mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
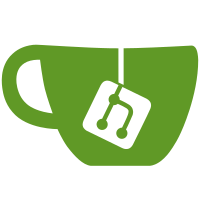
The CoglPipelineCache is now extended to store templates for state affecting vertex shaders and combined programs. The GLSL fragend, vertend and progend now uses this to get cached shaders and a program. When a new pipeline is created it will now get hashed three times if the GLSL backends are in use (once for the fragend, once for the vertend and once for the progend). Ideally we should add some way for the progend to check its cache before the fragends and vertends are checked so that it can bypass them entirely if it can find a cached combined program.
78 lines
2.7 KiB
C
78 lines
2.7 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2011 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_PIPELINE_CACHE_H__
|
|
#define __COGL_PIPELINE_CACHE_H__
|
|
|
|
#include "cogl-pipeline.h"
|
|
|
|
typedef struct _CoglPipelineCache CoglPipelineCache;
|
|
|
|
CoglPipelineCache *
|
|
cogl_pipeline_cache_new (void);
|
|
|
|
void
|
|
cogl_pipeline_cache_free (CoglPipelineCache *cache);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_FRAGMENT_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipeline *
|
|
_cogl_pipeline_cache_get_fragment_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_VERTEX_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipeline *
|
|
_cogl_pipeline_cache_get_vertex_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the combination of the state state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_VERTEX_CODEGEN and
|
|
* COGL_PIPELINE_STATE_AFFECTS_FRAGMENT_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipeline *
|
|
_cogl_pipeline_cache_get_combined_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
#endif /* __COGL_PIPELINE_CACHE_H__ */
|