mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
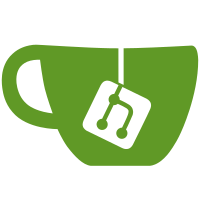
This adds a wrapper macro to clutter-private that will use g_object_notify_by_pspec if it's compiled against a version of GLib that is sufficiently new. Otherwise it will notify by the property name as before by extracting the name from the pspec. The objects can then store a static array of GParamSpecs and notify using those as suggested in the documentation for g_object_notify_by_pspec. Note that the name of the variable used for storing the array of GParamSpecs is obj_props instead of properties as used in the documentation because some places in Clutter uses 'properties' as the name of a local variable. Mose of the classes in Clutter have been converted using the script in the bug report. Some classes have not been modified even though the script picked them up as described here: json-generator: We probably don't want to modify the internal copy of JSON behaviour-depth: rectangle: score: stage-manager: These aren't using the separate GParamSpec* variable style. blur-effect: win32/device-manager: Don't actually define any properties even though it has the enum. box-layout: flow-layout: Have some per-child properties that don't work automatically with the script. clutter-model: The script gets confused with ClutterModelIter stage: Script gets confused because PROP_USER_RESIZE doesn't match "user-resizable" test-layout: Don't really want to modify the tests http://bugzilla.clutter-project.org/show_bug.cgi?id=2150
148 lines
3.9 KiB
C
148 lines
3.9 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Copyright (C) 2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* Author:
|
|
* Emmanuele Bassi <ebassi@linux.intel.com>
|
|
*/
|
|
|
|
/**
|
|
* SECTION:clutter-layout-meta
|
|
* @short_description: Wrapper for actors inside a layout manager
|
|
*
|
|
* #ClutterLayoutMeta is a wrapper object created by #ClutterLayoutManager
|
|
* implementations in order to store child-specific data and properties.
|
|
*
|
|
* A #ClutterLayoutMeta wraps a #ClutterActor inside a #ClutterContainer
|
|
* using a #ClutterLayoutManager.
|
|
*
|
|
* #ClutterLayoutMeta is available since Clutter 1.2
|
|
*/
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "clutter-layout-meta.h"
|
|
#include "clutter-debug.h"
|
|
#include "clutter-private.h"
|
|
|
|
G_DEFINE_ABSTRACT_TYPE (ClutterLayoutMeta,
|
|
clutter_layout_meta,
|
|
CLUTTER_TYPE_CHILD_META);
|
|
|
|
enum
|
|
{
|
|
PROP_0,
|
|
|
|
PROP_MANAGER,
|
|
|
|
PROP_LAST
|
|
};
|
|
|
|
static GParamSpec *obj_props[PROP_LAST];
|
|
|
|
static void
|
|
clutter_layout_meta_set_property (GObject *object,
|
|
guint prop_id,
|
|
const GValue *value,
|
|
GParamSpec *pspec)
|
|
{
|
|
ClutterLayoutMeta *layout_meta = CLUTTER_LAYOUT_META (object);
|
|
|
|
switch (prop_id)
|
|
{
|
|
case PROP_MANAGER:
|
|
layout_meta->manager = g_value_get_object (value);
|
|
break;
|
|
|
|
default:
|
|
G_OBJECT_WARN_INVALID_PROPERTY_ID (object, prop_id, pspec);
|
|
break;
|
|
}
|
|
}
|
|
|
|
static void
|
|
clutter_layout_meta_get_property (GObject *object,
|
|
guint prop_id,
|
|
GValue *value,
|
|
GParamSpec *pspec)
|
|
{
|
|
ClutterLayoutMeta *layout_meta = CLUTTER_LAYOUT_META (object);
|
|
|
|
switch (prop_id)
|
|
{
|
|
case PROP_MANAGER:
|
|
g_value_set_object (value, layout_meta->manager);
|
|
break;
|
|
|
|
default:
|
|
G_OBJECT_WARN_INVALID_PROPERTY_ID (object, prop_id, pspec);
|
|
break;
|
|
}
|
|
}
|
|
|
|
static void
|
|
clutter_layout_meta_class_init (ClutterLayoutMetaClass *klass)
|
|
{
|
|
GObjectClass *gobject_class = G_OBJECT_CLASS (klass);
|
|
GParamSpec *pspec;
|
|
|
|
gobject_class->set_property = clutter_layout_meta_set_property;
|
|
gobject_class->get_property = clutter_layout_meta_get_property;
|
|
|
|
/**
|
|
* ClutterLayoutMeta:manager:
|
|
*
|
|
* The #ClutterLayoutManager that created this #ClutterLayoutMeta.
|
|
*
|
|
* Since: 1.2
|
|
*/
|
|
pspec = g_param_spec_object ("manager",
|
|
P_("Manager"),
|
|
P_("The manager that created this data"),
|
|
CLUTTER_TYPE_LAYOUT_MANAGER,
|
|
G_PARAM_CONSTRUCT_ONLY |
|
|
CLUTTER_PARAM_READWRITE);
|
|
obj_props[PROP_MANAGER] = pspec;
|
|
g_object_class_install_property (gobject_class, PROP_MANAGER, pspec);
|
|
}
|
|
|
|
static void
|
|
clutter_layout_meta_init (ClutterLayoutMeta *self)
|
|
{
|
|
}
|
|
|
|
/**
|
|
* clutter_layout_meta_get_manager:
|
|
* @data: a #ClutterLayoutMeta
|
|
*
|
|
* Retrieves the actor wrapped by @data
|
|
*
|
|
* Return value: (transfer none): a #ClutterLayoutManager
|
|
*
|
|
* Since: 1.2
|
|
*/
|
|
ClutterLayoutManager *
|
|
clutter_layout_meta_get_manager (ClutterLayoutMeta *data)
|
|
{
|
|
g_return_val_if_fail (CLUTTER_IS_LAYOUT_META (data), NULL);
|
|
|
|
return data->manager;
|
|
}
|