mirror of
https://github.com/brl/mutter.git
synced 2024-11-10 07:56:14 -05:00
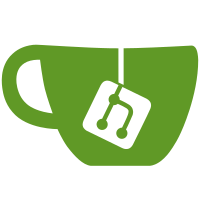
Revert all the work that happened on the master branch.
Sadly, this is the only way to merge the current development branch back
into master.
It is now abundantly clear that I merged the 1.99 branch far too soon,
and that Clutter 2.0 won't happen any time soon, if at all.
Since having the development happen on a separate branch throws a lot of
people into confusion, let's undo the clutter-1.99 → master merge, and
move back the development of Clutter to the master branch.
In order to do so, we need to do some surgery to the Git repository.
First, we do a massive revert in a single commit of all that happened
since the switch to 1.99 and the API version bump done with the
89a2862b05
commit. The history is too long
to be reverted commit by commit without being extremely messy.
216 lines
6.1 KiB
Perl
Executable File
216 lines
6.1 KiB
Perl
Executable File
#!/usr/bin/env perl
|
|
|
|
# Author : Simos Xenitellis <simos at gnome dot org>.
|
|
# Authos : Bastien Nocera <hadess@hadess.net>
|
|
# Version : 1.2
|
|
#
|
|
# Notes : It downloads keysymdef.h from the Internet, if not found locally,
|
|
# Notes : and creates an updated clutter-keysyms.h
|
|
|
|
use strict;
|
|
|
|
my $update_url = 'http://git.clutter-project.org/clutter/plain/clutter/clutter-keysyms-update.pl';
|
|
|
|
# Used for reading the keysymdef symbols.
|
|
my @keysymelements;
|
|
|
|
my $keysymdef_url = 'http://cgit.freedesktop.org/xorg/proto/x11proto/plain/keysymdef.h';
|
|
|
|
if ( ! -f "keysymdef.h" )
|
|
{
|
|
print "Trying to download keysymdef.h from\n", $keysymdef_url, "\n";
|
|
die "Unable to download keysymdef.h: $!"
|
|
unless system("wget -c -O keysymdef.h \"$keysymdef_url\"") == 0;
|
|
print " done.\n\n";
|
|
}
|
|
else
|
|
{
|
|
print "We are using existing keysymdef.h found in this directory.\n";
|
|
print "It is assumed that you took care and it is a recent version\n";
|
|
}
|
|
|
|
my $XF86keysym_url = 'http://cgit.freedesktop.org/xorg/proto/x11proto/plain/XF86keysym.h';
|
|
|
|
if ( ! -f "XF86keysym.h" )
|
|
{
|
|
print "Trying to download XF86keysym.h from\n", $XF86keysym_url, "\n";
|
|
die "Unable to download keysymdef.h: $!\n"
|
|
unless system("wget -c -O XF86keysym.h \"$XF86keysym_url\"") == 0;
|
|
print " done.\n\n";
|
|
}
|
|
else
|
|
{
|
|
print "We are using existing XF86keysym.h found in this directory.\n";
|
|
print "It is assumed that you took care and it is a recent version\n";
|
|
}
|
|
|
|
if ( -f "clutter-keysyms.h" )
|
|
{
|
|
print "There is already a clutter-keysyms.h file in this directory. We are not overwriting it.\n";
|
|
print "Please move it somewhere else in order to run this script.\n";
|
|
die "Exiting...\n\n";
|
|
}
|
|
|
|
die "Could not open file keysymdef.h: $!\n"
|
|
unless open(IN_KEYSYMDEF, "<:utf8", "keysymdef.h");
|
|
|
|
# Output: clutter/clutter/clutter-keysyms.h
|
|
die "Could not open file clutter-keysyms.h: $!\n"
|
|
unless open(OUT_KEYSYMS, ">:utf8", "clutter-keysyms.h");
|
|
|
|
# Output: clutter/clutter/deprecated/clutter-keysyms.h
|
|
die "Could not open file clutter-keysyms-compat.h: $!\n"
|
|
unless open(OUT_KEYSYMS_COMPAT, ">:utf8", "deprecated/clutter-keysyms.h");
|
|
|
|
my $LICENSE_HEADER= <<EOF;
|
|
/* Clutter
|
|
*
|
|
* Copyright (C) 2006, 2007, 2008 OpenedHand Ltd
|
|
* Copyright (C) 2009, 2010 Intel Corp
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses>.
|
|
*/
|
|
|
|
EOF
|
|
|
|
print OUT_KEYSYMS $LICENSE_HEADER;
|
|
print OUT_KEYSYMS_COMPAT $LICENSE_HEADER;
|
|
|
|
print OUT_KEYSYMS<<EOF;
|
|
|
|
/*
|
|
* File auto-generated from script at:
|
|
* $update_url
|
|
*
|
|
* using the input files:
|
|
* $keysymdef_url
|
|
* and
|
|
* $XF86keysym_url
|
|
*/
|
|
|
|
#ifndef __CLUTTER_KEYSYMS_H__
|
|
#define __CLUTTER_KEYSYMS_H__
|
|
|
|
EOF
|
|
|
|
print OUT_KEYSYMS_COMPAT<<EOF;
|
|
/*
|
|
* Compatibility version of clutter-keysyms.h.
