mirror of
https://github.com/brl/mutter.git
synced 2024-11-11 16:56:16 -05:00
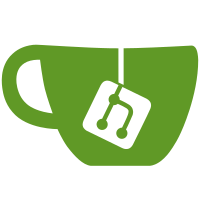
2002-02-08 Havoc Pennington <hp@pobox.com> * src/window.c (meta_window_show): when mapping a window with struts, invalidate the work areas it's on. Should fix at least part of the problem with windows maximizing over panels. * src/workspace.c (meta_workspace_invalidate_work_area): also queue move/resize on sticky windows * src/tools/Makefile.am: consolidate reload-theme, restart into a "metacity-message" app and add enable/disable keybindings to the messages it knows about. * src/keybindings.c: (meta_change_keygrab): grab keyboard synchronously (meta_display_process_key_event): if all keybindings are toggled off, ReplayKeyboard, else AsyncKeyboard, except that the debug binding for toggling back on is always processed (meta_set_keybindings_disabled): function to disable/enable all keybindings
140 lines
3.7 KiB
C
140 lines
3.7 KiB
C
/* Metacity send-magic-messages app */
|
|
|
|
/*
|
|
* Copyright (C) 2002 Havoc Pennington
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
|
|
* 02111-1307, USA.
|
|
*/
|
|
|
|
#include <gtk/gtk.h>
|
|
#include <gdk/gdkx.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
static void
|
|
send_restart (void)
|
|
{
|
|
XEvent xev;
|
|
|
|
xev.xclient.type = ClientMessage;
|
|
xev.xclient.serial = 0;
|
|
xev.xclient.send_event = True;
|
|
xev.xclient.display = gdk_display;
|
|
xev.xclient.window = gdk_x11_get_default_root_xwindow ();
|
|
xev.xclient.message_type = XInternAtom (gdk_display,
|
|
"_METACITY_RESTART_MESSAGE",
|
|
False);
|
|
xev.xclient.format = 32;
|
|
xev.xclient.data.l[0] = 0;
|
|
xev.xclient.data.l[1] = 0;
|
|
xev.xclient.data.l[2] = 0;
|
|
|
|
XSendEvent (gdk_display,
|
|
gdk_x11_get_default_root_xwindow (),
|
|
False,
|
|
SubstructureRedirectMask | SubstructureNotifyMask,
|
|
&xev);
|
|
|
|
XFlush (gdk_display);
|
|
XSync (gdk_display, False);
|
|
}
|
|
|
|
static void
|
|
send_reload_theme (void)
|
|
{
|
|
XEvent xev;
|
|
|
|
xev.xclient.type = ClientMessage;
|
|
xev.xclient.serial = 0;
|
|
xev.xclient.send_event = True;
|
|
xev.xclient.display = gdk_display;
|
|
xev.xclient.window = gdk_x11_get_default_root_xwindow ();
|
|
xev.xclient.message_type = XInternAtom (gdk_display,
|
|
"_METACITY_RELOAD_THEME_MESSAGE",
|
|
False);
|
|
xev.xclient.format = 32;
|
|
xev.xclient.data.l[0] = 0;
|
|
xev.xclient.data.l[1] = 0;
|
|
xev.xclient.data.l[2] = 0;
|
|
|
|
XSendEvent (gdk_display,
|
|
gdk_x11_get_default_root_xwindow (),
|
|
False,
|
|
SubstructureRedirectMask | SubstructureNotifyMask,
|
|
&xev);
|
|
|
|
XFlush (gdk_display);
|
|
XSync (gdk_display, False);
|
|
}
|
|
|
|
static void
|
|
send_set_keybindings (gboolean enabled)
|
|
{
|
|
XEvent xev;
|
|
|
|
xev.xclient.type = ClientMessage;
|
|
xev.xclient.serial = 0;
|
|
xev.xclient.send_event = True;
|
|
xev.xclient.display = gdk_display;
|
|
xev.xclient.window = gdk_x11_get_default_root_xwindow ();
|
|
xev.xclient.message_type = XInternAtom (gdk_display,
|
|
"_METACITY_SET_KEYBINDINGS_MESSAGE",
|
|
False);
|
|
xev.xclient.format = 32;
|
|
xev.xclient.data.l[0] = enabled;
|
|
xev.xclient.data.l[1] = 0;
|
|
xev.xclient.data.l[2] = 0;
|
|
|
|
XSendEvent (gdk_display,
|
|
gdk_x11_get_default_root_xwindow (),
|
|
False,
|
|
SubstructureRedirectMask | SubstructureNotifyMask,
|
|
&xev);
|
|
|
|
XFlush (gdk_display);
|
|
XSync (gdk_display, False);
|
|
}
|
|
|
|
static void
|
|
usage (void)
|
|
{
|
|
g_printerr ("Usage: metacity-message (restart|reload-theme|enable-keybindings|disable-keybindings)\n");
|
|
exit (1);
|
|
}
|
|
|
|
int
|
|
main (int argc, char **argv)
|
|
{
|
|
gtk_init (&argc, &argv);
|
|
|
|
if (argc < 2)
|
|
usage ();
|
|
|
|
if (strcmp (argv[1], "restart") == 0)
|
|
send_restart ();
|
|
else if (strcmp (argv[1], "reload-theme") == 0)
|
|
send_reload_theme ();
|
|
else if (strcmp (argv[1], "enable-keybindings") == 0)
|
|
send_set_keybindings (TRUE);
|
|
else if (strcmp (argv[1], "disable-keybindings") == 0)
|
|
send_set_keybindings (FALSE);
|
|
else
|
|
usage ();
|
|
|
|
return 0;
|
|
}
|
|
|