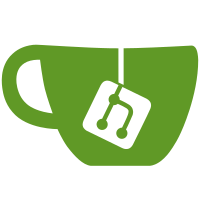
The Animatable interface was created specifically for the Animation class. It turns out that it might be fairly useful to others - such as ClutterAnimator and ClutterState. The newly-added API in this cycle for querying and accessing custom properties should not require that we pass a ClutterAnimation to the implementations: the Animatable itself should be enough. This is necessary to allow language bindings to wrap clutter_actor_animate() correctly and do type validation and demarshalling between native values and GValues; an Animation instance is not available until the animate() call returns, and validation must be performed before that happens. There is nothing we can do about the animate_property() virtual function - but in that case we might want to be able to access the animation from an Animatable implementation to get the Interval for the property, just like ClutterActor does in order to animate ClutterActorMeta objects.
114 lines
4.7 KiB
C
114 lines
4.7 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Copyright (C) 2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* Author:
|
|
* Emmanuele Bassi <ebassi@linux.intel.com>
|
|
*/
|
|
|
|
#ifndef __CLUTTER_ANIMATABLE_H__
|
|
#define __CLUTTER_ANIMATABLE_H__
|
|
|
|
#if !defined(__CLUTTER_H_INSIDE__) && !defined(CLUTTER_COMPILATION)
|
|
#error "Only <clutter/clutter.h> can be included directly."
|
|
#endif
|
|
|
|
#include <clutter/clutter-animation.h>
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_ANIMATABLE (clutter_animatable_get_type ())
|
|
#define CLUTTER_ANIMATABLE(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), CLUTTER_TYPE_ANIMATABLE, ClutterAnimatable))
|
|
#define CLUTTER_IS_ANIMATABLE(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), CLUTTER_TYPE_ANIMATABLE))
|
|
#define CLUTTER_ANIMATABLE_GET_IFACE(obj) (G_TYPE_INSTANCE_GET_INTERFACE ((obj), CLUTTER_TYPE_ANIMATABLE, ClutterAnimatableIface))
|
|
|
|
typedef struct _ClutterAnimatable ClutterAnimatable; /* dummy typedef */
|
|
typedef struct _ClutterAnimatableIface ClutterAnimatableIface;
|
|
|
|
/**
|
|
* ClutterAnimatable:
|
|
*
|
|
* #ClutterAnimatable is an opaque structure whose members cannot be directly
|
|
* accessed
|
|
*
|
|
* Since: 1.0
|
|
*/
|
|
|
|
/**
|
|
* ClutterAnimatableIface:
|
|
* @animate_property: virtual function for custom interpolation of a
|
|
* property
|
|
* @find_property: virtual function for retrieving the #GParamSpec of
|
|
* an animatable property
|
|
* @get_initial_state: virtual function for retrieving the initial
|
|
* state of an animatable property
|
|
* @set_final_state: virtual function for setting the state of an
|
|
* animatable property
|
|
*
|
|
* Base interface for #GObject<!-- -->s that can be animated by a
|
|
* a #ClutterAnimation.
|
|
*
|
|
* Since: 1.0
|
|
*/
|
|
struct _ClutterAnimatableIface
|
|
{
|
|
/*< private >*/
|
|
GTypeInterface parent_iface;
|
|
|
|
/*< public >*/
|
|
gboolean (* animate_property) (ClutterAnimatable *animatable,
|
|
ClutterAnimation *animation,
|
|
const gchar *property_name,
|
|
const GValue *initial_value,
|
|
const GValue *final_value,
|
|
gdouble progress,
|
|
GValue *value);
|
|
GParamSpec *(* find_property) (ClutterAnimatable *animatable,
|
|
const gchar *property_name);
|
|
void (* get_initial_state) (ClutterAnimatable *animatable,
|
|
const gchar *property_name,
|
|
GValue *value);
|
|
void (* set_final_state) (ClutterAnimatable *animatable,
|
|
const gchar *property_name,
|
|
const GValue *value);
|
|
};
|
|
|
|
GType clutter_animatable_get_type (void) G_GNUC_CONST;
|
|
|
|
gboolean clutter_animatable_animate_property (ClutterAnimatable *animatable,
|
|
ClutterAnimation *animation,
|
|
const gchar *property_name,
|
|
const GValue *initial_value,
|
|
const GValue *final_value,
|
|
gdouble progress,
|
|
GValue *value);
|
|
|
|
GParamSpec *clutter_animatable_find_property (ClutterAnimatable *animatable,
|
|
const gchar *property_name);
|
|
void clutter_animatable_get_initial_state (ClutterAnimatable *animatable,
|
|
const gchar *property_name,
|
|
GValue *value);
|
|
void clutter_animatable_set_final_state (ClutterAnimatable *animatable,
|
|
const gchar *property_name,
|
|
const GValue *value);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __CLUTTER_ANIMATABLE_H__ */
|