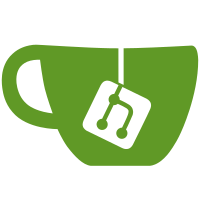
There's no good reason for waiting for the proxy to be initialized to connect signals. In fact, connecting the signal beforehand ensures that the handler is in place when the proxy fetches the properties, so we don't have to call the handler explicitly. That in turn will allow us in a follow-up to rely on the signal parameters to only process changed properties. To achieve that by constructing the proxy manually, and then initialize it asynchronously in a Promise. Part-of: <https://gitlab.gnome.org/GNOME/gnome-shell/-/merge_requests/2385>
114 lines
3.5 KiB
JavaScript
114 lines
3.5 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
/* exported Indicator */
|
|
|
|
const {Gio, GLib, GObject} = imports.gi;
|
|
const Signals = imports.misc.signals;
|
|
|
|
const Main = imports.ui.main;
|
|
const PanelMenu = imports.ui.panelMenu;
|
|
const PopupMenu = imports.ui.popupMenu;
|
|
|
|
const {loadInterfaceXML} = imports.misc.fileUtils;
|
|
|
|
const BUS_NAME = 'org.gnome.SettingsDaemon.Rfkill';
|
|
const OBJECT_PATH = '/org/gnome/SettingsDaemon/Rfkill';
|
|
|
|
const RfkillManagerInterface = loadInterfaceXML('org.gnome.SettingsDaemon.Rfkill');
|
|
const rfkillManagerInfo = Gio.DBusInterfaceInfo.new_for_xml(RfkillManagerInterface);
|
|
|
|
var RfkillManager = class extends Signals.EventEmitter {
|
|
constructor() {
|
|
super();
|
|
|
|
this._proxy = new Gio.DBusProxy({
|
|
g_connection: Gio.DBus.session,
|
|
g_name: BUS_NAME,
|
|
g_object_path: OBJECT_PATH,
|
|
g_interface_name: rfkillManagerInfo.name,
|
|
g_interface_info: rfkillManagerInfo,
|
|
});
|
|
this._proxy.connect('g-properties-changed', this._changed.bind(this));
|
|
this._proxy.init_async(GLib.PRIORITY_DEFAULT, null)
|
|
.catch(e => console.error(e.message));
|
|
}
|
|
|
|
get airplaneMode() {
|
|
return this._proxy.AirplaneMode;
|
|
}
|
|
|
|
set airplaneMode(v) {
|
|
this._proxy.AirplaneMode = v;
|
|
}
|
|
|
|
get hwAirplaneMode() {
|
|
return this._proxy.HardwareAirplaneMode;
|
|
}
|
|
|
|
get shouldShowAirplaneMode() {
|
|
return this._proxy.ShouldShowAirplaneMode;
|
|
}
|
|
|
|
_changed() {
|
|
this.emit('airplane-mode-changed');
|
|
}
|
|
};
|
|
|
|
var _manager;
|
|
function getRfkillManager() {
|
|
if (_manager != null)
|
|
return _manager;
|
|
|
|
_manager = new RfkillManager();
|
|
return _manager;
|
|
}
|
|
|
|
var Indicator = GObject.registerClass(
|
|
class Indicator extends PanelMenu.SystemIndicator {
|
|
_init() {
|
|
super._init();
|
|
|
|
this._manager = getRfkillManager();
|
|
this._manager.connect('airplane-mode-changed', this._sync.bind(this));
|
|
|
|
this._indicator = this._addIndicator();
|
|
this._indicator.icon_name = 'airplane-mode-symbolic';
|
|
this._indicator.hide();
|
|
|
|
// The menu only appears when airplane mode is on, so just
|
|
// statically build it as if it was on, rather than dynamically
|
|
// changing the menu contents.
|
|
this._item = new PopupMenu.PopupSubMenuMenuItem(_("Airplane Mode On"), true);
|
|
this._item.icon.icon_name = 'airplane-mode-symbolic';
|
|
this._offItem = this._item.menu.addAction(_("Turn Off"), () => {
|
|
this._manager.airplaneMode = false;
|
|
});
|
|
this._item.menu.addSettingsAction(_("Network Settings"), 'gnome-network-panel.desktop');
|
|
this.menu.addMenuItem(this._item);
|
|
|
|
Main.sessionMode.connect('updated', this._sessionUpdated.bind(this));
|
|
this._sessionUpdated();
|
|
|
|
this._sync();
|
|
}
|
|
|
|
_sessionUpdated() {
|
|
let sensitive = !Main.sessionMode.isLocked && !Main.sessionMode.isGreeter;
|
|
this.menu.setSensitive(sensitive);
|
|
}
|
|
|
|
_sync() {
|
|
let airplaneMode = this._manager.airplaneMode;
|
|
let hwAirplaneMode = this._manager.hwAirplaneMode;
|
|
let showAirplaneMode = this._manager.shouldShowAirplaneMode;
|
|
|
|
this._indicator.visible = airplaneMode && showAirplaneMode;
|
|
this._item.visible = airplaneMode && showAirplaneMode;
|
|
this._offItem.setSensitive(!hwAirplaneMode);
|
|
|
|
if (hwAirplaneMode)
|
|
this._offItem.label.text = _("Use hardware switch to turn off");
|
|
else
|
|
this._offItem.label.text = _("Turn Off");
|
|
}
|
|
});
|