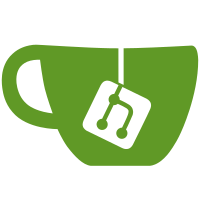
Rework part of the show/hide machinery. Allow groups sub-classes and composite actors to override show_all/hide_all in order to decide which children they wish to show/hide. This means that if an actor overrides the default show/hide virtual methods, it'll have to chain up to the parent class show/hide. While we're at it, provide the fully recursive clutter_actor_show_all() and clutter_actor_hide_all() methods. * clutter/clutter-behaviour-path.c: Add apidoc for the ClutterKnot functions; add pathological equality case for clutter_knot_equal(). * clutter/clutter-event.h: * clutter/clutter-feature.h: * clutter/clutter-behaviour.c: * clutter/clutter-behaviour-scale.c:Fix parameters name so that gtk-doc doesn't complain. * clutter/clutter-actor.c: * clutter/clutter-event.c: Add apidoc * clutter/clutter-actor.h: * clutter/clutter-actor.c: Add a clutter_actor_show_all() and a clutter_actor_hide_all() functions; provide a mechanism for groups and composited actors to programmatically select what to show/hide when clutter_actor_show_all() and clutter_actor_hide_all() are called. If you are overriding the ClutterActor::show or the ClutterActor::hide virtual methods you should chain up with the parent class. * clutter/clutter-group.c: Override show_all and hide_all and recursively show/hide every child inside the group; clutter_group_show_all() and clutter_group_hide_all() remain as non recursive versions of clutter_actor_show_all() and clutter_actor_hide_all() (maybe we should rename them in order to avoid name clashes with the bindings). * clutter/clutter-stage.c: * clutter/clutter-texture.c: Chain up with parent class show and hide vfuncs. * clutter/clutter-clone-texture.h: * clutter/clutter-clone-texture.c: Provide API for changing the parent texture of a clone texture actor. * examples/behave.c: * examples/super-oh.c: * examples/test.c: Use clutter_actor_show_all() instead of clutter_group_show_all().
111 lines
4.5 KiB
C
111 lines
4.5 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2006 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef _HAVE_CLUTTER_TEXTURE_H
|
|
#define _HAVE_CLUTTER_TEXTURE_H
|
|
|
|
#include <clutter/clutter-actor.h>
|
|
#include <gdk-pixbuf/gdk-pixbuf.h>
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_TEXTURE (clutter_texture_get_type ())
|
|
#define CLUTTER_TEXTURE(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), CLUTTER_TYPE_TEXTURE, ClutterTexture))
|
|
#define CLUTTER_TEXTURE_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), CLUTTER_TYPE_TEXTURE, ClutterTextureClass))
|
|
#define CLUTTER_IS_TEXTURE(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), CLUTTER_TYPE_TEXTURE))
|
|
#define CLUTTER_IS_TEXTURE_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), CLUTTER_TYPE_TEXTURE))
|
|
#define CLUTTER_TEXTURE_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), CLUTTER_TYPE_TEXTURE, ClutterTextureClass))
|
|
|
|
typedef struct _ClutterTexture ClutterTexture;
|
|
typedef struct _ClutterTextureClass ClutterTextureClass;
|
|
typedef struct _ClutterTexturePrivate ClutterTexturePrivate;
|
|
|
|
struct _ClutterTexture
|
|
{
|
|
ClutterActor parent;
|
|
|
|
ClutterTexturePrivate *priv;
|
|
};
|
|
|
|
struct _ClutterTextureClass
|
|
{
|
|
ClutterActorClass parent_class;
|
|
|
|
void (*size_change) (ClutterTexture *texture,
|
|
gint width,
|
|
gint height);
|
|
void (*pixbuf_change) (ClutterTexture *texture);
|
|
|
|
/* padding, for future expansion */
|
|
void (*_clutter_texture1) (void);
|
|
void (*_clutter_texture2) (void);
|
|
void (*_clutter_texture3) (void);
|
|
void (*_clutter_texture4) (void);
|
|
void (*_clutter_texture5) (void);
|
|
void (*_clutter_texture6) (void);
|
|
};
|
|
|
|
GType clutter_texture_get_type (void) G_GNUC_CONST;
|
|
|
|
ClutterActor *clutter_texture_new (void);
|
|
ClutterActor *clutter_texture_new_from_pixbuf (GdkPixbuf *pixbuf);
|
|
void clutter_texture_set_from_data (ClutterTexture *texture,
|
|
const guchar *data,
|
|
gboolean has_alpha,
|
|
gint width,
|
|
gint height,
|
|
gint rowstride,
|
|
gint bpp);
|
|
void clutter_texture_set_pixbuf (ClutterTexture *texture,
|
|
GdkPixbuf *pixbuf);
|
|
GdkPixbuf * clutter_texture_get_pixbuf (ClutterTexture *texture);
|
|
void clutter_texture_get_base_size (ClutterTexture *texture,
|
|
gint *width,
|
|
gint *height);
|
|
|
|
/* Below mainly for subclassed texture based actors */
|
|
|
|
void clutter_texture_bind_tile (ClutterTexture *texture,
|
|
gint index);
|
|
void clutter_texture_get_n_tiles (ClutterTexture *texture,
|
|
gint *n_x_tiles,
|
|
gint *n_y_tiles);
|
|
void clutter_texture_get_x_tile_detail (ClutterTexture *texture,
|
|
gint x_index,
|
|
gint *pos,
|
|
gint *size,
|
|
gint *waste);
|
|
void clutter_texture_get_y_tile_detail (ClutterTexture *texture,
|
|
gint y_index,
|
|
gint *pos,
|
|
gint *size,
|
|
gint *waste);
|
|
gboolean clutter_texture_has_generated_tiles (ClutterTexture *texture);
|
|
gboolean clutter_texture_is_tiled (ClutterTexture *texture);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* _HAVE_CLUTTER_TEXTURE_H */
|