mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
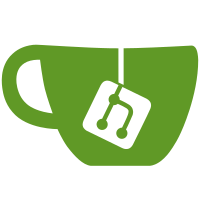
When we are in the the event translation function sometimes we need to synthesise events: the double and triple click events are synthetic events placed on the queue after a sequence of events has been received, for instance. Until now, the events were placed on the queue after the translation from the native events was successful. This led to a loss of ordering because we put the synthesised event on the queue before the last event that triggered it. This patch puts the events on the queue before translating them, with a "pending" flag set; if the translation sequence is completed then the flag is removed - otherwise the event is removed from the queue altogether. The queue manipulation functions have been modified to ignore the "pending" flag when looking for events. This patch also adds a private structure overlayed on the ClutterEvent struct so that we can extend the events with private data without exposing it in the public API.
165 lines
5.4 KiB
C
165 lines
5.4 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2006 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef _HAVE_CLUTTER_PRIVATE_H
|
|
#define _HAVE_CLUTTER_PRIVATE_H
|
|
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <unistd.h>
|
|
#include <math.h>
|
|
|
|
#include <glib.h>
|
|
|
|
#include <pango/pangoft2.h>
|
|
|
|
#include "clutter-event.h"
|
|
#include "clutter-backend.h"
|
|
#include "clutter-stage.h"
|
|
#include "clutter-feature.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
typedef enum {
|
|
CLUTTER_ACTOR_UNUSED_FLAG = 0,
|
|
|
|
CLUTTER_ACTOR_IN_DESTRUCTION = 1 << 0,
|
|
CLUTTER_ACTOR_IS_TOPLEVEL = 1 << 1,
|
|
CLUTTER_ACTOR_IN_REPARENT = 1 << 2,
|
|
CLUTTER_ACTOR_SYNC_MATRICES = 1 << 3 /* Used by stage to indicate GL
|
|
* viewport / perspective etc
|
|
* needs (re)setting.
|
|
*/
|
|
} ClutterPrivateFlags;
|
|
|
|
typedef enum {
|
|
CLUTTER_PICK_NONE = 0,
|
|
CLUTTER_PICK_REACTIVE,
|
|
CLUTTER_PICK_ALL
|
|
} ClutterPickMode;
|
|
|
|
typedef enum {
|
|
/* this flag is set when an event has been put on the queue but still
|
|
* needs processing; the event queue must ignore every event with this
|
|
* flag set
|
|
*/
|
|
CLUTTER_EVENT_PENDING = 1 << 0
|
|
} ClutterEventFlags;
|
|
|
|
typedef struct _ClutterEventPrivate ClutterEventPrivate;
|
|
typedef struct _ClutterMainContext ClutterMainContext;
|
|
|
|
/* Private structure, to be used for extending ClutterEvent without
|
|
* exposing new members and breaking compatibility.
|
|
*/
|
|
struct _ClutterEventPrivate
|
|
{
|
|
ClutterEvent event;
|
|
guint flags;
|
|
};
|
|
|
|
struct _ClutterMainContext
|
|
{
|
|
/* holds a pointer to the backend, which controls the stage */
|
|
ClutterBackend *backend;
|
|
|
|
/* the main event queue */
|
|
GQueue *events_queue;
|
|
|
|
PangoFT2FontMap *font_map;
|
|
|
|
guint update_idle;
|
|
|
|
guint is_initialized : 1;
|
|
GTimer *timer; /* Used for debugging scheduler */
|
|
|
|
ClutterPickMode pick_mode; /* Indicates pick render mode */
|
|
guint motion_events_per_actor : 1;
|
|
gint num_reactives; /* Num of reactive actors */
|
|
};
|
|
|
|
#define CLUTTER_CONTEXT() (clutter_context_get_default ())
|
|
ClutterMainContext *clutter_context_get_default (void);
|
|
|
|
#define CLUTTER_PRIVATE_FLAGS(a) (CLUTTER_ACTOR ((a))->private_flags)
|
|
#define CLUTTER_SET_PRIVATE_FLAGS(a,f) G_STMT_START{ (CLUTTER_PRIVATE_FLAGS (a) |= (f)); }G_STMT_END
|
|
#define CLUTTER_UNSET_PRIVATE_FLAGS(a,f) G_STMT_START{ (CLUTTER_PRIVATE_FLAGS (a) &= ~(f)); }G_STMT_END
|
|
|
|
#define CLUTTER_PARAM_READABLE \
|
|
G_PARAM_READABLE | G_PARAM_STATIC_NAME | G_PARAM_STATIC_NICK | G_PARAM_STATIC_BLURB
|
|
#define CLUTTER_PARAM_WRITABLE \
|
|
G_PARAM_WRITABLE | G_PARAM_STATIC_NAME | G_PARAM_STATIC_NICK | G_PARAM_STATIC_BLURB
|
|
#define CLUTTER_PARAM_READWRITE \
|
|
G_PARAM_READABLE | G_PARAM_WRITABLE | G_PARAM_STATIC_NAME | G_PARAM_STATIC_NICK |G_PARAM_STATIC_BLURB
|
|
|
|
/* signal accumulators */
|
|
gboolean _clutter_boolean_accumulator (GSignalInvocationHint *ihint,
|
|
GValue *return_accu,
|
|
const GValue *handler_return,
|
|
gpointer dummy);
|
|
|
|
/* vfuncs implemnted by backend */
|
|
|
|
GType _clutter_backend_impl_get_type (void);
|
|
|
|
ClutterActor *_clutter_backend_get_stage (ClutterBackend *backend);
|
|
|
|
void _clutter_backend_redraw (ClutterBackend *backend);
|
|
|
|
void _clutter_backend_add_options (ClutterBackend *backend,
|
|
GOptionGroup *group);
|
|
gboolean _clutter_backend_pre_parse (ClutterBackend *backend,
|
|
GError **error);
|
|
gboolean _clutter_backend_post_parse (ClutterBackend *backend,
|
|
GError **error);
|
|
gboolean _clutter_backend_init_stage (ClutterBackend *backend,
|
|
GError **error);
|
|
void _clutter_backend_init_events (ClutterBackend *backend);
|
|
|
|
ClutterFeatureFlags _clutter_backend_get_features (ClutterBackend *backend);
|
|
|
|
/* backend helpers */
|
|
void _clutter_event_button_generate (ClutterBackend *backend,
|
|
ClutterEvent *event);
|
|
|
|
void _clutter_feature_init (void);
|
|
|
|
ClutterActor* _clutter_do_pick (ClutterStage *stage,
|
|
gint x,
|
|
gint y,
|
|
ClutterPickMode mode);
|
|
|
|
/* Does this need to be private ? */
|
|
void clutter_do_event (ClutterEvent *event);
|
|
|
|
void _clutter_actor_apply_modelview_transform (ClutterActor * self);
|
|
|
|
void _clutter_actor_apply_modelview_transform_recursive (ClutterActor * self);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* _HAVE_CLUTTER_PRIVATE_H */
|