mirror of
https://github.com/brl/mutter.git
synced 2025-07-08 03:38:13 +00:00
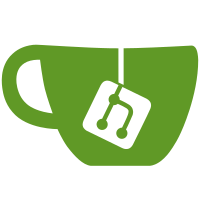
My previous work to provide muti-texturing support has been extended into a CoglMaterial abstraction that adds control over the texture combine functions (controlling how multiple texture layers are blended together), the gl blend function (used for blending the final primitive with the framebuffer), the alpha function (used to discard fragments based on their alpha channel), describing attributes such as a diffuse, ambient and specular color (for use with the standard OpenGL lighting model), and per layer rotations. (utilizing the new CoglMatrix utility API) For now the only way this abstraction is exposed is via a new cogl_material_rectangle function, that is similar to cogl_texture_rectangle but doesn't take a texture handle (the source material is pulled from the context), and the array of texture coordinates is extended to be able to supply coordinates for each layer. Note: this function doesn't support sliced textures; supporting sliced textures is a non trivial problem, considering the ability to rotate layers. Note: cogl_material_rectangle, has quite a few workarounds, for a number of other limitations within Cogl a.t.m. Note: The GLES1/2 multi-texturing support has yet to be updated to use the material abstraction.
73 lines
1.9 KiB
C
73 lines
1.9 KiB
C
#ifndef __COGL_MATERIAL_PRIVATE_H
|
|
#define __COGL_MATERIAL_PRIVATE_H
|
|
|
|
#include "cogl-material.h"
|
|
#include "cogl-matrix.h"
|
|
|
|
#include <glib.h>
|
|
|
|
typedef struct _CoglMaterial CoglMaterial;
|
|
typedef struct _CoglMaterialLayer CoglMaterialLayer;
|
|
|
|
typedef enum _CoglMaterialLayerFlags
|
|
{
|
|
COGL_MATERIAL_LAYER_FLAG_USER_MATRIX = 1L<<0,
|
|
} CoglMaterialLayerFlags;
|
|
|
|
struct _CoglMaterialLayer
|
|
{
|
|
guint ref_count;
|
|
guint index; /*!< lowest index is blended first then others
|
|
on top */
|
|
gulong flags;
|
|
CoglHandle texture; /*!< The texture for this layer, or COGL_INVALID_HANDLE
|
|
for an empty layer */
|
|
|
|
/* Determines how the color of individual texture fragments
|
|
* are calculated. */
|
|
CoglMaterialLayerCombineFunc texture_combine_rgb_func;
|
|
CoglMaterialLayerCombineSrc texture_combine_rgb_src[3];
|
|
CoglMaterialLayerCombineOp texture_combine_rgb_op[3];
|
|
|
|
CoglMaterialLayerCombineFunc texture_combine_alpha_func;
|
|
CoglMaterialLayerCombineSrc texture_combine_alpha_src[3];
|
|
CoglMaterialLayerCombineOp texture_combine_alpha_op[3];
|
|
|
|
/* TODO: Support purely GLSL based material layers */
|
|
|
|
CoglMatrix matrix;
|
|
};
|
|
|
|
typedef enum _CoglMaterialFlags
|
|
{
|
|
COGL_MATERIAL_FLAG_ENABLE_BLEND = 1L<<0,
|
|
COGL_MATERIAL_FLAG_SHOWN_SAMPLER_WARNING = 1L<<1
|
|
} CoglMaterialFlags;
|
|
|
|
struct _CoglMaterial
|
|
{
|
|
guint ref_count;
|
|
|
|
gulong flags;
|
|
|
|
/* Standard OpenGL lighting model attributes */
|
|
GLfloat ambient[4];
|
|
GLfloat diffuse[4];
|
|
GLfloat specular[4];
|
|
GLfloat emission[4];
|
|
GLfloat shininess;
|
|
|
|
/* Determines what fragments are discarded based on their alpha */
|
|
CoglMaterialAlphaFunc alpha_func;
|
|
GLfloat alpha_func_reference;
|
|
|
|
/* Determines how this material is blended with other primitives */
|
|
CoglMaterialBlendFactor blend_src_factor;
|
|
CoglMaterialBlendFactor blend_dst_factor;
|
|
|
|
GList *layers;
|
|
};
|
|
|
|
#endif /* __COGL_MATERIAL_PRIVATE_H */
|
|
|