mirror of
https://github.com/brl/mutter.git
synced 2025-07-05 18:39:52 +00:00
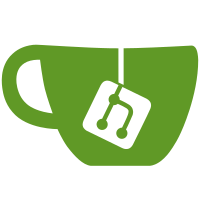
One can add a wl_global filter to a wl_display instance, which can be used to decide what clients should see what globals. This has so far been used to limit a Xwayland specific protocol extension to only Xwayland. In order to expand the logic about what globals are filtered to what clients, introduce a filter manager and port the Xwayland specific protocol filter to this new manager. Tests are added, using a new dummy protocol, to ensure that filtering is working as expected. Part-of: <https://gitlab.gnome.org/GNOME/mutter/-/merge_requests/2810>
105 lines
3.0 KiB
C
105 lines
3.0 KiB
C
/*
|
|
* Copyright 2023 Red Hat
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
|
|
* 02111-1307, USA.
|
|
*/
|
|
|
|
#include "config.h"
|
|
|
|
#include "meta-wayland-filter-manager.h"
|
|
|
|
#include "meta-wayland.h"
|
|
|
|
struct _MetaWaylandFilterManager
|
|
{
|
|
GHashTable *filters;
|
|
};
|
|
|
|
typedef struct _MetaWaylandFilter
|
|
{
|
|
MetaWaylandFilterFunc func;
|
|
gpointer user_data;
|
|
} MetaWaylandFilter;
|
|
|
|
static bool
|
|
global_filter_func (const struct wl_client *client,
|
|
const struct wl_global *global,
|
|
void *user_data)
|
|
{
|
|
MetaWaylandFilterManager *filter_manager = user_data;
|
|
MetaWaylandFilter *filter;
|
|
|
|
filter = g_hash_table_lookup (filter_manager->filters, global);
|
|
if (!filter)
|
|
return true;
|
|
|
|
switch (filter->func (client, global, filter->user_data))
|
|
{
|
|
case META_WAYLAND_ACCESS_ALLOWED:
|
|
return true;
|
|
case META_WAYLAND_ACCESS_DENIED:
|
|
return false;
|
|
}
|
|
|
|
g_assert_not_reached ();
|
|
}
|
|
|
|
MetaWaylandFilterManager *
|
|
meta_wayland_filter_manager_new (MetaWaylandCompositor *compositor)
|
|
{
|
|
struct wl_display *wayland_display =
|
|
meta_wayland_compositor_get_wayland_display (compositor);
|
|
MetaWaylandFilterManager *filter_manager;
|
|
|
|
filter_manager = g_new0 (MetaWaylandFilterManager, 1);
|
|
filter_manager->filters = g_hash_table_new_full (NULL, NULL, NULL, g_free);
|
|
wl_display_set_global_filter (wayland_display,
|
|
global_filter_func, filter_manager);
|
|
|
|
return filter_manager;
|
|
}
|
|
|
|
void
|
|
meta_wayland_filter_manager_free (MetaWaylandFilterManager *filter_manager)
|
|
{
|
|
g_hash_table_unref (filter_manager->filters);
|
|
g_free (filter_manager);
|
|
}
|
|
|
|
void
|
|
meta_wayland_filter_manager_add_global (MetaWaylandFilterManager *filter_manager,
|
|
struct wl_global *global,
|
|
MetaWaylandFilterFunc filter_func,
|
|
gpointer user_data)
|
|
{
|
|
MetaWaylandFilter *filter;
|
|
|
|
g_return_if_fail (!g_hash_table_lookup (filter_manager->filters, global));
|
|
|
|
filter = g_new0 (MetaWaylandFilter, 1);
|
|
filter->func = filter_func;
|
|
filter->user_data = user_data;
|
|
|
|
g_hash_table_insert (filter_manager->filters, global, filter);
|
|
}
|
|
|
|
void
|
|
meta_wayland_filter_manager_remove_global (MetaWaylandFilterManager *filter_manager,
|
|
struct wl_global *global)
|
|
{
|
|
g_hash_table_remove (filter_manager->filters, global);
|
|
}
|