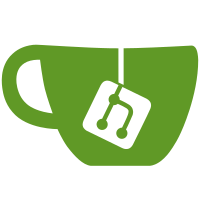
Any time estimates we can come up with are notoriously unreliable; even on devices that report correct (dis)charging rates, any change in workload, screen brightness etc. can throw our estimate off by a huge amount. This is further compounded by bad firmware and battery firmware which reports inaccurate data as neither Windows nor Android do not use that data. So instead, limit ourselves to only showing the current percentage and leave its interpretation to the user. As an added bonus, we end up with shorter strings that are less likely to cause problems with ellipsization when translated. https://bugzilla.gnome.org/show_bug.cgi?id=708472
90 lines
3.3 KiB
JavaScript
90 lines
3.3 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
const Gio = imports.gi.Gio;
|
|
const Lang = imports.lang;
|
|
const UPower = imports.gi.UPowerGlib;
|
|
|
|
const Main = imports.ui.main;
|
|
const PanelMenu = imports.ui.panelMenu;
|
|
const PopupMenu = imports.ui.popupMenu;
|
|
|
|
const BUS_NAME = 'org.freedesktop.UPower';
|
|
const OBJECT_PATH = '/org/freedesktop/UPower/devices/DisplayDevice';
|
|
|
|
const DisplayDeviceInterface = '<node> \
|
|
<interface name="org.freedesktop.UPower.Device"> \
|
|
<property name="Type" type="u" access="read"/> \
|
|
<property name="State" type="u" access="read"/> \
|
|
<property name="Percentage" type="d" access="read"/> \
|
|
<property name="TimeToEmpty" type="x" access="read"/> \
|
|
<property name="TimeToFull" type="x" access="read"/> \
|
|
<property name="IsPresent" type="b" access="read"/> \
|
|
<property name="IconName" type="s" access="read"/> \
|
|
</interface> \
|
|
</node>';
|
|
|
|
const PowerManagerProxy = Gio.DBusProxy.makeProxyWrapper(DisplayDeviceInterface);
|
|
|
|
const Indicator = new Lang.Class({
|
|
Name: 'PowerIndicator',
|
|
Extends: PanelMenu.SystemIndicator,
|
|
|
|
_init: function() {
|
|
this.parent();
|
|
|
|
this._indicator = this._addIndicator();
|
|
|
|
this._proxy = new PowerManagerProxy(Gio.DBus.system, BUS_NAME, OBJECT_PATH,
|
|
Lang.bind(this, function(proxy, error) {
|
|
if (error) {
|
|
log(error.message);
|
|
return;
|
|
}
|
|
this._proxy.connect('g-properties-changed',
|
|
Lang.bind(this, this._sync));
|
|
this._sync();
|
|
}));
|
|
|
|
this._item = new PopupMenu.PopupSubMenuMenuItem("", true);
|
|
this._item.menu.addSettingsAction(_("Power Settings"), 'gnome-power-panel.desktop');
|
|
this.menu.addMenuItem(this._item);
|
|
|
|
Main.sessionMode.connect('updated', Lang.bind(this, this._sessionUpdated));
|
|
this._sessionUpdated();
|
|
},
|
|
|
|
_sessionUpdated: function() {
|
|
let sensitive = !Main.sessionMode.isLocked && !Main.sessionMode.isGreeter;
|
|
this.menu.setSensitive(sensitive);
|
|
},
|
|
|
|
_getStatus: function() {
|
|
if (this._proxy.State == UPower.DeviceState.FULLY_CHARGED)
|
|
return _("Fully Charged");
|
|
else if (this._proxy.State == UPower.DeviceState.CHARGING)
|
|
return _("%d\u2009%% Charging").format(hours, minutes, this._proxy.Percentage);
|
|
return _("%d\u2009%% Charged").format(this._proxy.Percentage);
|
|
},
|
|
|
|
_sync: function() {
|
|
// Do we have batteries or a UPS?
|
|
let visible = this._proxy.IsPresent;
|
|
if (visible) {
|
|
this._item.actor.show();
|
|
} else {
|
|
// If there's no battery, then we use the power icon.
|
|
this._item.actor.hide();
|
|
this._indicator.icon_name = 'system-shutdown-symbolic';
|
|
return;
|
|
}
|
|
|
|
// The icons
|
|
let icon = this._proxy.IconName;
|
|
this._indicator.icon_name = icon;
|
|
this._item.icon.icon_name = icon;
|
|
|
|
// The status label
|
|
this._item.label.text = this._getStatus();
|
|
},
|
|
});
|