mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
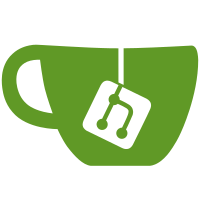
My previous work to provide muti-texturing support has been extended into a CoglMaterial abstraction that adds control over the texture combine functions (controlling how multiple texture layers are blended together), the gl blend function (used for blending the final primitive with the framebuffer), the alpha function (used to discard fragments based on their alpha channel), describing attributes such as a diffuse, ambient and specular color (for use with the standard OpenGL lighting model), and per layer rotations. (utilizing the new CoglMatrix utility API) For now the only way this abstraction is exposed is via a new cogl_material_rectangle function, that is similar to cogl_texture_rectangle but doesn't take a texture handle (the source material is pulled from the context), and the array of texture coordinates is extended to be able to supply coordinates for each layer. Note: this function doesn't support sliced textures; supporting sliced textures is a non trivial problem, considering the ability to rotate layers. Note: cogl_material_rectangle, has quite a few workarounds, for a number of other limitations within Cogl a.t.m. Note: The GLES1/2 multi-texturing support has yet to be updated to use the material abstraction.
87 lines
2.4 KiB
C
87 lines
2.4 KiB
C
/*
|
|
* Clutter COGL
|
|
*
|
|
* A basic GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2007 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_TEXTURE_H
|
|
#define __COGL_TEXTURE_H
|
|
|
|
#include "cogl-bitmap.h"
|
|
|
|
typedef struct _CoglTexture CoglTexture;
|
|
typedef struct _CoglTexSliceSpan CoglTexSliceSpan;
|
|
typedef struct _CoglSpanIter CoglSpanIter;
|
|
typedef struct _CoglCompositeTexture CoglCompositeTexture;
|
|
typedef struct _CoglCompositeTextureLayer CoglCompositeTextureLayer;
|
|
|
|
struct _CoglTexSliceSpan
|
|
{
|
|
gint start;
|
|
gint size;
|
|
gint waste;
|
|
};
|
|
|
|
struct _CoglTexture
|
|
{
|
|
guint ref_count;
|
|
CoglBitmap bitmap;
|
|
gboolean bitmap_owner;
|
|
GLenum gl_target;
|
|
GLenum gl_intformat;
|
|
GLenum gl_format;
|
|
GLenum gl_type;
|
|
GArray *slice_x_spans;
|
|
GArray *slice_y_spans;
|
|
GArray *slice_gl_handles;
|
|
gint max_waste;
|
|
COGLenum min_filter;
|
|
COGLenum mag_filter;
|
|
gboolean is_foreign;
|
|
GLint wrap_mode;
|
|
gboolean auto_mipmap;
|
|
};
|
|
|
|
struct _CoglCompositeTextureLayer
|
|
{
|
|
guint ref_count;
|
|
|
|
guint index; /*!< lowest index is blended first then others
|
|
on top */
|
|
CoglTexture *tex; /*!< The texture for this layer, or NULL
|
|
for an empty layer */
|
|
|
|
/* TODO: Add more control over the texture environment for each texture
|
|
* unit. For example we should support dot3 normal mapping. */
|
|
};
|
|
|
|
struct _CoglCompositeTexture
|
|
{
|
|
guint ref_count;
|
|
GList *layers;
|
|
};
|
|
|
|
CoglTexture*
|
|
_cogl_texture_pointer_from_handle (CoglHandle handle);
|
|
|
|
#endif /* __COGL_TEXTURE_H */
|