mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 22:46:02 -04:00
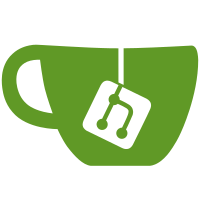
This is a workaround for a race condition when resizing windows while there are in-flight glXCopySubBuffer blits happening. The problem stems from the fact that rectangles for the blits are described relative to the bottom left of the window and because we can't guarantee control over the X window gravity used when resizing so the gravity is typically NorthWest not SouthWest. This means if you grow a window vertically the server will make sure to place the old contents of the window at the top-left/north-west of your new larger window, but that may happen asynchronous to GLX preparing to do a blit specified relative to the bottom-left/south-west of the window (based on the old smaller window geometry). When the GLX issued blit finally happens relative to the new bottom of your window, the destination will have shifted relative to the top-left where all the pixels you care about are so it will result in a nasty artefact making resizing look very ugly! We can't currently fix this completely, in-part because the window manager tends to trample any gravity we might set. This workaround instead simply disables blits for a while if we are notified of any resizes happening so if the user is resizing a window via the window manager then they may see an artefact for one frame but then we will fallback to redrawing the full stage until the cooling off period is over.
95 lines
3.1 KiB
C
95 lines
3.1 KiB
C
/* Clutter.
|
|
* An OpenGL based 'interactive canvas' library.
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
* Copyright (C) 2006-2007 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __CLUTTER_STAGE_X11_H__
|
|
#define __CLUTTER_STAGE_X11_H__
|
|
|
|
#include <clutter/clutter-group.h>
|
|
#include <clutter/clutter-stage.h>
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xatom.h>
|
|
|
|
#include "clutter-backend-x11.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_STAGE_X11 (clutter_stage_x11_get_type ())
|
|
#define CLUTTER_STAGE_X11(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), CLUTTER_TYPE_STAGE_X11, ClutterStageX11))
|
|
#define CLUTTER_IS_STAGE_X11(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), CLUTTER_TYPE_STAGE_X11))
|
|
#define CLUTTER_STAGE_X11_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), CLUTTER_TYPE_STAGE_X11, ClutterStageX11Class))
|
|
#define CLUTTER_IS_STAGE_X11_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), CLUTTER_TYPE_STAGE_X11))
|
|
#define CLUTTER_STAGE_X11_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), CLUTTER_TYPE_STAGE_X11, ClutterStageX11Class))
|
|
|
|
typedef struct _ClutterStageX11 ClutterStageX11;
|
|
typedef struct _ClutterStageX11Class ClutterStageX11Class;
|
|
|
|
typedef enum
|
|
{
|
|
STAGE_X11_WITHDRAWN = 1 << 1
|
|
} ClutterStageX11State;
|
|
|
|
struct _ClutterStageX11
|
|
{
|
|
ClutterGroup parent_instance;
|
|
|
|
guint is_foreign_xwin : 1;
|
|
guint fullscreening : 1;
|
|
guint is_cursor_visible : 1;
|
|
guint viewport_initialized : 1;
|
|
|
|
Window xwin;
|
|
gint xwin_width;
|
|
gint xwin_height; /* FIXME target_width / height */
|
|
gchar *title;
|
|
|
|
guint clipped_redraws_cool_off;
|
|
|
|
ClutterStageState state;
|
|
|
|
ClutterStageX11State wm_state;
|
|
|
|
int event_types[CLUTTER_X11_XINPUT_LAST_EVENT];
|
|
GList *devices;
|
|
|
|
ClutterStage *wrapper;
|
|
};
|
|
|
|
struct _ClutterStageX11Class
|
|
{
|
|
ClutterGroupClass parent_class;
|
|
};
|
|
|
|
GType clutter_stage_x11_get_type (void) G_GNUC_CONST;
|
|
|
|
/* Private to subclasses */
|
|
void clutter_stage_x11_fix_window_size (ClutterStageX11 *stage_x11,
|
|
gint new_width,
|
|
gint new_height);
|
|
void clutter_stage_x11_set_wm_protocols (ClutterStageX11 *stage_x11);
|
|
void clutter_stage_x11_map (ClutterStageX11 *stage_x11);
|
|
void clutter_stage_x11_unmap (ClutterStageX11 *stage_x11);
|
|
|
|
GList *clutter_stage_x11_get_input_devices (ClutterStageX11 *stage_x11);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __CLUTTER_STAGE_H__ */
|