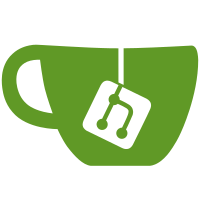
Right now the mockup is 1280x800. We don't fit at all well into 800x600. This patch bumps us up by default to 1024x768 which is the lowest we're going to work at for now, until we get some more intelligent resizing. svn path=/trunk/; revision=60
66 lines
1.8 KiB
Python
Executable File
66 lines
1.8 KiB
Python
Executable File
#!/usr/bin/python
|
|
|
|
import os
|
|
import random
|
|
import shutil
|
|
import signal
|
|
import subprocess
|
|
import sys
|
|
import tempfile
|
|
import time
|
|
|
|
from launcher import Launcher
|
|
|
|
launcher = Launcher()
|
|
|
|
# GL_EXT_texture_from_pixmap doesn't work in Xepyhr
|
|
launcher.set_use_tfp(False)
|
|
|
|
# Temporary directory to hold our X credentials
|
|
tmpdir = tempfile.mkdtemp("", "gnome-shell.")
|
|
try:
|
|
display = ":" + str(random.randint(10, 99))
|
|
xauth_file = os.path.join(tmpdir, "database")
|
|
|
|
# Create a random 128-byte key and format it as hex
|
|
f = open("/dev/urandom", "r")
|
|
key = f.read(16)
|
|
f.close()
|
|
hexkey = "".join(("%02x" % ord(byte) for byte in key))
|
|
|
|
# Store that in an xauthority file as the key for connecting to our Xephyr
|
|
retcode = subprocess.call(["xauth",
|
|
"-f", xauth_file,
|
|
"add", display, "MIT-MAGIC-COOKIE-1", hexkey])
|
|
if retcode != 0:
|
|
raise RuntimeError("xauth failed")
|
|
|
|
# Launch Xephyr
|
|
xephyr = subprocess.Popen(["Xephyr", display,
|
|
"-auth", xauth_file,
|
|
"-screen", "1024x748",
|
|
"-host-cursor"])
|
|
os.environ['DISPLAY'] = display
|
|
os.environ['XAUTHORITY'] = xauth_file
|
|
|
|
# Wait for server to get going: LAME
|
|
time.sleep(1)
|
|
|
|
# Start some windows in our session. Specify explicit geometries
|
|
# so we don't end up with the title bars underneath the panel
|
|
subprocess.Popen(["xterm", "-geometry", "+30+30"])
|
|
subprocess.Popen(["xlogo", "-geometry", "-0-0"])
|
|
subprocess.Popen(["xeyes", "-geometry", "-0+30"])
|
|
|
|
# Now launch metacity-clutter with our plugin
|
|
shell = launcher.start_shell()
|
|
|
|
# Wait for Xephyr to exit
|
|
try:
|
|
retcode = xephyr.wait()
|
|
except KeyboardInterrupt, e:
|
|
os.kill(xephyr.pid, signal.SIGKILL)
|
|
finally:
|
|
shutil.rmtree(tmpdir)
|
|
|