mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
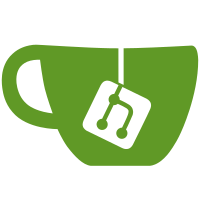
The CoglDebugFlags are now stored in an array of unsigned ints rather than a single variable. The flags are accessed using macros instead of directly peeking at the cogl_debug_flags variable. The index values are stored in the enum rather than the actual mask values so that the enum doesn't need to be more than 32 bits wide. The hope is that the code to determine the index into the array can be optimized out by the compiler so it should have exactly the same performance as the old code.
79 lines
1.8 KiB
C
79 lines
1.8 KiB
C
|
|
#ifdef COGL_ENABLE_PROFILE
|
|
|
|
#include "cogl-profile.h"
|
|
#include "cogl-debug.h"
|
|
|
|
#include <stdlib.h>
|
|
|
|
UProfContext *_cogl_uprof_context;
|
|
|
|
static gboolean
|
|
debug_option_getter (void *user_data)
|
|
{
|
|
unsigned int shift = GPOINTER_TO_UINT (user_data);
|
|
return COGL_DEBUG_ENABLED (shift);
|
|
}
|
|
|
|
static void
|
|
debug_option_setter (gboolean value, void *user_data)
|
|
{
|
|
unsigned int shift = GPOINTER_TO_UINT (user_data);
|
|
|
|
if (value)
|
|
COGL_DEBUG_SET_FLAG (shift);
|
|
else
|
|
COGL_DEBUG_CLEAR_FLAG (shift);
|
|
}
|
|
|
|
static void
|
|
print_exit_report (void)
|
|
{
|
|
if (getenv ("COGL_PROFILE_OUTPUT_REPORT"))
|
|
{
|
|
UProfReport *report = uprof_report_new ("Cogl report");
|
|
uprof_report_add_context (report, _cogl_uprof_context);
|
|
uprof_report_print (report);
|
|
uprof_report_unref (report);
|
|
}
|
|
uprof_context_unref (_cogl_uprof_context);
|
|
}
|
|
|
|
void
|
|
_cogl_uprof_init (void)
|
|
{
|
|
_cogl_uprof_context = uprof_context_new ("Cogl");
|
|
#define OPT(MASK_NAME, GROUP, NAME, NAME_FORMATTED, DESCRIPTION) \
|
|
G_STMT_START { \
|
|
int shift = COGL_DEBUG_ ## MASK_NAME; \
|
|
uprof_context_add_boolean_option (_cogl_uprof_context, \
|
|
GROUP, \
|
|
NAME, \
|
|
NAME_FORMATTED, \
|
|
DESCRIPTION, \
|
|
debug_option_getter, \
|
|
debug_option_setter, \
|
|
GUINT_TO_POINTER (shift)); \
|
|
} G_STMT_END;
|
|
|
|
#include "cogl-debug-options.h"
|
|
#undef OPT
|
|
|
|
g_atexit (print_exit_report);
|
|
}
|
|
|
|
void
|
|
_cogl_profile_trace_message (const char *format, ...)
|
|
{
|
|
va_list ap;
|
|
|
|
va_start (ap, format);
|
|
g_logv (G_LOG_DOMAIN, G_LOG_LEVEL_MESSAGE, format, ap);
|
|
va_end (ap);
|
|
|
|
if (_cogl_uprof_context)
|
|
uprof_context_vtrace_message (_cogl_uprof_context, format, ap);
|
|
}
|
|
|
|
#endif
|