mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
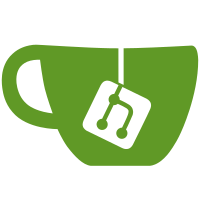
CoglHandle is a common source of complaints and confusion because people expect a "handle" to be some form of integer type with some indirection to lookup the corresponding objects as opposed to a direct pointer. This patch starts by renaming CoglHandle to CoglObject * and creating corresponding cogl_object_ APIs to replace the cogl_handle ones. The next step though is to remove all use of CoglHandle in the Cogl APIs and replace with strongly typed pointer types such as CoglMaterial * or CoglTexture * etc also all occurrences of COGL_INVALID_HANDLE can just use NULL instead. After this we will consider switching to GTypeInstance internally so we can have inheritance for our types and hopefully improve how we handle bindings. Note all these changes will be done in a way that maintains the API and ABI.
272 lines
6.3 KiB
C
272 lines
6.3 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009,2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include <glib-object.h>
|
|
#include <gobject/gvaluecollector.h>
|
|
|
|
#include "cogl.h"
|
|
|
|
#include "cogl-fixed.h"
|
|
#include "cogl-internal.h"
|
|
#include "cogl-material.h"
|
|
#include "cogl-offscreen.h"
|
|
#include "cogl-shader.h"
|
|
#include "cogl-texture.h"
|
|
#include "cogl-types.h"
|
|
#include "cogl-handle.h"
|
|
#include "cogl-util.h"
|
|
|
|
/**
|
|
* cogl_util_next_p2:
|
|
* @a: Value to get the next power
|
|
*
|
|
* Calculates the next power greater than @a.
|
|
*
|
|
* Return value: The next power after @a.
|
|
*/
|
|
int
|
|
cogl_util_next_p2 (int a)
|
|
{
|
|
int rval = 1;
|
|
|
|
while (rval < a)
|
|
rval <<= 1;
|
|
|
|
return rval;
|
|
}
|
|
|
|
/* gtypes */
|
|
|
|
void *
|
|
cogl_object_ref (void *object)
|
|
{
|
|
CoglObject *obj = object;
|
|
|
|
g_return_val_if_fail (object != NULL, NULL);
|
|
|
|
obj->ref_count++;
|
|
return object;
|
|
}
|
|
|
|
CoglHandle
|
|
cogl_handle_ref (CoglHandle handle)
|
|
{
|
|
return cogl_object_ref (handle);
|
|
}
|
|
|
|
void
|
|
cogl_object_unref (void *object)
|
|
{
|
|
CoglObject *obj = object;
|
|
|
|
g_return_if_fail (object != NULL);
|
|
g_return_if_fail (obj->ref_count > 0);
|
|
|
|
if (--obj->ref_count < 1)
|
|
{
|
|
void (*free_func)(void *obj);
|
|
|
|
COGL_OBJECT_DEBUG_FREE (obj);
|
|
free_func = obj->klass->virt_free;
|
|
free_func (obj);
|
|
}
|
|
}
|
|
|
|
void
|
|
cogl_handle_unref (CoglHandle handle)
|
|
{
|
|
cogl_object_unref (handle);
|
|
}
|
|
|
|
GType
|
|
cogl_object_get_type (void)
|
|
{
|
|
static GType our_type = 0;
|
|
|
|
/* XXX: We are keeping the "CoglHandle" name for now incase it would
|
|
* break bindings to change to "CoglObject" */
|
|
if (G_UNLIKELY (our_type == 0))
|
|
our_type = g_boxed_type_register_static (g_intern_static_string ("CoglHandle"),
|
|
(GBoxedCopyFunc) cogl_object_ref,
|
|
(GBoxedFreeFunc) cogl_object_unref);
|
|
|
|
return our_type;
|
|
}
|
|
|
|
GType
|
|
cogl_handle_get_type (void)
|
|
{
|
|
return cogl_object_get_type ();
|
|
}
|
|
/*
|
|
* CoglFixed
|
|
*/
|
|
|
|
static GTypeInfo _info = {
|
|
0,
|
|
NULL,
|
|
NULL,
|
|
NULL,
|
|
NULL,
|
|
NULL,
|
|
0,
|
|
0,
|
|
NULL,
|
|
NULL,
|
|
};
|
|
|
|
static GTypeFundamentalInfo _finfo = { 0, };
