mirror of
https://github.com/brl/mutter.git
synced 2025-07-10 20:47:19 +00:00
backends/native: Add libinput-based MetaInputSettings implementation
The libinput_device is fetched from the ClutterInputDevice, and configured through the libinput_device_*config* API. https://bugzilla.gnome.org/show_bug.cgi?id=739397
This commit is contained in:
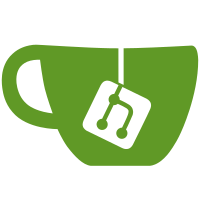
committed by
Jasper St. Pierre
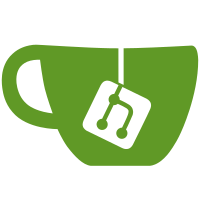
parent
3c06f2dc90
commit
2d878d3f55
@ -275,6 +275,8 @@ libmutter_la_SOURCES += \
|
||||
backends/native/meta-cursor-renderer-native.h \
|
||||
backends/native/meta-idle-monitor-native.c \
|
||||
backends/native/meta-idle-monitor-native.h \
|
||||
backends/native/meta-input-settings-native.c \
|
||||
backends/native/meta-input-settings-native.h \
|
||||
backends/native/meta-monitor-manager-kms.c \
|
||||
backends/native/meta-monitor-manager-kms.h \
|
||||
backends/native/meta-launcher.c \
|
||||
|
@ -33,6 +33,11 @@
|
||||
#include "meta-input-settings-private.h"
|
||||
#include "x11/meta-input-settings-x11.h"
|
||||
|
||||
#ifdef HAVE_NATIVE_BACKEND
|
||||
#include "native/meta-backend-native.h"
|
||||
#include "native/meta-input-settings-native.h"
|
||||
#endif
|
||||
|
||||
#include <meta/util.h>
|
||||
|
||||
typedef struct _MetaInputSettingsPrivate MetaInputSettingsPrivate;
|
||||
@ -604,6 +609,14 @@ meta_input_settings_init (MetaInputSettings *settings)
|
||||
MetaInputSettings *
|
||||
meta_input_settings_create (void)
|
||||
{
|
||||
#ifdef HAVE_NATIVE_BACKEND
|
||||
MetaBackend *backend;
|
||||
|
||||
backend = meta_get_backend ();
|
||||
|
||||
if (META_IS_BACKEND_NATIVE (backend))
|
||||
return g_object_new (META_TYPE_INPUT_SETTINGS_NATIVE, NULL);
|
||||
#endif
|
||||
if (!meta_is_wayland_compositor ())
|
||||
return g_object_new (META_TYPE_INPUT_SETTINGS_X11, NULL);
|
||||
|
||||
|
217
src/backends/native/meta-input-settings-native.c
Normal file
217
src/backends/native/meta-input-settings-native.c
Normal file
@ -0,0 +1,217 @@
|
||||
/* -*- mode: C; c-file-style: "gnu"; indent-tabs-mode: nil; -*- */
|
||||
|
||||
/*
|
||||
* Copyright (C) 2014 Red Hat
|
||||
*
|
||||
* This program is free software; you can redistribute it and/or
|
||||
* modify it under the terms of the GNU General Public License as
|
||||
* published by the Free Software Foundation; either version 2 of the
|
||||
* License, or (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful, but
|
||||
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
||||
* General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program; if not, write to the Free Software
|
||||
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
|
||||
* 02111-1307, USA.