|
|
*
|
|
* Since Clutter 1.4, the key symbol defines have been changed to have
|
|
* a KEY_ prefix. This is a compatibility header that is included when
|
|
* deprecated symbols are enabled. Consider porting to the new names
|
|
* instead.
|
|
*/
|
|
|
|
#ifndef __CLUTTER_KEYSYMS_DEPRECATED_H__
|
|
#define __CLUTTER_KEYSYMS_DEPRECATED_H__
|
|
|
|
#ifndef CLUTTER_DISABLE_DEPRECATED
|
|
|
|
EOF
|
|
|
|
while (<IN_KEYSYMDEF>)
|
|
{
|
|
next if ( ! /^#define / );
|
|
|
|
@keysymelements = split(/\s+/);
|
|
die "Internal error, no \@keysymelements: $_\n" unless @keysymelements;
|
|
|
|
$_ = $keysymelements[1];
|
|
die "Internal error, was expecting \"XC_*\", found: $_\n" if ( ! /^XK_/ );
|
|
|
|
$_ = $keysymelements[2];
|
|
die "Internal error, was expecting \"0x*\", found: $_\n" if ( ! /^0x/ );
|
|
|
|
my $element = $keysymelements[1];
|
|
my $binding = $element;
|
|
$binding =~ s/^XK_/CLUTTER_KEY_/g;
|
|
my $compat_binding = $element;
|
|
$compat_binding =~ s/^XK_/CLUTTER_/g;
|
|
|
|
printf OUT_KEYSYMS "#define %s 0x%03x\n", $binding, hex($keysymelements[2]);
|
|
printf OUT_KEYSYMS_COMPAT "#define %s 0x%03x\n", $compat_binding, hex($keysymelements[2]);
|
|
}
|
|
|
|
close IN_KEYSYMDEF;
|
|
|
|
#$cluttersyms{"0"} = "0000";
|
|
|
|
# Source: http://gitweb.freedesktop.org/?p=xorg/proto/x11proto.git;a=blob;f=XF86keysym.h
|
|
die "Could not open file XF86keysym.h: $!\n" unless open(IN_XF86KEYSYM, "<:utf8", "XF86keysym.h");
|
|
|
|
while (<IN_XF86KEYSYM>)
|
|
{
|
|
next if ( ! /^#define / );
|
|
|
|
@keysymelements = split(/\s+/);
|
|
die "Internal error, no \@keysymelements: $_\n" unless @keysymelements;
|
|
|
|
$_ = $keysymelements[1];
|
|
die "Internal error, was expecting \"XF86XK_*\", found: $_\n" if ( ! /^XF86XK_/ );
|
|
|
|
# Work-around https://bugs.freedesktop.org/show_bug.cgi?id=11193
|
|
if ($_ eq "XF86XK_XF86BackForward") {
|
|
$keysymelements[1] = "XF86XK_AudioForward";
|
|
}
|
|
# XF86XK_Clear could end up a dupe of XK_Clear
|
|
# XF86XK_Select could end up a dupe of XK_Select
|
|
if ($_ eq "XF86XK_Clear") {
|
|
$keysymelements[1] = "XF86XK_WindowClear";
|
|
}
|
|
if ($_ eq "XF86XK_Select") {
|
|
$keysymelements[1] = "XF86XK_SelectButton";
|
|
}
|
|
|
|
# Ignore XF86XK_Q
|
|
next if ( $_ eq "XF86XK_Q");
|
|
# XF86XK_Calculater is misspelled, and a dupe
|
|
next if ( $_ eq "XF86XK_Calculater");
|
|
|
|
$_ = $keysymelements[2];
|
|
die "Internal error, was expecting \"0x*\", found: $_\n" if ( ! /^0x/ );
|
|
|
|
my $element = $keysymelements[1];
|
|
my $binding = $element;
|
|
$binding =~ s/^XF86XK_/CLUTTER_KEY_/g;
|
|
my $compat_binding = $element;
|
|
$compat_binding =~ s/^XF86XK_/CLUTTER_/g;
|
|
|
|
printf OUT_KEYSYMS "#define %s 0x%03x\n", $binding, hex($keysymelements[2]);
|
|
printf OUT_KEYSYMS_COMPAT "#define %s 0x%03x\n", $compat_binding, hex($keysymelements[2]);
|
|
}
|
|
|
|
close IN_XF86KEYSYM;
|
|
|
|
|
|
print OUT_KEYSYMS<<EOF;
|
|
|
|
#endif /* __CLUTTER_KEYSYMS_H__ */
|
|
EOF
|
|
|
|
print OUT_KEYSYMS_COMPAT<<EOF;
|
|
|
|
#endif /* CLUTTER_DISABLE_DEPRECATED */
|
|
|
|
#endif /* __CLUTTER_KEYSYMS_DEPRECATED_H__ */
|
|
EOF
|
|
|
|
foreach my $f (qw/ keysymdef.h XF86keysym.h /) {
|
|
unlink $f or die "Unable to delete $f: $!";
|
|
}
|
|
|
|
printf "We just finished converting keysymdef.h to clutter-keysyms.h "
|
|
. "and deprecated/clutter-keysyms.h\nThank you\n";
|