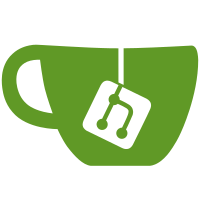
The CSS3 Transitions specification from the W3C defines the possibility of using a parametrized step() timing function, with the following prototype: steps(n_steps, [ start | end ]) where @n_steps represents the number of steps used to divide an interval between 0 and 1; the 'start' and 'end' tokens describe whether the value change should happen at the start of the transition, or at the end. For instance, the "steps(3, start)" timing function has the following profile: 1 | x | | | x---| | ' | | x---' | | ' | 0 |---' | Whereas the "steps(3, end)" timing function has the following profile: 1 | x---| | ' | | x---' | | ' | x---' | | | 0 | | Since ClutterTimeline uses an enumeration for controlling the progress mode, we need additional API to define the parameters of the steps() progress; for this reason, we need a CLUTTER_STEPS enumeration value, and a method for setting the number of steps and the value transition policy. The CSS3 Transitions spec helpfully also defines a step-start and a step-end shorthands, which expand to step(1, start) and step(1, end) respectively; we can provide a CLUTTER_STEP_START and CLUTTER_STEP_END enumeration values for those.
111 lines
3.8 KiB
C
111 lines
3.8 KiB
C
#include <glib.h>
|
|
#include <clutter/clutter.h>
|
|
#include "test-conform-common.h"
|
|
|
|
void
|
|
timeline_progress_step (TestConformSimpleFixture *fixture G_GNUC_UNUSED,
|
|
gconstpointer dummy G_GNUC_UNUSED)
|
|
{
|
|
ClutterTimeline *timeline;
|
|
|
|
timeline = clutter_timeline_new (1000);
|
|
|
|
if (g_test_verbose ())
|
|
g_print ("mode: step(3, end)\n");
|
|
|
|
clutter_timeline_rewind (timeline);
|
|
clutter_timeline_set_step_progress (timeline, 3, CLUTTER_STEP_MODE_END);
|
|
g_assert_cmpint (clutter_timeline_get_progress (timeline), ==, 0);
|
|
|
|
clutter_timeline_advance (timeline, 1000 / 3 - 1);
|
|
g_assert_cmpint (clutter_timeline_get_progress (timeline) * 1000, ==, 0);
|
|
|
|
clutter_timeline_advance (timeline, 1000 / 3 + 1);
|
|
g_assert_cmpint (clutter_timeline_get_progress (timeline) * 1000, ==, 333);
|
|
|
|
clutter_timeline_advance (timeline, 1000 / 3 * 2 - 1);
|
|
g_assert_cmpint (clutter_timeline_get_progress (timeline) * 1000, ==, 333);
|
|
|
|
clutter_timeline_advance (timeline, 1000 / 3 * 2 + 1);
|
|
g_assert_cmpint (clutter_timeline_get_progress (timeline) * 1000, ==, 666);
|
|
|
|
clutter_timeline_rewind (timeline);
|
|
clutter_timeline_set_progress_mode (timeline, CLUTTER_STEP_START);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 1);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 500);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 999);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 1000);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
if (g_test_verbose ())
|
|
g_print ("mode: step-start\n");
|
|
|
|
clutter_timeline_rewind (timeline);
|
|
clutter_timeline_set_progress_mode (timeline, CLUTTER_STEP_START);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 1);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 500);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 999);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_advance (timeline, 1000);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
if (g_test_verbose ())
|
|
g_print ("mode: step-end\n");
|
|
|
|
clutter_timeline_rewind (timeline);
|
|
clutter_timeline_set_progress_mode (timeline, CLUTTER_STEP_END);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 1);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 500);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 999);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 1000);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
g_object_unref (timeline);
|
|
}
|
|
|
|
void
|
|
timeline_progress_mode (TestConformSimpleFixture *fixture G_GNUC_UNUSED,
|
|
gconstpointer dummy G_GNUC_UNUSED)
|
|
{
|
|
ClutterTimeline *timeline;
|
|
|
|
timeline = clutter_timeline_new (1000);
|
|
|
|
g_assert (clutter_timeline_get_progress_mode (timeline) == CLUTTER_LINEAR);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
clutter_timeline_advance (timeline, 500);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.5);
|
|
|
|
clutter_timeline_advance (timeline, 1000);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 1.0);
|
|
|
|
clutter_timeline_rewind (timeline);
|
|
g_assert_cmpfloat (clutter_timeline_get_progress (timeline), ==, 0.0);
|
|
|
|
g_object_unref (timeline);
|
|
}
|