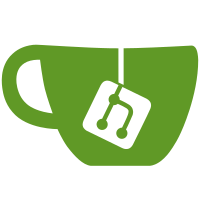
Start using the new methods to simplify signal cleanup. For now, focus on replacing existing cleanups; in most cases this means signals connected in the constructor and disconnected on destroy, but also other cases with a similarly defined lifetime (say: from show to hide). This doesn't change signal connections that only exist for a short time (say: once), handlers that are connected on-demand (say: the first time a particular method is called), or connections that aren't tracked (read: disconnected) at all. We will eventually replace the latter with connectObject() as well - especially from actor subclasses - but the changeset is already big enough as-is :-) Part-of: <https://gitlab.gnome.org/GNOME/gnome-shell/-/merge_requests/1953>
109 lines
3.1 KiB
JavaScript
109 lines
3.1 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
const Gio = imports.gi.Gio;
|
|
const Signals = imports.signals;
|
|
|
|
const { loadInterfaceXML } = imports.misc.fileUtils;
|
|
|
|
const ProviderIface = loadInterfaceXML("org.freedesktop.realmd.Provider");
|
|
const Provider = Gio.DBusProxy.makeProxyWrapper(ProviderIface);
|
|
|
|
const ServiceIface = loadInterfaceXML("org.freedesktop.realmd.Service");
|
|
const Service = Gio.DBusProxy.makeProxyWrapper(ServiceIface);
|
|
|
|
const RealmIface = loadInterfaceXML("org.freedesktop.realmd.Realm");
|
|
const Realm = Gio.DBusProxy.makeProxyWrapper(RealmIface);
|
|
|
|
var Manager = class {
|
|
constructor() {
|
|
this._aggregateProvider = Provider(Gio.DBus.system,
|
|
'org.freedesktop.realmd',
|
|
'/org/freedesktop/realmd',
|
|
this._reloadRealms.bind(this));
|
|
this._realms = {};
|
|
this._loginFormat = null;
|
|
|
|
this._aggregateProvider.connectObject('g-properties-changed',
|
|
(proxy, properties) => {
|
|
if ('Realms' in properties.deep_unpack())
|
|
this._reloadRealms();
|
|
}, this);
|
|
}
|
|
|
|
_reloadRealms() {
|
|
let realmPaths = this._aggregateProvider.Realms;
|
|
|
|
if (!realmPaths)
|
|
return;
|
|
|
|
for (let i = 0; i < realmPaths.length; i++) {
|
|
Realm(Gio.DBus.system,
|
|
'org.freedesktop.realmd',
|
|
realmPaths[i],
|
|
this._onRealmLoaded.bind(this));
|
|
}
|
|
}
|
|
|
|
_reloadRealm(realm) {
|
|
if (!realm.Configured) {
|
|
if (this._realms[realm.get_object_path()])
|
|
delete this._realms[realm.get_object_path()];
|
|
|
|
return;
|
|
}
|
|
|
|
this._realms[realm.get_object_path()] = realm;
|
|
|
|
this._updateLoginFormat();
|
|
}
|
|
|
|
_onRealmLoaded(realm, error) {
|
|
if (error)
|
|
return;
|
|
|
|
this._reloadRealm(realm);
|
|
|
|
realm.connect('g-properties-changed', (proxy, properties) => {
|
|
if ('Configured' in properties.deep_unpack())
|
|
this._reloadRealm(realm);
|
|
});
|
|
}
|
|
|
|
_updateLoginFormat() {
|
|
let newLoginFormat;
|
|
|
|
for (let realmPath in this._realms) {
|
|
let realm = this._realms[realmPath];
|
|
if (realm.LoginFormats && realm.LoginFormats.length > 0) {
|
|
newLoginFormat = realm.LoginFormats[0];
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (this._loginFormat != newLoginFormat) {
|
|
this._loginFormat = newLoginFormat;
|
|
this.emit('login-format-changed', newLoginFormat);
|
|
}
|
|
}
|
|
|
|
get loginFormat() {
|
|
if (this._loginFormat)
|
|
return this._loginFormat;
|
|
|
|
this._updateLoginFormat();
|
|
|
|
return this._loginFormat;
|
|
}
|
|
|
|
release() {
|
|
Service(Gio.DBus.system,
|
|
'org.freedesktop.realmd',
|
|
'/org/freedesktop/realmd',
|
|
service => service.ReleaseRemote());
|
|
this._aggregateProvider.disconnectObject(this);
|
|
this._realms = { };
|
|
this._updateLoginFormat();
|
|
}
|
|
};
|
|
Signals.addSignalMethods(Manager.prototype);
|