|
||||
*
|
||||
* Author: Carlos Garnacho <carlosg@gnome.org>
|
||||
*/
|
||||
|
||||
#include "config.h"
|
||||
|
||||
#include <clutter/evdev/clutter-evdev.h>
|
||||
#include <libinput.h>
|
||||
|
||||
#include "meta-input-settings-native.h"
|
||||
|
||||
G_DEFINE_TYPE (MetaInputSettingsNative, meta_input_settings_native, META_TYPE_INPUT_SETTINGS)
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_send_events (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
GDesktopDeviceSendEvents mode)
|
||||
{
|
||||
enum libinput_config_send_events_mode libinput_mode;
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
switch (mode)
|
||||
{
|
||||
case G_DESKTOP_DEVICE_SEND_EVENTS_DISABLED:
|
||||
libinput_mode = LIBINPUT_CONFIG_SEND_EVENTS_DISABLED;
|
||||
break;
|
||||
case G_DESKTOP_DEVICE_SEND_EVENTS_DISABLED_ON_EXTERNAL_MOUSE:
|
||||
libinput_mode = LIBINPUT_CONFIG_SEND_EVENTS_DISABLED_ON_EXTERNAL_MOUSE;
|
||||
break;
|
||||
case G_DESKTOP_DEVICE_SEND_EVENTS_ENABLED:
|
||||
libinput_mode = LIBINPUT_CONFIG_SEND_EVENTS_ENABLED;
|
||||
break;
|
||||
default:
|
||||
g_assert_not_reached ();
|
||||
}
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
libinput_device_config_send_events_set_mode (libinput_device, libinput_mode);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_matrix (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
gfloat matrix[6])
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
if (libinput_device_config_calibration_has_matrix (libinput_device) > 0)
|
||||
libinput_device_config_calibration_set_matrix (libinput_device, matrix);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_speed (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
gdouble speed)
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
libinput_device_config_accel_set_speed (libinput_device,
|
||||
CLAMP (speed, -1, 1));
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_left_handed (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
gboolean enabled)
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
if (libinput_device_config_left_handed_is_available (libinput_device))
|
||||
libinput_device_config_left_handed_set (libinput_device, enabled);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_tap_enabled (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
gboolean enabled)
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
if (libinput_device_config_tap_get_finger_count (libinput_device) > 0)
|
||||
libinput_device_config_tap_set_enabled (libinput_device,
|
||||
enabled ?
|
||||
LIBINPUT_CONFIG_TAP_ENABLED :
|
||||
LIBINPUT_CONFIG_TAP_DISABLED);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_invert_scroll (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
gboolean inverted)
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
if (libinput_device_config_scroll_has_natural_scroll (libinput_device))
|
||||
libinput_device_config_scroll_set_natural_scroll_enabled (libinput_device,
|
||||
inverted);
|
||||
}
|
||||
|
||||
static gboolean
|
||||
device_set_scroll_method (struct libinput_device *libinput_device,
|
||||
enum libinput_config_scroll_method method)
|
||||
{
|
||||
enum libinput_config_scroll_method supported;
|
||||
|
||||
supported = libinput_device_config_scroll_get_methods (libinput_device);
|
||||
|
||||
if (method & supported)
|
||||
libinput_device_config_scroll_set_method (libinput_device, method);
|
||||
|
||||
return (method & supported) != 0;
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_scroll_method (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
GDesktopTouchpadScrollMethod mode)
|
||||
{
|
||||
enum libinput_config_scroll_method scroll_method = 0;
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
switch (mode)
|
||||
{
|
||||
case G_DESKTOP_TOUCHPAD_SCROLL_METHOD_DISABLED:
|
||||
scroll_method = LIBINPUT_CONFIG_SCROLL_NO_SCROLL;
|
||||
break;
|
||||
case G_DESKTOP_TOUCHPAD_SCROLL_METHOD_EDGE_SCROLLING:
|
||||
scroll_method = LIBINPUT_CONFIG_SCROLL_2FG;
|
||||
break;
|
||||
case G_DESKTOP_TOUCHPAD_SCROLL_METHOD_TWO_FINGER_SCROLLING:
|
||||
scroll_method = LIBINPUT_CONFIG_SCROLL_EDGE;
|
||||
break;
|
||||
default:
|
||||
g_assert_not_reached ();
|
||||
return;
|
||||
}
|
||||
|
||||
device_set_scroll_method (libinput_device, scroll_method);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_scroll_button (MetaInputSettings *settings,
