mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
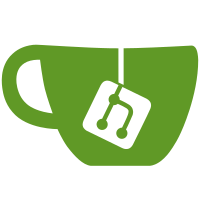
Adds missing notices, and ensures all the notices are consistent. The Cogl blurb also now reads: * Cogl * * An object oriented GL/GLES Abstraction/Utility Layer
132 lines
3.4 KiB
C
132 lines
3.4 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-shader-private.h"
|
|
#include "cogl-internal.h"
|
|
#include "cogl-context.h"
|
|
#include "cogl-handle.h"
|
|
|
|
#include <glib.h>
|
|
|
|
/* Expecting ARB functions not to be defined */
|
|
#define glCreateShaderObjectARB ctx->pf_glCreateShaderObjectARB
|
|
#define glGetObjectParameterivARB ctx->pf_glGetObjectParameterivARB
|
|
#define glGetInfoLogARB ctx->pf_glGetInfoLogARB
|
|
#define glCompileShaderARB ctx->pf_glCompileShaderARB
|
|
#define glShaderSourceARB ctx->pf_glShaderSourceARB
|
|
#define glDeleteObjectARB ctx->pf_glDeleteObjectARB
|
|
|
|
static void _cogl_shader_free (CoglShader *shader);
|
|
|
|
COGL_HANDLE_DEFINE (Shader, shader);
|
|
|
|
static void
|
|
_cogl_shader_free (CoglShader *shader)
|
|
{
|
|
/* Frees shader resources but its handle is not
|
|
released! Do that separately before this! */
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
glDeleteObjectARB (shader->gl_handle);
|
|
}
|
|
|
|
CoglHandle
|
|
cogl_create_shader (COGLenum shaderType)
|
|
{
|
|
CoglShader *shader;
|
|
|
|
_COGL_GET_CONTEXT (ctx, 0);
|
|
|
|
shader = g_slice_new (CoglShader);
|
|
shader->gl_handle = glCreateShaderObjectARB (shaderType);
|
|
|
|
return _cogl_shader_handle_new (shader);
|
|
}
|
|
|
|
void
|
|
cogl_shader_source (CoglHandle handle,
|
|
const gchar *source)
|
|
{
|
|
CoglShader *shader;
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
|
|
if (!cogl_is_shader (handle))
|
|
return;
|
|
|
|
shader = _cogl_shader_pointer_from_handle (handle);
|
|
|
|
glShaderSourceARB (shader->gl_handle, 1, &source, NULL);
|
|
}
|
|
|
|
void
|
|
cogl_shader_compile (CoglHandle handle)
|
|
{
|
|
CoglShader *shader;
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
|
|
if (!cogl_is_shader (handle))
|
|
return;
|
|
|
|
shader = _cogl_shader_pointer_from_handle (handle);
|
|
|
|
glCompileShaderARB (shader->gl_handle);
|
|
}
|
|
|
|
void
|
|
cogl_shader_get_info_log (CoglHandle handle,
|
|
guint size,
|
|
gchar *buffer)
|
|
{
|
|
CoglShader *shader;
|
|
COGLint len;
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
|
|
if (!cogl_is_shader (handle))
|
|
return;
|
|
|
|
shader = _cogl_shader_pointer_from_handle (handle);
|
|
|
|
glGetInfoLogARB (shader->gl_handle, size-1, &len, buffer);
|
|
buffer[len]='\0';
|
|
}
|
|
|
|
void
|
|
cogl_shader_get_parameteriv (CoglHandle handle,
|
|
COGLenum pname,
|
|
COGLint *dest)
|
|
{
|
|
CoglShader *shader;
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
|
|
if (!cogl_is_shader (handle))
|
|
return;
|
|
|
|
shader = _cogl_shader_pointer_from_handle (handle);
|
|
|
|
glGetObjectParameterivARB (shader->gl_handle, pname, dest);
|
|
}
|