mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
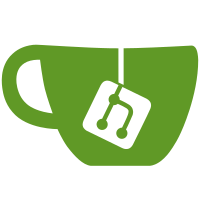
Previously a path copy was implemented such that only the array of path nodes was shared with the source and the rest of the data is copied. This was so that the copy could avoid a deep copy if the source path is appended to because the copy keeps track of its own length. This optimisation is probably not worthwhile because it makes the copies less cheap. Instead the CoglPath struct now just contains a single pointer to a new CoglPathData struct which is separately ref-counted. When the path is modified it will be copied if the ref count on the data is not 1.
93 lines
2.0 KiB
C
93 lines
2.0 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_PATH_PRIVATE_H
|
|
#define __COGL_PATH_PRIVATE_H
|
|
|
|
#include "cogl-handle.h"
|
|
|
|
#define COGL_PATH(tex) ((CoglPath *)(tex))
|
|
|
|
typedef struct _floatVec2
|
|
{
|
|
float x;
|
|
float y;
|
|
} floatVec2;
|
|
|
|
typedef struct _CoglPathNode
|
|
{
|
|
float x;
|
|
float y;
|
|
unsigned int path_size;
|
|
} CoglPathNode;
|
|
|
|
typedef struct _CoglBezQuad
|
|
{
|
|
floatVec2 p1;
|
|
floatVec2 p2;
|
|
floatVec2 p3;
|
|
} CoglBezQuad;
|
|
|
|
typedef struct _CoglBezCubic
|
|
{
|
|
floatVec2 p1;
|
|
floatVec2 p2;
|
|
floatVec2 p3;
|
|
floatVec2 p4;
|
|
} CoglBezCubic;
|
|
|
|
typedef struct _CoglPath CoglPath;
|
|
typedef struct _CoglPathData CoglPathData;
|
|
|
|
struct _CoglPath
|
|
{
|
|
CoglHandleObject _parent;
|
|
|
|
CoglPathData *data;
|
|
};
|
|
|
|
struct _CoglPathData
|
|
{
|
|
unsigned int ref_count;
|
|
|
|
GArray *path_nodes;
|
|
|
|
floatVec2 path_start;
|
|
floatVec2 path_pen;
|
|
unsigned int last_path;
|
|
floatVec2 path_nodes_min;
|
|
floatVec2 path_nodes_max;
|
|
};
|
|
|
|
/* This is an internal version of cogl_path_new that doesn't affect
|
|
the current path and just creates a new handle */
|
|
CoglHandle
|
|
_cogl_path_new (void);
|
|
|
|
void
|
|
_cogl_add_path_to_stencil_buffer (CoglHandle path,
|
|
gboolean merge,
|
|
gboolean need_clear);
|
|
|
|
#endif /* __COGL_PATH_PRIVATE_H */
|