mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 06:25:51 -04:00
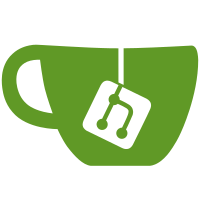
This adds a CoglGpuInfo struct to the CoglContext which contains some enums describing the GL driver in use. This currently includes the driver package (ie, is it Mesa) the version number of the package and the vendor of the GPU (ie, is it by Intel). There is also a bitmask which will contain the workarounds that we should do for that particular driver configuration. The struct is initialised on context creation by using a series of string comparisons on the strings returned from glGetString. Reviewed-by: Robert Bragg <robert@linux.intel.com>
75 lines
1.8 KiB
C
75 lines
1.8 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2012 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_GPU_INFO_PRIVATE_H
|
|
#define __COGL_GPU_INFO_PRIVATE_H
|
|
|
|
#include "cogl-context.h"
|
|
|
|
typedef enum
|
|
{
|
|
COGL_GPU_INFO_VENDOR_UNKNOWN,
|
|
COGL_GPU_INFO_VENDOR_INTEL
|
|
} CoglGpuInfoVendor;
|
|
|
|
typedef enum
|
|
{
|
|
COGL_GPU_INFO_DRIVER_PACKAGE_UNKNOWN,
|
|
COGL_GPU_INFO_DRIVER_PACKAGE_MESA
|
|
} CoglGpuInfoDriverPackage;
|
|
|
|
typedef enum
|
|
{
|
|
COGL_GPU_INFO_DRIVER_STUB
|
|
} CoglGpuInfoDriverBug;
|
|
|
|
typedef struct _CoglGpuInfoVersion CoglGpuInfoVersion;
|
|
|
|
typedef struct _CoglGpuInfo CoglGpuInfo;
|
|
|
|
struct _CoglGpuInfo
|
|
{
|
|
CoglGpuInfoVendor vendor;
|
|
const char *vendor_name;
|
|
|
|
CoglGpuInfoDriverPackage driver_package;
|
|
const char *driver_package_name;
|
|
int driver_package_version;
|
|
|
|
CoglGpuInfoDriverBug driver_bugs;
|
|
};
|
|
|
|
/*
|
|
* _cogl_gpu_info_init:
|
|
* @ctx: A #CoglContext
|
|
* @gpu: A return location for the GPU information
|
|
*
|
|
* Determines information about the GPU and driver from the given
|
|
* context.
|
|
*/
|
|
void
|
|
_cogl_gpu_info_init (CoglContext *ctx,
|
|
CoglGpuInfo *gpu);
|
|
|
|
#endif /* __COGL_GPU_INFO_PRIVATE_H */
|