|
||||
ClutterInputDevice *device,
|
||||
guint button)
|
||||
{
|
||||
struct libinput_device *libinput_device;
|
||||
|
||||
libinput_device = clutter_evdev_input_device_get_libinput_device (device);
|
||||
|
||||
if (!device_set_scroll_method (libinput_device,
|
||||
LIBINPUT_CONFIG_SCROLL_ON_BUTTON_DOWN))
|
||||
return;
|
||||
|
||||
libinput_device_config_scroll_set_button (libinput_device, button);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_set_keyboard_repeat (MetaInputSettings *settings,
|
||||
gboolean enabled,
|
||||
guint delay,
|
||||
guint interval)
|
||||
{
|
||||
ClutterDeviceManager *manager = clutter_device_manager_get_default ();
|
||||
|
||||
clutter_evdev_set_keyboard_repeat (manager, enabled, delay, interval);
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_class_init (MetaInputSettingsNativeClass *klass)
|
||||
{
|
||||
MetaInputSettingsClass *input_settings_class = META_INPUT_SETTINGS_CLASS (klass);
|
||||
|
||||
input_settings_class->set_send_events = meta_input_settings_native_set_send_events;
|
||||
input_settings_class->set_matrix = meta_input_settings_native_set_matrix;
|
||||
input_settings_class->set_speed = meta_input_settings_native_set_speed;
|
||||
input_settings_class->set_left_handed = meta_input_settings_native_set_left_handed;
|
||||
input_settings_class->set_tap_enabled = meta_input_settings_native_set_tap_enabled;
|
||||
input_settings_class->set_invert_scroll = meta_input_settings_native_set_invert_scroll;
|
||||
input_settings_class->set_scroll_method = meta_input_settings_native_set_scroll_method;
|
||||
input_settings_class->set_scroll_button = meta_input_settings_native_set_scroll_button;
|
||||
input_settings_class->set_keyboard_repeat = meta_input_settings_native_set_keyboard_repeat;
|
||||
}
|
||||
|
||||
static void
|
||||
meta_input_settings_native_init (MetaInputSettingsNative *settings)
|
||||
{
|
||||
}
|
49
src/backends/native/meta-input-settings-native.h
Normal file
49
src/backends/native/meta-input-settings-native.h
Normal file
@ -0,0 +1,49 @@
|
||||
/* -*- mode: C; c-file-style: "gnu"; indent-tabs-mode: nil; -*- */
|
||||
|
||||
/*
|
||||
* Copyright 2014 Red Hat, Inc.
|
||||
*
|
||||
* This program is free software; you can redistribute it and/or
|
||||
* modify it under the terms of the GNU General Public License as
|
||||
* published by the Free Software Foundation; either version 2 of the
|
||||
* License, or (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful, but
|
||||
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
||||
* General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
||||
*
|
||||
* Author: Carlos Garnacho <carlosg@gnome.org>
|
||||
*/
|
||||
|
||||
#ifndef META_INPUT_SETTINGS_NATIVE_H
|
||||
#define META_INPUT_SETTINGS_NATIVE_H
|
||||
|
||||
#include "meta-input-settings-private.h"
|
||||
|
||||
#define META_TYPE_INPUT_SETTINGS_NATIVE (meta_input_settings_native_get_type ())
|
||||
#define META_INPUT_SETTINGS_NATIVE(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), META_TYPE_INPUT_SETTINGS_NATIVE, MetaInputSettingsNative))
|
||||
#define META_INPUT_SETTINGS_NATIVE_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), META_TYPE_INPUT_SETTINGS_NATIVE, MetaInputSettingsNativeClass))
|
||||
#define META_IS_INPUT_SETTINGS_NATIVE(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), META_TYPE_INPUT_SETTINGS_NATIVE))
|
||||
#define META_IS_INPUT_SETTINGS_NATIVE_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), META_TYPE_INPUT_SETTINGS_NATIVE))
|
||||
#define META_INPUT_SETTINGS_NATIVE_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), META_TYPE_INPUT_SETTINGS_NATIVE, MetaInputSettingsNativeClass))
|
||||
|
||||
typedef struct _MetaInputSettingsNative MetaInputSettingsNative;
|
||||
typedef struct _MetaInputSettingsNativeClass MetaInputSettingsNativeClass;
|
||||
|
||||
struct _MetaInputSettingsNative
|
||||
{
|
||||
MetaInputSettings parent_instance;
|
||||
};
|
||||
|
||||
struct _MetaInputSettingsNativeClass
|
||||
{
|
||||
MetaInputSettingsClass parent_class;
|
||||
};
|
||||
|
||||
GType meta_input_settings_native_get_type (void) G_GNUC_CONST;
|
||||
|
||||
#endif /* META_INPUT_SETTINGS_NATIVE_H */
|
Reference in New Issue
Block a user