mirror of
https://github.com/brl/mutter.git
synced 2024-09-21 06:56:12 -04:00
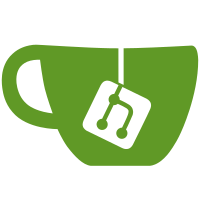
Instead of using our own homegrown alpha functions, we should use the easing functions also shared by other animation frameworks, like jQuery and Tween, in the interests of code portability. The easing functions have been defined by Robert Penner and are divided into three categories: In Out InOut Each category has a particular curve: Quadratic Cubic Quartic Quintic Sinusoidal Exponential Circular In addition, there are "physical" curves: Elastic Back (overshooting cubic) Bounce (exponentially decaying parabolic) Finally, the Linear curve is also provided as a reference. The functions are private, and are meant to be used only through their logical id as provided by the AnimationMode enumeration. The tests should be updated as well to match the new easing functions.
115 lines
3.2 KiB
C
115 lines
3.2 KiB
C
#include <stdlib.h>
|
|
#include <gmodule.h>
|
|
#include <clutter/clutter.h>
|
|
|
|
static gboolean is_expanded = FALSE;
|
|
|
|
static void
|
|
on_animation_complete (ClutterAnimation *animation,
|
|
ClutterActor *actor)
|
|
{
|
|
is_expanded = !is_expanded;
|
|
|
|
g_print ("Animation complete\n");
|
|
|
|
clutter_actor_set_reactive (actor, TRUE);
|
|
}
|
|
|
|
static gboolean
|
|
on_button_press (ClutterActor *actor,
|
|
ClutterButtonEvent *event,
|
|
gpointer dummy)
|
|
{
|
|
ClutterAnimation *animation;
|
|
gint old_x, old_y, new_x, new_y;
|
|
guint old_width, old_height, new_width, new_height;
|
|
gdouble new_angle;
|
|
ClutterVertex vertex = { 0, };
|
|
ClutterColor new_color = { 0, };
|
|
|
|
clutter_actor_get_position (actor, &old_x, &old_y);
|
|
clutter_actor_get_size (actor, &old_width, &old_height);
|
|
|
|
/* determine the final state of the animation depending on
|
|
* the state of the actor
|
|
*/
|
|
if (!is_expanded)
|
|
{
|
|
new_x = old_x - 100;
|
|
new_y = old_y - 100;
|
|
new_width = old_width + 200;
|
|
new_height = old_height + 200;
|
|
new_angle = 360.0;
|
|
|
|
new_color.red = 0xdd;
|
|
new_color.green = 0x44;
|
|
new_color.blue = 0xdd;
|
|
new_color.alpha = 0xff;
|
|
}
|
|
else
|
|
{
|
|
new_x = old_x + 100;
|
|
new_y = old_y + 100;
|
|
new_width = old_width - 200;
|
|
new_height = old_height - 200;
|
|
new_angle = 0.0;
|
|
|
|
new_color.red = 0x44;
|
|
new_color.green = 0xdd;
|
|
new_color.blue = 0x44;
|
|
new_color.alpha = 0x88;
|
|
}
|
|
|
|
vertex.x = CLUTTER_UNITS_FROM_FLOAT ((float) new_width / 2);
|
|
vertex.y = CLUTTER_UNITS_FROM_FLOAT ((float) new_height / 2);
|
|
|
|
animation =
|
|
clutter_actor_animate (actor, CLUTTER_EASE_IN_EXPO, 2000,
|
|
"x", new_x,
|
|
"y", new_y,
|
|
"width", new_width,
|
|
"height", new_height,
|
|
"color", &new_color,
|
|
"rotation-angle-z", new_angle,
|
|
"fixed::rotation-center-z", &vertex,
|
|
"fixed::reactive", FALSE,
|
|
NULL);
|
|
g_signal_connect (animation,
|
|
"completed", G_CALLBACK (on_animation_complete),
|
|
actor);
|
|
|
|
return TRUE;
|
|
}
|
|
|
|
G_MODULE_EXPORT int
|
|
test_animation_main (int argc, char *argv[])
|
|
{
|
|
ClutterActor *stage, *rect;
|
|
ClutterColor stage_color = { 0x66, 0x66, 0xdd, 0xff };
|
|
ClutterColor rect_color = { 0x44, 0xdd, 0x44, 0xff };
|
|
|
|
clutter_init (&argc, &argv);
|
|
|
|
stage = clutter_stage_get_default ();
|
|
clutter_stage_set_color (CLUTTER_STAGE (stage), &stage_color);
|
|
|
|
rect = clutter_rectangle_new_with_color (&rect_color);
|
|
clutter_container_add_actor (CLUTTER_CONTAINER (stage), rect);
|
|
clutter_actor_set_size (rect, 50, 50);
|
|
clutter_actor_set_anchor_point (rect, 25, 25);
|
|
clutter_actor_set_position (rect,
|
|
clutter_actor_get_width (stage) / 2,
|
|
clutter_actor_get_height (stage) / 2);
|
|
clutter_actor_set_opacity (rect, 0x88);
|
|
clutter_actor_set_reactive (rect, TRUE);
|
|
g_signal_connect (rect,
|
|
"button-press-event", G_CALLBACK (on_button_press),
|
|
NULL);
|
|
|
|
clutter_actor_show (stage);
|
|
|
|
clutter_main ();
|
|
|
|
return EXIT_SUCCESS;
|
|
}
|