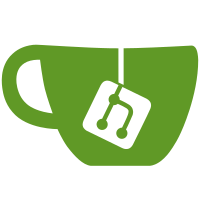
Previously when a pipeline is added to the cache it would never be removed. If the application is generating a lot of unique pipelines this can end up effectively leaking a large number of resources including the GL program objects. Arguably this isn't really a problem because if the application is generating that many unique pipelines then it is doing something wrong anyway. It also implies that it will be recompiling shaders very often so the cache leaking will likely be the least of the problems. This patch makes it keep track of which pipelines in the cache are in use. The cache now returns a struct representing the entry instead of directly returning the pipeline. This entry contains a usage counter which the pipeline backends can use to mark when there is a pipeline alive that is using the cache entry. When the hash table decides that it's a good time to prune some entries, it will make a list of all of the pipelines that are not in use and then remove the least recently used half of the pipelines. That way it is less likely to remove pipelines that the application is actually regenerating often even if they aren't in use all of the time. When the cache is pruned the hash table makes a note of how small the cache could be if it removed all of the unused pipelines. The hash table starts pruning when there are more entries than twice this minimum expected size. The idea is that if that case it hit then the hash table is more than half full of useless pipelines so the application is generating lots of redundant pipelines and it is a good time to remove them. Reviewed-by: Robert Bragg <robert@linux.intel.com> (cherry picked from commit c21aac22992bb7fef5a8d0913130b8245e67f2eb) Conflicts: cogl/driver/gl/cogl-pipeline-fragend-glsl.c cogl/driver/gl/cogl-pipeline-progend-glsl.c cogl/driver/gl/cogl-pipeline-vertend-glsl.c cogl/driver/gl/gl/cogl-pipeline-fragend-arbfp.c
87 lines
3.0 KiB
C
87 lines
3.0 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2011 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_PIPELINE_CACHE_H__
|
|
#define __COGL_PIPELINE_CACHE_H__
|
|
|
|
#include "cogl-pipeline.h"
|
|
|
|
typedef struct _CoglPipelineCache CoglPipelineCache;
|
|
|
|
typedef struct
|
|
{
|
|
CoglPipeline *pipeline;
|
|
|
|
/* Number of usages of this template. If this drops to zero then it
|
|
* will be a candidate for removal from the cache */
|
|
int usage_count;
|
|
} CoglPipelineCacheEntry;
|
|
|
|
CoglPipelineCache *
|
|
_cogl_pipeline_cache_new (void);
|
|
|
|
void
|
|
_cogl_pipeline_cache_free (CoglPipelineCache *cache);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_FRAGMENT_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipelineCacheEntry *
|
|
_cogl_pipeline_cache_get_fragment_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_VERTEX_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipelineCacheEntry *
|
|
_cogl_pipeline_cache_get_vertex_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
/*
|
|
* Gets a pipeline from the cache that has the same state as
|
|
* @key_pipeline for the combination of the state state in
|
|
* COGL_PIPELINE_STATE_AFFECTS_VERTEX_CODEGEN and
|
|
* COGL_PIPELINE_STATE_AFFECTS_FRAGMENT_CODEGEN. If there is no
|
|
* matching pipline already then a copy of key_pipeline is stored in
|
|
* the cache so that it will be used next time the function is called
|
|
* with a similar pipeline. In that case the copy itself will be
|
|
* returned
|
|
*/
|
|
CoglPipelineCacheEntry *
|
|
_cogl_pipeline_cache_get_combined_template (CoglPipelineCache *cache,
|
|
CoglPipeline *key_pipeline);
|
|
|
|
#endif /* __COGL_PIPELINE_CACHE_H__ */
|