mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
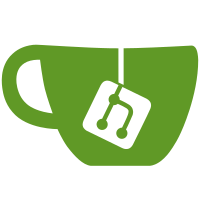
As part of the on going, incremental effort to purge the non type safe CoglHandle type from the Cogl API this patch tackles most of the CoglHandle uses relating to textures. We'd postponed making this change for quite a while because we wanted to have a clearer understanding of how we wanted to evolve the texture APIs towards Cogl 2.0 before exposing type safety here which would be difficult to change later since it would imply breaking APIs. The basic idea that we are steering towards now is that CoglTexture can be considered to be the most primitive interface we have for any object representing a texture. The texture interface would provide roughly these methods: cogl_texture_get_width cogl_texture_get_height cogl_texture_can_repeat cogl_texture_can_mipmap cogl_texture_generate_mipmap; cogl_texture_get_format cogl_texture_set_region cogl_texture_get_region Besides the texture interface we will then start to expose types corresponding to specific texture types: CoglTexture2D, CoglTexture3D, CoglTexture2DSliced, CoglSubTexture, CoglAtlasTexture and CoglTexturePixmapX11. We will then also expose an interface for the high-level texture types we have (such as CoglTexture2DSlice, CoglSubTexture and CoglAtlasTexture) called CoglMetaTexture. CoglMetaTexture is an additional interface that lets you iterate a virtual region of a meta texture and get mappings of primitive textures to sub-regions of that virtual region. Internally we already have this kind of abstraction for dealing with sliced texture, sub-textures and atlas textures in a consistent way, so this will just make that abstraction public. The aim here is to clarify that there is a difference between primitive textures (CoglTexture2D/3D) and some of the other high-level textures, and also enable developers to implement primitives that can support meta textures since they can only be used with the cogl_rectangle API currently. The thing that's not so clean-cut with this are the texture constructors we have currently; such as cogl_texture_new_from_file which no longer make sense when CoglTexture is considered to be an interface. These will basically just become convenient factory functions and it's just a bit unusual that they are within the cogl_texture namespace. It's worth noting here that all the texture type APIs will also have their own type specific constructors so these functions will only be used for the convenience of being able to create a texture without really wanting to know the details of what type of texture you need. Longer term for 2.0 we may come up with replacement names for these factory functions or the other thing we are considering is designing some asynchronous factory functions instead since it's so often detrimental to application performance to be blocked waiting for a texture to be uploaded to the GPU. Reviewed-by: Neil Roberts <neil@linux.intel.com>
106 lines
3.4 KiB
C
106 lines
3.4 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_JOURNAL_PRIVATE_H
|
|
#define __COGL_JOURNAL_PRIVATE_H
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-handle.h"
|
|
#include "cogl-clip-stack.h"
|
|
|
|
#define COGL_JOURNAL_VBO_POOL_SIZE 8
|
|
|
|
typedef struct _CoglJournal
|
|
{
|
|
CoglObject _parent;
|
|
|
|
GArray *entries;
|
|
GArray *vertices;
|
|
size_t needed_vbo_len;
|
|
|
|
/* A pool of attribute buffers is used so that we can avoid repeatedly
|
|
reallocating buffers. Only one of these buffers at a time will be
|
|
used by Cogl but we keep more than one alive anyway in case the
|
|
GL driver is internally using the buffer and it would have to
|
|
allocate a new one when we start writing to it */
|
|
CoglAttributeBuffer *vbo_pool[COGL_JOURNAL_VBO_POOL_SIZE];
|
|
/* The next vbo to use from the pool. We just cycle through them in
|
|
order */
|
|
unsigned int next_vbo_in_pool;
|
|
|
|
int fast_read_pixel_count;
|
|
|
|
} CoglJournal;
|
|
|
|
/* To improve batching of geometry when submitting vertices to OpenGL we
|
|
* log the texture rectangles we want to draw to a journal, so when we
|
|
* later flush the journal we aim to batch data, and gl draw calls. */
|
|
typedef struct _CoglJournalEntry
|
|
{
|
|
CoglPipeline *pipeline;
|
|
int n_layers;
|
|
CoglMatrix model_view;
|
|
CoglClipStack *clip_stack;
|
|
/* Offset into ctx->logged_vertices */
|
|
size_t array_offset;
|
|
/* XXX: These entries are pretty big now considering the padding in
|
|
* CoglPipelineFlushOptions and CoglMatrix, so we might need to optimize this
|
|
* later. */
|
|
} CoglJournalEntry;
|
|
|
|
CoglJournal *
|
|
_cogl_journal_new (void);
|
|
|
|
void
|
|
_cogl_journal_log_quad (CoglJournal *journal,
|
|
const float *position,
|
|
CoglPipeline *pipeline,
|
|
int n_layers,
|
|
CoglTexture *layer0_override_texture,
|
|
const float *tex_coords,
|
|
unsigned int tex_coords_len);
|
|
|
|
void
|
|
_cogl_journal_flush (CoglJournal *journal,
|
|
CoglFramebuffer *framebuffer);
|
|
|
|
void
|
|
_cogl_journal_discard (CoglJournal *journal);
|
|
|
|
gboolean
|
|
_cogl_journal_all_entries_within_bounds (CoglJournal *journal,
|
|
float clip_x0,
|
|
float clip_y0,
|
|
float clip_x1,
|
|
float clip_y1);
|
|
|
|
gboolean
|
|
_cogl_journal_try_read_pixel (CoglJournal *journal,
|
|
int x,
|
|
int y,
|
|
CoglPixelFormat format,
|
|
guint8 *pixel,
|
|
gboolean *found_intersection);
|
|
|
|
#endif /* __COGL_JOURNAL_PRIVATE_H */
|