mirror of
https://github.com/brl/mutter.git
synced 2024-12-02 12:50:53 -05:00
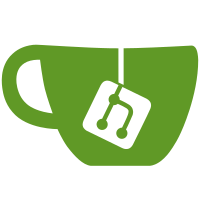
* clutter/clutter-timeline.h: * clutter/clutter-timeline.c: Add a "started" and a "paused" signals; add more sanity checks on the public functions. (clutter_timeline_get_loop): Add a getter function for the loop property. * clutter/clutter-marshal.list: Add marshallers. * clutter/clutter-timeline.h: * clutter/clutter-actor.h: Add padding for future expansion without breaking ABI.
164 lines
3.0 KiB
Scheme
164 lines
3.0 KiB
Scheme
;; -*- scheme -*-
|
|
;;
|
|
;; Try and keep everything sorted
|
|
;;
|
|
|
|
;; Boxed types
|
|
|
|
(define-boxed Color
|
|
(in-module "Clutter")
|
|
(c-name "ClutterColor")
|
|
(gtype-id "CLUTTER_TYPE_COLOR")
|
|
(fields
|
|
'("guint8" "red")
|
|
'("guint8" "green")
|
|
'("guint8" "blue")
|
|
'("guint8" "alpha")
|
|
)
|
|
)
|
|
|
|
(define-boxed ActorBox
|
|
(in-module "Clutter")
|
|
(c-name "ClutterActorBox")
|
|
(gtype-id "CLUTTER_TYPE_ACTOR_BOX")
|
|
(fields
|
|
'("gint" "x1")
|
|
'("gint" "y1")
|
|
'("gint" "x2")
|
|
'("gint" "y2")
|
|
)
|
|
)
|
|
|
|
(define-boxed Event
|
|
(in-module "Clutter")
|
|
(c-name "ClutterEvent")
|
|
(gtype-id "CLUTTER_TYPE_EVENT")
|
|
(fields
|
|
'("ClutterEventType" "type")
|
|
)
|
|
)
|
|
|
|
(define-boxed Geometry
|
|
(in-module "Clutter")
|
|
(c-name "ClutterGeometry")
|
|
(gtype-id "CLUTTER_TYPE_GEOMETRY")
|
|
(fields
|
|
'("gint" "x")
|
|
'("gint" "y")
|
|
'("gint" "width")
|
|
'("gint" "height")
|
|
)
|
|
)
|
|
|
|
;; Enumerations and flags ...
|
|
|
|
(define-flags ActorFlags
|
|
(in-module "Clutter")
|
|
(c-name "ClutterActorFlags")
|
|
(gtype-id "CLUTTER_TYPE_ACTOR_FLAGS")
|
|
(values
|
|
'("mapped" "CLUTTER_ACTOR_MAPPED")
|
|
'("realized" "CLUTTER_ACTOR_REALIZED")
|
|
)
|
|
)
|
|
|
|
(define-enum EventType
|
|
(in-module "Clutter")
|
|
(c-name "ClutterEventType")
|
|
(gtype-id "CLUTTER_TYPE_EVENT_TYPE")
|
|
(values
|
|
'("nothing" "CLUTTER_NOTHING")
|
|
'("key-press" "CLUTTER_KEY_PRESS")
|
|
'("key-release" "CLUTTER_KEY_RELEASE")
|
|
'("motion" "CLUTTER_MOTION")
|
|
'("button-press" "CLUTTER_BUTTON_PRESS")
|
|
'("2button-press" "CLUTTER_2BUTTON_PRESS")
|
|
'("button-release" "CLUTTER_BUTTON_RELEASE")
|
|
)
|
|
)
|
|
|
|
;; Interfaces
|
|
|
|
(define-interface Media
|
|
(in-module "Clutter")
|
|
(c-name "ClutterMedia")
|
|
(gtype-id "CLUTTER_TYPE_MEDIA")
|
|
(vtable "ClutterMediaInterface")
|
|
)
|
|
|
|
;; Objects
|
|
|
|
(define-object CloneTexture
|
|
(in-module "Clutter")
|
|
(parent "ClutterActor")
|
|
(c-name "ClutterCloneTexture")
|
|
(gtype-id "CLUTTER_TYPE_CLONE_TEXTURE")
|
|
)
|
|
|
|
(define-object Actor
|
|
(in-module "Clutter")
|
|
(parent "GObject")
|
|
(c-name "ClutterActor")
|
|
(gtype-id "CLUTTER_TYPE_ACTOR")
|
|
)
|
|
|
|
(define-object Group
|
|
(in-module "Clutter")
|
|
(parent "ClutterActor")
|
|
(c-name "ClutterGroup")
|
|
(gtype-id "CLUTTER_TYPE_GROUP")
|
|
)
|
|
|
|
(define-object Label
|
|
(in-module "Clutter")
|
|
(parent "ClutterTexture")
|
|
(c-name "ClutterLabel")
|
|
(gtype-id "CLUTTER_TYPE_LABEL")
|
|
)
|
|
|
|
(define-object Rectangle
|
|
(in-module "Clutter")
|
|
(parent "ClutterActor")
|
|
(c-name "ClutterRectangle")
|
|
(gtype-id "CLUTTER_TYPE_RECTANGLE")
|
|
)
|
|
|
|
(define-object Stage
|
|
(in-module "Clutter")
|
|
(parent "ClutterGroup")
|
|
(c-name "ClutterStage")
|
|
(gtype-id "CLUTTER_TYPE_STAGE")
|
|
)
|
|
|
|
(define-object Texture
|
|
(in-module "Clutter")
|
|
(parent "ClutterActor")
|
|
(c-name "ClutterTexture")
|
|
(gtype-id "CLUTTER_TYPE_TEXTURE")
|
|
)
|
|
|
|
(define-object Timeline
|
|
(in-module "Clutter")
|
|
(parent "GObject")
|
|
(c-name "ClutterTimeline")
|
|
(gtype-id "CLUTTER_TYPE_TIMELINE")
|
|
)
|
|
|
|
(define-object VideoTexture
|
|
(in-module "Clutter")
|
|
(parent "ClutterTexture")
|
|
(implements "ClutterMedia")
|
|
(c-name "ClutterVideoTexture")
|
|
(gtype-id "CLUTTER_TYPE_VIDEO_TEXTURE")
|
|
)
|
|
|
|
|
|
;; Pointers
|
|
|
|
|
|
|
|
;; Unsupported
|
|
|
|
|
|
|