
|
}
|
|
|
|
CoglBool
|
|
_cogl_texture_2d_nop_can_create (CoglContext *ctx,
|
|
int width,
|
|
int height,
|
|
CoglPixelFormat internal_format)
|
|
{
|
|
return TRUE;
|
|
}
|
|
|
|
void
|
|
_cogl_texture_2d_nop_init (CoglTexture2D *tex_2d)
|
|
{
|
|
}
|
|
|
|
CoglTexture2D *
|
|
_cogl_texture_2d_nop_new_with_size (CoglContext *ctx,
|
|
int width,
|
|
int height,
|
|
CoglPixelFormat internal_format,
|
|
CoglError **error)
|
|
{
|
|
return _cogl_texture_2d_create_base (ctx,
|
|
width, height,
|
|
internal_format);
|
|
}
|
|
|
|
CoglTexture2D *
|
|
_cogl_texture_2d_nop_new_from_bitmap (CoglBitmap *bmp,
|
|
CoglPixelFormat internal_format,
|
|
CoglError **error)
|
|
{
|
|
return _cogl_texture_2d_nop_new_with_size (_cogl_bitmap_get_context (bmp),
|
|
cogl_bitmap_get_width (bmp),
|
|
cogl_bitmap_get_height (bmp),
|
|
internal_format,
|
|
error);
|
|
}
|
|
|
|
#if defined (COGL_HAS_EGL_SUPPORT) && defined (EGL_KHR_image_base)
|
|
CoglTexture2D *
|
|
_cogl_egl_texture_2d_nop_new_from_image (CoglContext *ctx,
|
|
int width,
|
|
int height,
|
|
CoglPixelFormat format,
|
|
EGLImageKHR image,
|
|
CoglError **error)
|
|
{
|
|
_cogl_set_error (error,
|
|
COGL_SYSTEM_ERROR,
|
|
COGL_SYSTEM_ERROR_UNSUPPORTED,
|
|
"Creating 2D textures from an EGLImage isn't "
|
|
"supported by the NOP backend");
|
|
return NULL;
|
|
}
|
|
#endif
|
|
|
|
void
|
|
_cogl_texture_2d_nop_flush_legacy_texobj_filters (CoglTexture *tex,
|
|
GLenum min_filter,
|
|
GLenum mag_filter)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_texture_2d_nop_flush_legacy_texobj_wrap_modes (CoglTexture *tex,
|
|
GLenum wrap_mode_s,
|
|
GLenum wrap_mode_t,
|
|
GLenum wrap_mode_p)
|
|
{
|
|
}
|
|
|
|
void
|
|
_cogl_texture_2d_nop_copy_from_framebuffer (CoglTexture2D *tex_2d,
|
|
int src_x,
|
|
int src_y,
|
|
int width,
|
|
int height,
|
|
CoglFramebuffer *src_fb,
|
|
int dst_x,
|
|
int dst_y,
|
|
int level)
|
|
{
|
|
}
|
|
|
|
unsigned int
|
|
_cogl_texture_2d_nop_get_gl_handle (CoglTexture2D *tex_2d)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
void
|
|
_cogl_texture_2d_nop_generate_mipmap (CoglTexture2D *tex_2d)
|
|
{
|
|
}
|
|
|
|
CoglBool
|
|
_cogl_texture_2d_nop_copy_from_bitmap (CoglTexture2D *tex_2d,
|
|
int src_x,
|
|
int src_y,
|
|
int width,
|
|
int height,
|
|
CoglBitmap *bitmap,
|
|
int dst_x,
|
|
int dst_y,
|
|
int level,
|
|
CoglError **error)
|
|
{
|
|
return TRUE;
|
|
}
|
|
|
|
void
|
|
_cogl_texture_2d_nop_get_data (CoglTexture2D *tex_2d,
|
|
CoglPixelFormat format,
|
|
size_t rowstride,
|
|
uint8_t *data)
|
|
{
|
|
}
|