|
|
|
|
static void
|
|
cogl_value_init_fixed (GValue *value)
|
|
{
|
|
value->data[0].v_int = 0;
|
|
}
|
|
|
|
static void
|
|
cogl_value_copy_fixed (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_int = src->data[0].v_int;
|
|
}
|
|
|
|
static char *
|
|
cogl_value_collect_fixed (GValue *value,
|
|
unsigned int n_collect_values,
|
|
GTypeCValue *collect_values,
|
|
unsigned int collect_flags)
|
|
{
|
|
value->data[0].v_int = collect_values[0].v_int;
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static char *
|
|
cogl_value_lcopy_fixed (const GValue *value,
|
|
unsigned int n_collect_values,
|
|
GTypeCValue *collect_values,
|
|
unsigned int collect_flags)
|
|
{
|
|
gint32 *fixed_p = collect_values[0].v_pointer;
|
|
|
|
if (!fixed_p)
|
|
return g_strdup_printf ("value location for '%s' passed as NULL",
|
|
G_VALUE_TYPE_NAME (value));
|
|
|
|
*fixed_p = value->data[0].v_int;
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_fixed_int (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_int = COGL_FIXED_TO_INT (src->data[0].v_int);
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_fixed_double (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_double = COGL_FIXED_TO_DOUBLE (src->data[0].v_int);
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_fixed_float (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_float = COGL_FIXED_TO_FLOAT (src->data[0].v_int);
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_int_fixed (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_int = COGL_FIXED_FROM_INT (src->data[0].v_int);
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_double_fixed (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_int = COGL_FIXED_FROM_DOUBLE (src->data[0].v_double);
|
|
}
|
|
|
|
static void
|
|
cogl_value_transform_float_fixed (const GValue *src,
|
|
GValue *dest)
|
|
{
|
|
dest->data[0].v_int = COGL_FIXED_FROM_FLOAT (src->data[0].v_float);
|
|
}
|
|
|
|
|
|
static const GTypeValueTable _cogl_fixed_value_table = {
|
|
cogl_value_init_fixed,
|
|
NULL,
|
|
cogl_value_copy_fixed,
|
|
NULL,
|
|
"i",
|
|
cogl_value_collect_fixed,
|
|
"p",
|
|
cogl_value_lcopy_fixed
|
|
};
|
|
|
|
GType
|
|
cogl_fixed_get_type (void)
|
|
{
|
|
static GType _cogl_fixed_type = 0;
|
|
|
|
if (G_UNLIKELY (_cogl_fixed_type == 0))
|
|
{
|
|
_info.value_table = & _cogl_fixed_value_table;
|
|
_cogl_fixed_type =
|
|
g_type_register_fundamental (g_type_fundamental_next (),
|
|
g_intern_static_string ("CoglFixed"),
|
|
&_info, &_finfo, 0);
|
|
|
|
g_value_register_transform_func (_cogl_fixed_type, G_TYPE_INT,
|
|
cogl_value_transform_fixed_int);
|
|
g_value_register_transform_func (G_TYPE_INT, _cogl_fixed_type,
|
|
cogl_value_transform_int_fixed);
|
|
|
|
g_value_register_transform_func (_cogl_fixed_type, G_TYPE_FLOAT,
|
|
cogl_value_transform_fixed_float);
|
|
g_value_register_transform_func (G_TYPE_FLOAT, _cogl_fixed_type,
|
|
cogl_value_transform_float_fixed);
|
|
|
|
g_value_register_transform_func (_cogl_fixed_type, G_TYPE_DOUBLE,
|
|
cogl_value_transform_fixed_double);
|
|
g_value_register_transform_func (G_TYPE_DOUBLE, _cogl_fixed_type,
|
|
cogl_value_transform_double_fixed);
|
|
}
|
|
|
|
return _cogl_fixed_type;
|
|
}
|
|
|
|
|