mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
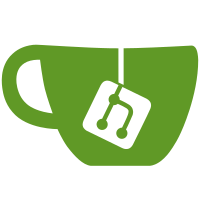
* clutter/cogl/gles/cogl-gles2-wrapper.c: All of the settings and uniforms are now proxied into COGL variables instead of setting the GL uniforms directly. Just before glDrawArrays is executed a shader is generated using the given settings to avoid using 'if' statements. The shaders are cached. * clutter/cogl/gles/cogl-fixed-vertex-shader.glsl: * clutter/cogl/gles/cogl-fixed-fragment-shader.glsl: The shaders are now split into parts using comments instead of 'if' statements so that the simplest shader can be generated on the fly. * clutter/cogl/gles/stringify.sh: Now splits up the shader sources into separate C strings where deliminated by special comments. * clutter/cogl/gles/cogl-program.h: * clutter/cogl/gles/cogl-program.c: A custom shader can no longer be directly linked with the fixed-functionality replacement because the replacement changes depending on the settings. Instead the bound shader is linked with the appropriate replacement shader just before glDrawArrays is executed. The custom uniform variables must also be proxied through COGL variables because their location can change when relinked.
45 lines
1.3 KiB
C
45 lines
1.3 KiB
C
/*
|
|
* Clutter COGL
|
|
*
|
|
* A basic GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2008 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_PROGRAM_H
|
|
#define __COGL_PROGRAM_H
|
|
|
|
#include "cogl-gles2-wrapper.h"
|
|
|
|
typedef struct _CoglProgram CoglProgram;
|
|
|
|
struct _CoglProgram
|
|
{
|
|
guint ref_count;
|
|
|
|
GSList *attached_shaders;
|
|
|
|
char *custom_uniform_names[COGL_GLES2_NUM_CUSTOM_UNIFORMS];
|
|
};
|
|
|
|
CoglProgram *_cogl_program_pointer_from_handle (CoglHandle handle);
|
|
|
|
#endif /* __COGL_PROGRAM_H */
|