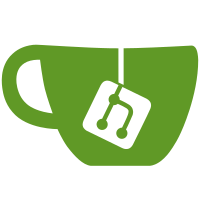
Previously, we had ShellAppInfo, which contains fundamental information about an application, and methods on ShellAppMonitor to retrieve "live" information like the window list. AppIcon ended up being used as the "App" class which was painful for various reasons; among them that we need to handle window list changes, and some consumers weren't ready for that. Clean things up a bit by introducing a new ShellApp class in C, which currently wraps a ShellAppInfo. AppIcon then is more like the display actor for a ShellApp. Notably, the ".windows" property moves out of it. The altTab code which won't handle dynamic changes instead is changed to maintain a cached version. ShellAppMonitor gains some more methods related to ShellApp now. In the future, we might consider changing ShellApp to be a GInterface, which could be implemented by ShellDesktopFileApp, ShellWindowApp. Then we could axe ShellAppInfo from the "public" API and it would return to being an internal loss mitigation layer for GMenu. https://bugzilla.gnome.org/show_bug.cgi?id=598227
57 lines
1.7 KiB
C
57 lines
1.7 KiB
C
/* -*- mode: C; c-file-style: "gnu"; indent-tabs-mode: nil; -*- */
|
|
#ifndef __SHELL_APP_H__
|
|
#define __SHELL_APP_H__
|
|
|
|
#include <glib-object.h>
|
|
#include <glib.h>
|
|
|
|
#include "window.h"
|
|
#include "shell-app-system.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
typedef struct _ShellApp ShellApp;
|
|
typedef struct _ShellAppClass ShellAppClass;
|
|
typedef struct _ShellAppPrivate ShellAppPrivate;
|
|
|
|
#define SHELL_TYPE_APP (shell_app_get_type ())
|
|
#define SHELL_APP(object) (G_TYPE_CHECK_INSTANCE_CAST ((object), SHELL_TYPE_APP, ShellApp))
|
|
#define SHELL_APP_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), SHELL_TYPE_APP, ShellAppClass))
|
|
#define SHELL_IS_APP(object) (G_TYPE_CHECK_INSTANCE_TYPE ((object), SHELL_TYPE_APP))
|
|
#define SHELL_IS_APP_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), SHELL_TYPE_APP))
|
|
#define SHELL_APP_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), SHELL_TYPE_APP, ShellAppClass))
|
|
|
|
struct _ShellAppClass
|
|
{
|
|
GObjectClass parent_class;
|
|
|
|
};
|
|
|
|
GType shell_app_get_type (void) G_GNUC_CONST;
|
|
|
|
const char *shell_app_get_id (ShellApp *app);
|
|
|
|
ClutterActor *shell_app_create_icon_texture (ShellApp *app, float size);
|
|
char *shell_app_get_name (ShellApp *app);
|
|
char *shell_app_get_description (ShellApp *app);
|
|
|
|
ShellAppInfo *shell_app_get_info (ShellApp *app);
|
|
|
|
GSList *shell_app_get_windows (ShellApp *app);
|
|
|
|
gboolean shell_app_is_on_workspace (ShellApp *app, MetaWorkspace *workspace);
|
|
|
|
int shell_app_compare (ShellApp *app, ShellApp *other);
|
|
|
|
ShellApp* _shell_app_new_for_window (MetaWindow *window);
|
|
|
|
ShellApp* _shell_app_new (ShellAppInfo *appinfo);
|
|
|
|
void _shell_app_add_window (ShellApp *app, MetaWindow *window);
|
|
|
|
void _shell_app_remove_window (ShellApp *app, MetaWindow *window);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __SHELL_APP_H__ */
|