ibusManager: Preload all ibus input sources in user configuration
Normally users switch xkb input sources and ibus input sources. But currently the first input source only is running. It's also good to preload all ibus engines in the logging session so that users switch input sources quickly without the launching time of input sources. The following is the ibus change: https://github.com/ibus/ibus/commit/cff35929a9 https://bugzilla.gnome.org/show_bug.cgi?id=695428
This commit is contained in:
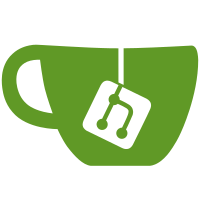
committed by
Rui Matos
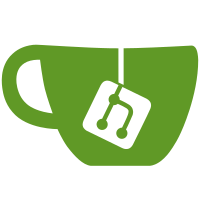
parent
050378743e
commit
e467a734a1
@@ -1,13 +1,14 @@
|
|||||||
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
||||||
|
|
||||||
const Gio = imports.gi.Gio;
|
const Gio = imports.gi.Gio;
|
||||||
|
const GLib = imports.gi.GLib;
|
||||||
const Lang = imports.lang;
|
const Lang = imports.lang;
|
||||||
|
const Mainloop = imports.mainloop;
|
||||||
const Signals = imports.signals;
|
const Signals = imports.signals;
|
||||||
|
|
||||||
try {
|
try {
|
||||||
var IBus = imports.gi.IBus;
|
var IBus = imports.gi.IBus;
|
||||||
if (!('new_async' in IBus.Bus))
|
_checkIBusVersion();
|
||||||
throw "IBus version is too old";
|
|
||||||
const IBusCandidatePopup = imports.ui.ibusCandidatePopup;
|
const IBusCandidatePopup = imports.ui.ibusCandidatePopup;
|
||||||
} catch (e) {
|
} catch (e) {
|
||||||
var IBus = null;
|
var IBus = null;
|
||||||
@@ -16,6 +17,22 @@ try {
|
|||||||
|
|
||||||
let _ibusManager = null;
|
let _ibusManager = null;
|
||||||
|
|
||||||
|
function _checkIBusVersion() {
|
||||||
|
var requiredMajor = 1;
|
||||||
|
var requiredMinor = 5;
|
||||||
|
var requiredMicro = 2;
|
||||||
|
|
||||||
|
if ((IBus.MAJOR_VERSION > requiredMajor) ||
|
||||||
|
(IBus.MAJOR_VERSION == requiredMajor && IBus.MINOR_VERSION > requiredMinor) ||
|
||||||
|
(IBus.MAJOR_VERSION == requiredMajor && IBus.MINOR_VERSION == requiredMinor &&
|
||||||
|
IBus.MICRO_VERSION >= requiredMicro))
|
||||||
|
return;
|
||||||
|
|
||||||
|
throw "Found IBus version %d.%d.%d but required is %d.%d.%d".
|
||||||
|
format(IBus.MAJOR_VERSION, IBus.MINOR_VERSION, IBus.MINOR_VERSION,
|
||||||
|
requiredMajor, requiredMinor, requiredMicro);
|
||||||
|
}
|
||||||
|
|
||||||
function getIBusManager() {
|
function getIBusManager() {
|
||||||
if (_ibusManager == null)
|
if (_ibusManager == null)
|
||||||
_ibusManager = new IBusManager();
|
_ibusManager = new IBusManager();
|
||||||
@@ -28,6 +45,7 @@ const IBusManager = new Lang.Class({
|
|||||||
// This is the longest we'll keep the keyboard frozen until an input
|
// This is the longest we'll keep the keyboard frozen until an input
|
||||||
// source is active.
|
// source is active.
|
||||||
_MAX_INPUT_SOURCE_ACTIVATION_TIME: 4000, // ms
|
_MAX_INPUT_SOURCE_ACTIVATION_TIME: 4000, // ms
|
||||||
|
_PRELOAD_ENGINES_DELAY_TIME: 30, // sec
|
||||||
|
|
||||||
_init: function() {
|
_init: function() {
|
||||||
if (!IBus)
|
if (!IBus)
|
||||||
@@ -42,6 +60,7 @@ const IBusManager = new Lang.Class({
|
|||||||
this._ready = false;
|
this._ready = false;
|
||||||
this._registerPropertiesId = 0;
|
this._registerPropertiesId = 0;
|
||||||
this._currentEngineName = null;
|
this._currentEngineName = null;
|
||||||
|
this._preloadEnginesId = 0;
|
||||||
|
|
||||||
this._ibus = IBus.Bus.new_async();
|
this._ibus = IBus.Bus.new_async();
|
||||||
this._ibus.connect('connected', Lang.bind(this, this._onConnected));
|
this._ibus.connect('connected', Lang.bind(this, this._onConnected));
|
||||||
@@ -176,5 +195,27 @@ const IBusManager = new Lang.Class({
|
|||||||
this._ibus.set_global_engine_async(id, this._MAX_INPUT_SOURCE_ACTIVATION_TIME,
|
this._ibus.set_global_engine_async(id, this._MAX_INPUT_SOURCE_ACTIVATION_TIME,
|
||||||
null, callback);
|
null, callback);
|
||||||
},
|
},
|
||||||
|
|
||||||
|
preloadEngines: function(ids) {
|
||||||
|
if (!IBus || !this._ibus || ids.length == 0)
|
||||||
|
return;
|
||||||
|
|
||||||
|
if (this._preloadEnginesId != 0) {
|
||||||
|
Mainloop.source_remove(this._preloadEnginesId);
|
||||||
|
this._preloadEnginesId = 0;
|
||||||
|
}
|
||||||
|
|
||||||
|
this._preloadEnginesId =
|
||||||
|
Mainloop.timeout_add_seconds(this._PRELOAD_ENGINES_DELAY_TIME,
|
||||||
|
Lang.bind(this, function() {
|
||||||
|
this._ibus.preload_engines_async(
|
||||||
|
ids,
|
||||||
|
-1,
|
||||||
|
null,
|
||||||
|
null);
|
||||||
|
this._preloadEnginesId = 0;
|
||||||
|
return GLib.SOURCE_REMOVE;
|
||||||
|
}));
|
||||||
|
},
|
||||||
});
|
});
|
||||||
Signals.addSignalMethods(IBusManager.prototype);
|
Signals.addSignalMethods(IBusManager.prototype);
|
||||||
|
@@ -381,6 +381,10 @@ const InputSourceManager = new Lang.Class({
|
|||||||
|
|
||||||
if (this._mruSources.length > 0)
|
if (this._mruSources.length > 0)
|
||||||
this._mruSources[0].activate();
|
this._mruSources[0].activate();
|
||||||
|
|
||||||
|
// All ibus engines are preloaded here to reduce the launching time
|
||||||
|
// when users switch the input sources.
|
||||||
|
this._ibusManager.preloadEngines(Object.keys(this._ibusSources));
|
||||||
},
|
},
|
||||||
|
|
||||||
_makeEngineShortName: function(engineDesc) {
|
_makeEngineShortName: function(engineDesc) {
|
||||||
|
Reference in New Issue
Block a user