mirror of
https://github.com/brl/mutter.git
synced 2024-09-20 14:35:48 -04:00
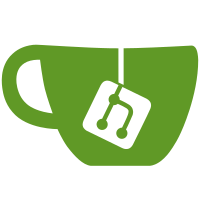
Since we'll want to share the fallback logic with CoglVertexArray this moves the malloc based fallback (for when OpenGL doesn't support vertex or pixel buffer objects) into cogl-buffer.c.
149 lines
4.4 KiB
C
149 lines
4.4 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*
|
|
* Authors:
|
|
* Damien Lespiau <damien.lespiau@intel.com>
|
|
* Robert Bragg <robert@linux.intel.com>
|
|
*/
|
|
|
|
#ifndef __COGL_BUFFER_PRIVATE_H__
|
|
#define __COGL_BUFFER_PRIVATE_H__
|
|
|
|
#include <glib.h>
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-object.h"
|
|
#include "cogl-buffer.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define COGL_BUFFER(buffer) ((CoglBuffer *)(buffer))
|
|
|
|
#define COGL_BUFFER_SET_FLAG(buffer, flag) \
|
|
((buffer)->flags |= (COGL_BUFFER_FLAG_ ## flag))
|
|
|
|
#define COGL_BUFFER_CLEAR_FLAG(buffer, flag) \
|
|
((buffer)->flags &= ~(COGL_BUFFER_FLAG_ ## flag))
|
|
|
|
#define COGL_BUFFER_FLAG_IS_SET(buffer, flag) \
|
|
((buffer)->flags & (COGL_BUFFER_FLAG_ ## flag))
|
|
|
|
typedef struct _CoglBufferVtable CoglBufferVtable;
|
|
|
|
struct _CoglBufferVtable
|
|
{
|
|
guint8 * (* map) (CoglBuffer *buffer,
|
|
CoglBufferAccess access,
|
|
CoglBufferMapHint hints);
|
|
|
|
void (* unmap) (CoglBuffer *buffer);
|
|
|
|
gboolean (* set_data) (CoglBuffer *buffer,
|
|
unsigned int offset,
|
|
const guint8 *data,
|
|
unsigned int size);
|
|
};
|
|
|
|
typedef enum _CoglBufferFlags
|
|
{
|
|
COGL_BUFFER_FLAG_NONE = 0,
|
|
COGL_BUFFER_FLAG_BUFFER_OBJECT = 1UL << 0, /* real openGL buffer object */
|
|
COGL_BUFFER_FLAG_MAPPED = 1UL << 1
|
|
} CoglBufferFlags;
|
|
|
|
typedef enum {
|
|
COGL_BUFFER_USAGE_HINT_TEXTURE,
|
|
COGL_BUFFER_USAGE_HINT_VERTICES
|
|
} CoglBufferUsageHint;
|
|
|
|
typedef enum {
|
|
COGL_BUFFER_BIND_TARGET_PIXEL_PACK,
|
|
COGL_BUFFER_BIND_TARGET_PIXEL_UNPACK,
|
|
COGL_BUFFER_BIND_TARGET_VERTEX_ARRAY,
|
|
COGL_BUFFER_BIND_TARGET_VERTEX_INDICES_ARRAY,
|
|
|
|
COGL_BUFFER_BIND_TARGET_COUNT
|
|
} CoglBufferBindTarget;
|
|
|
|
struct _CoglBuffer
|
|
{
|
|
CoglObject _parent;
|
|
CoglBufferVtable vtable;
|
|
|
|
CoglBufferBindTarget last_target;
|
|
|
|
CoglBufferFlags flags;
|
|
|
|
GLuint gl_handle; /* OpenGL handle */
|
|
unsigned int size; /* size of the buffer, in bytes */
|
|
CoglBufferUsageHint usage_hint;
|
|
CoglBufferUpdateHint update_hint;
|
|
|
|
guint8 *data; /* points to the mapped memory when
|
|
* the CoglBuffer is a VBO, PBO, ... or
|
|
* points to allocated memory in the
|
|
* fallback paths */
|
|
};
|
|
|
|
/* This is used to register a type to the list of handle types that
|
|
will be considered a texture in cogl_is_texture() */
|
|
void
|
|
_cogl_buffer_register_buffer_type (GQuark type);
|
|
|
|
#define COGL_BUFFER_DEFINE(TypeName, type_name) \
|
|
COGL_OBJECT_DEFINE_WITH_CODE \
|
|
(TypeName, type_name, \
|
|
_cogl_buffer_register_buffer_type (_cogl_object_ \
|
|
## type_name ## _get_type ()))
|
|
|
|
void
|
|
_cogl_buffer_initialize (CoglBuffer *buffer,
|
|
unsigned int size,
|
|
gboolean use_malloc,
|
|
CoglBufferBindTarget default_target,
|
|
CoglBufferUsageHint usage_hint,
|
|
CoglBufferUpdateHint update_hint);
|
|
|
|
void
|
|
_cogl_buffer_fini (CoglBuffer *buffer);
|
|
|
|
void
|
|
_cogl_buffer_bind (CoglBuffer *buffer,
|
|
CoglBufferBindTarget target);
|
|
|
|
void
|
|
_cogl_buffer_unbind (CoglBuffer *buffer);
|
|
|
|
CoglBufferUsageHint
|
|
_cogl_buffer_get_usage_hint (CoglBuffer *buffer);
|
|
|
|
GLenum
|
|
_cogl_buffer_access_to_gl_enum (CoglBufferAccess access);
|
|
|
|
GLenum
|
|
_cogl_buffer_hints_to_gl_enum (CoglBufferUsageHint usage_hint,
|
|
CoglBufferUpdateHint update_hint);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __COGL_BUFFER_PRIVATE_H__ */
|